diff --git a/.gitignore b/.gitignore
index 2736c60..3527a30 100644
--- a/.gitignore
+++ b/.gitignore
@@ -20,3 +20,4 @@ _test*
**/*.dll
**/core*[0-9]
.private
+
diff --git a/AUTHORS b/AUTHORS
index a7d71ab..6490085 100644
--- a/AUTHORS
+++ b/AUTHORS
@@ -1 +1,2 @@
-Laurent Le Goff
\ No newline at end of file
+Laurent Le Goff
+Stani Michiels, gmail:stani.be
\ No newline at end of file
diff --git a/README.md b/README.md
index c9aca0c..21c2d94 100644
--- a/README.md
+++ b/README.md
@@ -1,15 +1,19 @@
draw2d
======
-Package draw2d is a pure [go](http://golang.org) 2D vector graphics library with support for multiple output devices such as [images](http://golang.org/pkg/image) (draw2d), pdf documents (draw2dpdf) and opengl (draw2dopengl), which can also be used on the google app engine. It can be used as a pure go [Cairo](http://www.cairographics.org/) alternative.
+Package draw2d is a pure [go](http://golang.org) 2D vector graphics library with support for multiple output devices such as [images](http://golang.org/pkg/image) (draw2d), pdf documents (draw2dpdf) and opengl (draw2dgl), which can also be used on the google app engine. It can be used as a pure go [Cairo](http://www.cairographics.org/) alternative. draw2d is released under the BSD license. See the [documentation](http://godoc.org/github.com/llgcode/draw2d) for more details.
-See the [documentation](http://godoc.org/github.com/llgcode/draw2d) for more details.
+[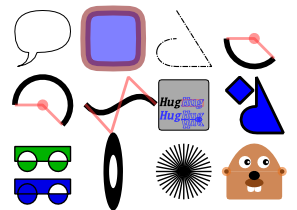](https://raw.githubusercontent.com/llgcode/draw2d/master/resource/image/geometry.pdf)[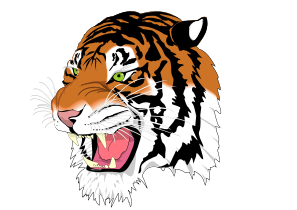](https://raw.githubusercontent.com/llgcode/draw2d/master/resource/image/postscript.pdf)
+
+Click on an image above to get the pdf, generated with exactly the same draw2d code. The first image is the output of `samples/geometry`. The second image is the result of `samples/postcript`, which demonstrates that draw2d can draw postscript files into images or pdf documents with the [ps](https://github.com/llgcode/ps) package.
Features
--------
Operations in draw2d include stroking and filling polygons, arcs, Bézier curves, drawing images and text rendering with truetype fonts. All drawing operations can be transformed by affine transformations (scale, rotation, translation).
+Package draw2d follows the conventions of the [HTML Canvas 2D Context](http://www.w3.org/TR/2dcontext/) for coordinate system, angles, etc...
+
Installation
------------
@@ -42,7 +46,7 @@ gc.Close()
gc.FillStroke()
// Save to file
-draw2d.SaveToPngFile(fn, dest)
+draw2d.SaveToPngFile("hello.png", dest)
```
The same Go code can also generate a pdf document with package draw2dpdf:
@@ -65,13 +69,26 @@ gc.Close()
gc.FillStroke()
// Save to file
-draw2dpdf.SaveToPdfFile(fn, dest)
+draw2dpdf.SaveToPdfFile("hello.pdf", dest)
```
-There are more examples here: https://github.com/llgcode/draw2d.samples
+There are more examples here: https://github.com/llgcode/draw2d/tree/master/samples
Drawing on opengl is provided by the draw2dgl package.
+Testing
+-------
+
+The samples are run as tests from the root package folder `draw2d` by:
+```
+go test ./...
+```
+Or if you want to run with test coverage:
+```
+go test -cover ./... | grep -v "no test"
+```
+This will generate output by the different backends in the output folder.
+
Acknowledgments
---------------
@@ -94,3 +111,4 @@ References
- [antigrain.com](http://www.antigrain.com)
- [freetype-go](http://code.google.com/p/freetype-go)
+ -
diff --git a/draw2d.go b/draw2d.go
index 0d7e3aa..25b0d12 100644
--- a/draw2d.go
+++ b/draw2d.go
@@ -3,8 +3,9 @@
// Package draw2d is a pure go 2D vector graphics library with support
// for multiple output devices such as images (draw2d), pdf documents
-// (draw2dpdf) and opengl (draw2dopengl), which can also be used on the
+// (draw2dpdf) and opengl (draw2dgl), which can also be used on the
// google app engine. It can be used as a pure go Cairo alternative.
+// draw2d is released under the BSD license.
//
// Features
//
@@ -13,6 +14,8 @@
// All drawing operations can be transformed by affine transformations
// (scale, rotation, translation).
//
+// Package draw2d follows the conventions of http://www.w3.org/TR/2dcontext for coordinate system, angles, etc...
+//
// Installation
//
// To install or update the package draw2d on your system, run:
@@ -40,15 +43,25 @@
// gc.FillStroke()
//
// // Save to file
-// draw2d.SaveToPngFile(fn, dest)
+// draw2d.SaveToPngFile("hello.png", dest)
//
// There are more examples here:
-// https://github.com/llgcode/draw2d.samples
+// https://github.com/llgcode/draw2d/tree/master/samples
//
// Drawing on pdf documents is provided by the draw2dpdf package.
// Drawing on opengl is provided by the draw2dgl package.
// See subdirectories at the bottom of this page.
//
+// Testing
+//
+// The samples are run as tests from the root package folder `draw2d` by:
+// go test ./...
+//
+// Or if you want to run with test coverage:
+// go test -cover ./... | grep -v "no test"
+//
+// This will generate output by the different backends in the output folder.
+//
// Acknowledgments
//
// Laurent Le Goff wrote this library, inspired by Postscript and
@@ -58,9 +71,6 @@
// graphic context (https://github.com/llgcode/ps). Stani Michiels
// implemented the pdf backend with the gofpdf package.
//
-// The package depends on freetype-go package for its rasterization
-// algorithm.
-//
// Packages using draw2d
//
// - https://github.com/llgcode/ps: Postscript interpreter written in Go
diff --git a/draw2dbase/curve.go b/draw2dbase/curve.go
index 6447bdc..211e107 100644
--- a/draw2dbase/curve.go
+++ b/draw2dbase/curve.go
@@ -46,9 +46,9 @@ func SubdivideCubic(c, c1, c2 []float64) {
c2[0], c2[1] = c1[6], c1[7]
}
-// TraceCubic generate lines subdividing the cubic curve using a Flattener
+// TraceCubic generate lines subdividing the cubic curve using a Liner
// flattening_threshold helps determines the flattening expectation of the curve
-func TraceCubic(t Flattener, cubic []float64, flatteningThreshold float64) {
+func TraceCubic(t Liner, cubic []float64, flatteningThreshold float64) {
// Allocation curves
var curves [CurveRecursionLimit * 8]float64
copy(curves[0:8], cubic[0:8])
@@ -101,9 +101,9 @@ func SubdivideQuad(c, c1, c2 []float64) {
return
}
-// TraceQuad generate lines subdividing the curve using a Flattener
+// TraceQuad generate lines subdividing the curve using a Liner
// flattening_threshold helps determines the flattening expectation of the curve
-func TraceQuad(t Flattener, quad []float64, flatteningThreshold float64) {
+func TraceQuad(t Liner, quad []float64, flatteningThreshold float64) {
// Allocates curves stack
var curves [CurveRecursionLimit * 6]float64
copy(curves[0:6], quad[0:6])
@@ -131,8 +131,8 @@ func TraceQuad(t Flattener, quad []float64, flatteningThreshold float64) {
}
}
-// TraceArc trace an arc using a Flattener
-func TraceArc(t Flattener, x, y, rx, ry, start, angle, scale float64) (lastX, lastY float64) {
+// TraceArc trace an arc using a Liner
+func TraceArc(t Liner, x, y, rx, ry, start, angle, scale float64) (lastX, lastY float64) {
end := start + angle
clockWise := true
if angle < 0 {
diff --git a/draw2dbase/curve_test.go b/draw2dbase/curve_test.go
index b737862..750521a 100644
--- a/draw2dbase/curve_test.go
+++ b/draw2dbase/curve_test.go
@@ -36,7 +36,7 @@ var (
func init() {
os.Mkdir("test_results", 0666)
- f, err := os.Create("test_results/_test.html")
+ f, err := os.Create("../output/curve/_test.html")
if err != nil {
log.Println(err)
os.Exit(1)
@@ -81,7 +81,7 @@ func TestCubicCurve(t *testing.T) {
raster.PolylineBresenham(img, image.Black, p.Points...)
//drawPoints(img, image.NRGBAColor{0, 0, 0, 0xff}, curve[:]...)
drawPoints(img, color.NRGBA{0, 0, 0, 0xff}, p.Points...)
- SaveToPngFile(fmt.Sprintf("test_results/_test%d.png", i/8), img)
+ SaveToPngFile(fmt.Sprintf("../output/curve/_test%d.png", i/8), img)
log.Printf("Num of points: %d\n", len(p.Points))
}
fmt.Println()
@@ -97,7 +97,7 @@ func TestQuadCurve(t *testing.T) {
raster.PolylineBresenham(img, image.Black, p.Points...)
//drawPoints(img, image.NRGBAColor{0, 0, 0, 0xff}, curve[:]...)
drawPoints(img, color.NRGBA{0, 0, 0, 0xff}, p.Points...)
- SaveToPngFile(fmt.Sprintf("test_results/_testQuad%d.png", i), img)
+ SaveToPngFile(fmt.Sprintf("../output/curve/_testQuad%d.png", i), img)
log.Printf("Num of points: %d\n", len(p.Points))
}
fmt.Println()
diff --git a/draw2dbase/flattener.go b/draw2dbase/flattener.go
index 70ec305..986c7ab 100644
--- a/draw2dbase/flattener.go
+++ b/draw2dbase/flattener.go
@@ -7,6 +7,12 @@ import (
"github.com/llgcode/draw2d"
)
+// Liner receive segment definition
+type Liner interface {
+ // LineTo Draw a line from the current position to the point (x, y)
+ LineTo(x, y float64)
+}
+
// Flattener receive segment definition
type Flattener interface {
// MoveTo Start a New line from the point (x, y)
diff --git a/draw2dimg/drawer.go b/draw2dimg/drawer.go
deleted file mode 100644
index 5e97344..0000000
--- a/draw2dimg/drawer.go
+++ /dev/null
@@ -1,80 +0,0 @@
-package draw2dimg
-
-import (
- "image"
- "image/draw"
-
- "code.google.com/p/freetype-go/freetype/raster"
- "code.google.com/p/freetype-go/freetype/truetype"
- "github.com/llgcode/draw2d"
- "github.com/llgcode/draw2d/draw2dbase"
-)
-
-type Drawer struct {
- matrix draw2d.Matrix
- img draw.Image
- painter Painter
- fillRasterizer *raster.Rasterizer
- strokeRasterizer *raster.Rasterizer
- glyphBuf *truetype.GlyphBuf
-}
-
-func NewDrawer(img *image.RGBA) *Drawer {
- width, height := img.Bounds().Dx(), img.Bounds().Dy()
- return &Drawer{
- draw2d.NewIdentityMatrix(),
- img,
- raster.NewRGBAPainter(img),
- raster.NewRasterizer(width, height),
- raster.NewRasterizer(width, height),
- truetype.NewGlyphBuf(),
- }
-}
-
-func (d *Drawer) Matrix() *draw2d.Matrix {
- return &d.matrix
-}
-
-func (d *Drawer) Fill(path *draw2d.Path, style draw2d.FillStyle) {
- switch fillStyle := style.(type) {
- case draw2d.SolidFillStyle:
- d.fillRasterizer.UseNonZeroWinding = fillStyle.FillRule == draw2d.FillRuleWinding
- d.painter.SetColor(fillStyle.Color)
- default:
- panic("FillStyle not supported")
- }
-
- flattener := draw2dbase.Transformer{d.matrix, draw2dbase.FtLineBuilder{d.fillRasterizer}}
-
- draw2dbase.Flatten(path, flattener, d.matrix.GetScale())
-
- d.fillRasterizer.Rasterize(d.painter)
- d.fillRasterizer.Clear()
-}
-
-func (d *Drawer) Stroke(path *draw2d.Path, style draw2d.StrokeStyle) {
- d.strokeRasterizer.UseNonZeroWinding = true
-
- stroker := draw2dbase.NewLineStroker(style.LineCap, style.LineJoin, draw2dbase.Transformer{d.matrix, draw2dbase.FtLineBuilder{d.strokeRasterizer}})
- stroker.HalfLineWidth = style.Width / 2
-
- var liner draw2dbase.Flattener
- if style.Dash != nil && len(style.Dash) > 0 {
- liner = draw2dbase.NewDashConverter(style.Dash, style.DashOffset, stroker)
- } else {
- liner = stroker
- }
-
- draw2dbase.Flatten(path, liner, d.matrix.GetScale())
-
- d.painter.SetColor(style.Color)
- d.strokeRasterizer.Rasterize(d.painter)
- d.strokeRasterizer.Clear()
-}
-
-func (d *Drawer) Text(text string, x, y float64, style draw2d.TextStyle) {
-
-}
-
-func (d *Drawer) Image(image image.Image, x, y float64, scaling draw2d.ImageScaling) {
-}
diff --git a/draw2dpdf/README.md b/draw2dpdf/README.md
new file mode 100644
index 0000000..6ac7e99
--- /dev/null
+++ b/draw2dpdf/README.md
@@ -0,0 +1,42 @@
+draw2d pdf
+==========
+
+Package draw2dpdf provides a graphic context that can draw vector graphics and text on pdf file with the [gofpdf](https://github.com/jung-kurt/gofpdf) package.
+
+Quick Start
+-----------
+
+The following Go code generates a simple drawing and saves it to a pdf document:
+```go
+// Initialize the graphic context on an RGBA image
+dest := draw2dpdf.NewPdf("L", "mm", "A4")
+gc := draw2d.NewGraphicContext(dest)
+
+// Set some properties
+gc.SetFillColor(color.RGBA{0x44, 0xff, 0x44, 0xff})
+gc.SetStrokeColor(color.RGBA{0x44, 0x44, 0x44, 0xff})
+gc.SetLineWidth(5)
+
+// Draw a closed shape
+gc.MoveTo(10, 10) // should always be called first for a new path
+gc.LineTo(100, 50)
+gc.QuadCurveTo(100, 10, 10, 10)
+gc.Close()
+gc.FillStroke()
+
+// Save to file
+draw2dpdf.SaveToPdfFile("hello.pdf", dest)
+```
+
+There are more examples here: https://github.com/llgcode/draw2d/tree/master/samples
+
+Alternative backends
+--------------------
+
+- Drawing on images is provided by the draw2d package.
+- Drawing on opengl is provided by the draw2dgl package.
+
+Acknowledgments
+---------------
+
+The pdf backend uses https://github.com/jung-kurt/gofpdf
\ No newline at end of file
diff --git a/draw2dpdf/doc.go b/draw2dpdf/doc.go
index b8ccdb4..922099c 100644
--- a/draw2dpdf/doc.go
+++ b/draw2dpdf/doc.go
@@ -2,7 +2,7 @@
// created: 26/06/2015 by Stani Michiels
// Package draw2dpdf provides a graphic context that can draw vector
-// graphics and text on pdf file.
+// graphics and text on pdf file with the gofpdf package.
//
// Quick Start
//
@@ -25,10 +25,12 @@
// gc.FillStroke()
//
// // Save to file
-// draw2dpdf.SaveToPdfFile(fn, dest)
+// draw2dpdf.SaveToPdfFile("hello.pdf", dest)
//
// There are more examples here:
-// https://github.com/llgcode/draw2d.samples
+// https://github.com/llgcode/draw2d/tree/master/samples
+//
+// Alternative backends
//
// Drawing on images is provided by the draw2d package.
// Drawing on opengl is provided by the draw2dgl package.
diff --git a/draw2dpdf/fileutil.go b/draw2dpdf/fileutil.go
index c3c8e66..349545d 100644
--- a/draw2dpdf/fileutil.go
+++ b/draw2dpdf/fileutil.go
@@ -1,3 +1,6 @@
+// Copyright 2015 The draw2d Authors. All rights reserved.
+// created: 26/06/2015 by Stani Michiels
+
package draw2dpdf
import "github.com/jung-kurt/gofpdf"
diff --git a/draw2dpdf/gc.go b/draw2dpdf/gc.go
index 2bd12c1..c4ddd7d 100644
--- a/draw2dpdf/gc.go
+++ b/draw2dpdf/gc.go
@@ -1,6 +1,6 @@
// Copyright 2015 The draw2d Authors. All rights reserved.
// created: 26/06/2015 by Stani Michiels
-// TODO: fonts, dpi
+// TODO: dashed line
package draw2dpdf
@@ -12,7 +12,6 @@ import (
"log"
"math"
"os"
- "path/filepath"
"strconv"
"code.google.com/p/freetype-go/freetype/truetype"
@@ -47,8 +46,14 @@ var (
// a page and set fill color to white.
func NewPdf(orientationStr, unitStr, sizeStr string) *gofpdf.Fpdf {
pdf := gofpdf.New(orientationStr, unitStr, sizeStr, draw2d.GetFontFolder())
+ // to be compatible with draw2d
+ pdf.SetMargins(0, 0, 0)
+ pdf.SetDrawColor(0, 0, 0)
+ pdf.SetFillColor(255, 255, 255)
+ pdf.SetLineCapStyle("round")
+ pdf.SetLineJoinStyle("round")
+ pdf.SetLineWidth(1)
pdf.AddPage()
- pdf.SetFillColor(255, 255, 255) // to be compatible with draw2d
return pdf
}
@@ -91,7 +96,7 @@ func NewGraphicContext(pdf *gofpdf.Fpdf) *GraphicContext {
// TODO: add type (tp) as parameter to argument list?
func (gc *GraphicContext) DrawImage(image image.Image) {
name := strconv.Itoa(int(imageCount))
- imageCount += 1
+ imageCount++
tp := "PNG" // "JPG", "JPEG", "PNG" and "GIF"
b := &bytes.Buffer{}
png.Encode(b, image)
@@ -108,7 +113,8 @@ func (gc *GraphicContext) Clear() {
clearRect(gc, 0, 0, width, height)
}
-// ClearRect draws a white rectangle over the specified area
+// ClearRect draws a white rectangle over the specified area.
+// Samples: line.
func (gc *GraphicContext) ClearRect(x1, y1, x2, y2 int) {
clearRect(gc, float64(x1), float64(y1), float64(x2), float64(y2))
}
@@ -134,18 +140,31 @@ func (gc *GraphicContext) GetDPI() int {
}
// GetStringBounds returns the approximate pixel bounds of the string s at x, y.
+// The left edge of the em square of the first character of s
+// and the baseline intersect at 0, 0 in the returned coordinates.
+// Therefore the top and left coordinates may well be negative.
func (gc *GraphicContext) GetStringBounds(s string) (left, top, right, bottom float64) {
_, h := gc.pdf.GetFontSize()
- return 0, 0, gc.pdf.GetStringWidth(s), h
+ d := gc.pdf.GetFontDesc("", "")
+ if d.Ascent == 0 {
+ // not defined (standard font?), use average of 81%
+ top = 0.81 * h
+ } else {
+ top = -float64(d.Ascent) * h / float64(d.Ascent-d.Descent)
+ }
+ return 0, top, gc.pdf.GetStringWidth(s), top + h
}
// CreateStringPath creates a path from the string s at x, y, and returns the string width.
func (gc *GraphicContext) CreateStringPath(text string, x, y float64) (cursor float64) {
- _, _, w, h := gc.GetStringBounds(text)
+ //fpdf uses the top left corner
+ left, top, right, bottom := gc.GetStringBounds(text)
+ w := right - left
+ h := bottom - top
+ // gc.pdf.SetXY(x, y-h) do not use this as y-h might be negative
margin := gc.pdf.GetCellMargin()
- gc.pdf.MoveTo(x-margin, y+margin-0.82*h)
+ gc.pdf.MoveTo(x-left-margin, y+top)
gc.pdf.CellFormat(w, h, text, "", 0, "BL", false, 0, "")
- // gc.pdf.Cell(w, h, text)
return w
}
@@ -173,29 +192,53 @@ func (gc *GraphicContext) StrokeStringAt(text string, x, y float64) (cursor floa
// Stroke strokes the paths with the color specified by SetStrokeColor
func (gc *GraphicContext) Stroke(paths ...*draw2d.Path) {
- gc.draw("D", paths...)
+ _, _, _, alphaS := gc.Current.StrokeColor.RGBA()
+ gc.draw("D", alphaS, paths...)
+ gc.Current.Path.Clear()
}
// Fill fills the paths with the color specified by SetFillColor
func (gc *GraphicContext) Fill(paths ...*draw2d.Path) {
- gc.draw("F", paths...)
+ style := "F"
+ if gc.Current.FillRule != draw2d.FillRuleWinding {
+ style += "*"
+ }
+ _, _, _, alphaF := gc.Current.FillColor.RGBA()
+ gc.draw(style, alphaF, paths...)
+ gc.Current.Path.Clear()
}
// FillStroke first fills the paths and than strokes them
func (gc *GraphicContext) FillStroke(paths ...*draw2d.Path) {
- gc.draw("FD", paths...)
+ var rule string
+ if gc.Current.FillRule != draw2d.FillRuleWinding {
+ rule = "*"
+ }
+ _, _, _, alphaS := gc.Current.StrokeColor.RGBA()
+ _, _, _, alphaF := gc.Current.FillColor.RGBA()
+ if alphaS == alphaF {
+ gc.draw("FD"+rule, alphaF, paths...)
+ } else {
+ gc.draw("F"+rule, alphaF, paths...)
+ gc.draw("S", alphaS, paths...)
+ }
+ gc.Current.Path.Clear()
}
var logger = log.New(os.Stdout, "", log.Lshortfile)
+const alphaMax = float64(0xFFFF)
+
// draw fills and/or strokes paths
-func (gc *GraphicContext) draw(style string, paths ...*draw2d.Path) {
+func (gc *GraphicContext) draw(style string, alpha uint32, paths ...*draw2d.Path) {
paths = append(paths, gc.Current.Path)
for _, p := range paths {
ConvertPath(p, gc.pdf)
}
- if gc.Current.FillRule == draw2d.FillRuleWinding {
- style += "*"
+ a := float64(alpha) / alphaMax
+ current, blendMode := gc.pdf.GetAlpha()
+ if a != current {
+ gc.pdf.SetAlpha(a, blendMode)
}
gc.pdf.DrawPath(style)
}
@@ -242,8 +285,9 @@ func (gc *GraphicContext) SetFontData(fontData draw2d.FontData) {
}
fn := draw2d.FontFileName(fontData)
fn = fn[:len(fn)-4]
- jfn := filepath.Join(draw2d.GetFontFolder(), fn+".json")
- gc.pdf.AddFont(fn, style, jfn)
+ size, _ := gc.pdf.GetFontSize()
+ gc.pdf.AddFont(fontData.Name, style, fn+".json")
+ gc.pdf.SetFont(fontData.Name, style, size)
}
// SetFontSize sets the font size in points (as in ``a 12 point font'').
@@ -254,6 +298,12 @@ func (gc *GraphicContext) SetFontSize(fontSize float64) {
gc.pdf.SetFontSize(fontSize * gc.Current.Scale)
}
+// SetLineDash sets the line dash pattern
+func (gc *GraphicContext) SetLineDash(Dash []float64, DashOffset float64) {
+ gc.StackGraphicContext.SetLineDash(Dash, DashOffset)
+ gc.pdf.SetDashPattern(Dash, DashOffset)
+}
+
// SetLineWidth sets the line width
func (gc *GraphicContext) SetLineWidth(LineWidth float64) {
gc.StackGraphicContext.SetLineWidth(LineWidth)
diff --git a/draw2dpdf/samples_test.go b/draw2dpdf/samples_test.go
index 5ceeb3c..ca3441f 100644
--- a/draw2dpdf/samples_test.go
+++ b/draw2dpdf/samples_test.go
@@ -1,3 +1,5 @@
+// Copyright 2015 The draw2d Authors. All rights reserved.
+// created: 26/06/2015 by Stani Michiels
// See also test_test.go
package draw2dpdf_test
@@ -6,29 +8,43 @@ import (
"testing"
"github.com/llgcode/draw2d"
- "github.com/llgcode/draw2d.samples"
- "github.com/llgcode/draw2d.samples/android"
- "github.com/llgcode/draw2d.samples/frameimage"
- "github.com/llgcode/draw2d.samples/gopher"
- "github.com/llgcode/draw2d.samples/helloworld"
- "github.com/llgcode/draw2d.samples/line"
- "github.com/llgcode/draw2d.samples/linecapjoin"
- "github.com/llgcode/draw2d.samples/postscript"
+ "github.com/llgcode/draw2d/samples/android"
+ "github.com/llgcode/draw2d/samples/frameimage"
+ "github.com/llgcode/draw2d/samples/geometry"
+ "github.com/llgcode/draw2d/samples/gopher"
+ "github.com/llgcode/draw2d/samples/gopher2"
+ "github.com/llgcode/draw2d/samples/helloworld"
+ "github.com/llgcode/draw2d/samples/line"
+ "github.com/llgcode/draw2d/samples/linecapjoin"
+ "github.com/llgcode/draw2d/samples/postscript"
)
func TestSampleAndroid(t *testing.T) {
test(t, android.Main)
}
+// TODO: FillString: w (width) is incorrect
+func TestSampleGeometry(t *testing.T) {
+ // Set the global folder for searching fonts
+ // The pdf backend needs for every ttf file its corresponding
+ // json/.z file which is generated by gofpdf/makefont.
+ draw2d.SetFontFolder("../resource/font")
+ test(t, geometry.Main)
+}
+
func TestSampleGopher(t *testing.T) {
test(t, gopher.Main)
}
+func TestSampleGopher2(t *testing.T) {
+ test(t, gopher2.Main)
+}
+
func TestSampleHelloWorld(t *testing.T) {
// Set the global folder for searching fonts
- // The pdf backend needs for every ttf file its corresponding json
- // file which is generated by gofpdf/makefont.
- draw2d.SetFontFolder(samples.Dir("helloworld", "../"))
+ // The pdf backend needs for every ttf file its corresponding
+ // json/.z file which is generated by gofpdf/makefont.
+ draw2d.SetFontFolder("../resource/font")
test(t, helloworld.Main)
}
diff --git a/draw2dpdf/test_test.go b/draw2dpdf/test_test.go
index a763c59..801ff36 100644
--- a/draw2dpdf/test_test.go
+++ b/draw2dpdf/test_test.go
@@ -1,10 +1,12 @@
+// Copyright 2015 The draw2d Authors. All rights reserved.
+// created: 26/06/2015 by Stani Michiels
+
// Package draw2dpdf_test gives test coverage with the command:
// go test -cover ./... | grep -v "no test"
// (It should be run from its parent draw2d directory.)
package draw2dpdf_test
import (
- "os"
"testing"
"github.com/llgcode/draw2d"
@@ -18,18 +20,20 @@ func test(t *testing.T, draw sample) {
dest := draw2dpdf.NewPdf("L", "mm", "A4")
gc := draw2dpdf.NewGraphicContext(dest)
// Draw sample
- fn, err := draw(gc, "pdf")
+ output, err := draw(gc, "pdf")
if err != nil {
- t.Errorf("Drawing %q failed: %v", fn, err)
+ t.Errorf("Drawing %q failed: %v", output, err)
return
}
- // Save to pdf only if it doesn't exist because of git
- if _, err = os.Stat(fn); err == nil {
- t.Skipf("Saving %q skipped, as it exists already. (Git would consider it modified.)", fn)
- return
- }
- err = draw2dpdf.SaveToPdfFile(fn, dest)
+ /*
+ // Save to pdf only if it doesn't exist because of git
+ if _, err = os.Stat(output); err == nil {
+ t.Skipf("Saving %q skipped, as it exists already. (Git would consider it modified.)", output)
+ return
+ }
+ */
+ err = draw2dpdf.SaveToPdfFile(output, dest)
if err != nil {
- t.Errorf("Saving %q failed: %v", fn, err)
+ t.Errorf("Saving %q failed: %v", output, err)
}
}
diff --git a/font.go b/font.go
index e62ef28..279706c 100644
--- a/font.go
+++ b/font.go
@@ -7,13 +7,15 @@ import (
"io/ioutil"
"log"
"path"
+ "path/filepath"
"code.google.com/p/freetype-go/freetype/truetype"
)
var (
- fontFolder = "../resource/font/"
- fonts = make(map[string]*truetype.Font)
+ fontFolder = "../resource/font/"
+ fonts = make(map[string]*truetype.Font)
+ fontNamer FontFileNamer = FontFileName
)
type FontStyle byte
@@ -38,6 +40,8 @@ type FontData struct {
Style FontStyle
}
+type FontFileNamer func(fontData FontData) string
+
func FontFileName(fontData FontData) string {
fontFileName := fontData.Name
switch fontData.Family {
@@ -62,11 +66,11 @@ func FontFileName(fontData FontData) string {
}
func RegisterFont(fontData FontData, font *truetype.Font) {
- fonts[FontFileName(fontData)] = font
+ fonts[fontNamer(fontData)] = font
}
func GetFont(fontData FontData) *truetype.Font {
- fontFileName := FontFileName(fontData)
+ fontFileName := fontNamer(fontData)
font := fonts[fontFileName]
if font != nil {
return font
@@ -80,7 +84,11 @@ func GetFontFolder() string {
}
func SetFontFolder(folder string) {
- fontFolder = folder
+ fontFolder = filepath.Clean(folder)
+}
+
+func SetFontNamer(fn FontFileNamer) {
+ fontNamer = fn
}
func loadFont(fontFileName string) *truetype.Font {
diff --git a/output/README.md b/output/README.md
new file mode 100644
index 0000000..037f0e2
--- /dev/null
+++ b/output/README.md
@@ -0,0 +1,11 @@
+Demo output
+===========
+
+These folders are empty when you check out the git repository. The output is generated by the tests:
+```
+go test ./...
+```
+or with coverage:
+```
+go test -cover ./... | grep -v "no test"
+```
diff --git a/output/curve/.gitignore b/output/curve/.gitignore
new file mode 100644
index 0000000..86d0cb2
--- /dev/null
+++ b/output/curve/.gitignore
@@ -0,0 +1,4 @@
+# Ignore everything in this directory
+*
+# Except this file
+!.gitignore
\ No newline at end of file
diff --git a/output/raster/.gitignore b/output/raster/.gitignore
new file mode 100644
index 0000000..86d0cb2
--- /dev/null
+++ b/output/raster/.gitignore
@@ -0,0 +1,4 @@
+# Ignore everything in this directory
+*
+# Except this file
+!.gitignore
\ No newline at end of file
diff --git a/output/samples/.gitignore b/output/samples/.gitignore
new file mode 100644
index 0000000..e39d62f
--- /dev/null
+++ b/output/samples/.gitignore
@@ -0,0 +1,6 @@
+# Ignore everything in this directory
+*
+# Except this file
+!.gitignore
+!geometry.png
+!postscript.png
\ No newline at end of file
diff --git a/output/samples/geometry.png b/output/samples/geometry.png
new file mode 100644
index 0000000..7113b44
Binary files /dev/null and b/output/samples/geometry.png differ
diff --git a/output/samples/postscript.png b/output/samples/postscript.png
new file mode 100644
index 0000000..373442d
Binary files /dev/null and b/output/samples/postscript.png differ
diff --git a/raster/raster_test.go b/raster/raster_test.go
index ac1dd57..48b8d5e 100644
--- a/raster/raster_test.go
+++ b/raster/raster_test.go
@@ -1,39 +1,16 @@
package raster
import (
- "bufio"
"image"
"image/color"
- "image/png"
- "log"
- "os"
"testing"
"code.google.com/p/freetype-go/freetype/raster"
- "github.com/llgcode/draw2d/curve"
+ "github.com/llgcode/draw2d/draw2dbase"
+ "github.com/llgcode/draw2d/draw2dimg"
)
-var flattening_threshold float64 = 0.5
-
-func savepng(filePath string, m image.Image) {
- f, err := os.Create(filePath)
- if err != nil {
- log.Println(err)
- os.Exit(1)
- }
- defer f.Close()
- b := bufio.NewWriter(f)
- err = png.Encode(b, m)
- if err != nil {
- log.Println(err)
- os.Exit(1)
- }
- err = b.Flush()
- if err != nil {
- log.Println(err)
- os.Exit(1)
- }
-}
+var flatteningThreshold = 0.5
type Path struct {
points []float64
@@ -54,53 +31,59 @@ func (p *Path) LineTo(x, y float64) {
func TestFreetype(t *testing.T) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
rasterizer := raster.NewRasterizer(200, 200)
rasterizer.UseNonZeroWinding = false
- rasterizer.Start(raster.Point{raster.Fix32(10 * 256), raster.Fix32(190 * 256)})
+ rasterizer.Start(raster.Point{
+ X: raster.Fix32(10 * 256),
+ Y: raster.Fix32(190 * 256)})
for j := 0; j < len(poly); j = j + 2 {
- rasterizer.Add1(raster.Point{raster.Fix32(poly[j] * 256), raster.Fix32(poly[j+1] * 256)})
+ rasterizer.Add1(raster.Point{
+ X: raster.Fix32(poly[j] * 256),
+ Y: raster.Fix32(poly[j+1] * 256)})
}
painter := raster.NewRGBAPainter(img)
painter.SetColor(color)
rasterizer.Rasterize(painter)
- savepng("_testFreetype.png", img)
+ draw2dimg.SaveToPngFile("../output/raster/TestFreetype.png", img)
}
func TestFreetypeNonZeroWinding(t *testing.T) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
rasterizer := raster.NewRasterizer(200, 200)
rasterizer.UseNonZeroWinding = true
- rasterizer.Start(raster.Point{raster.Fix32(10 * 256), raster.Fix32(190 * 256)})
+ rasterizer.Start(raster.Point{
+ X: raster.Fix32(10 * 256),
+ Y: raster.Fix32(190 * 256)})
for j := 0; j < len(poly); j = j + 2 {
- rasterizer.Add1(raster.Point{raster.Fix32(poly[j] * 256), raster.Fix32(poly[j+1] * 256)})
+ rasterizer.Add1(raster.Point{
+ X: raster.Fix32(poly[j] * 256),
+ Y: raster.Fix32(poly[j+1] * 256)})
}
painter := raster.NewRGBAPainter(img)
painter.SetColor(color)
rasterizer.Rasterize(painter)
- savepng("_testFreetypeNonZeroWinding.png", img)
+ draw2dimg.SaveToPngFile("../output/raster/TestFreetypeNonZeroWinding.png", img)
}
func TestRasterizer(t *testing.T) {
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
tr := [6]float64{1, 0, 0, 1, 0, 0}
@@ -108,15 +91,15 @@ func TestRasterizer(t *testing.T) {
//PolylineBresenham(img, image.Black, poly...)
r.RenderEvenOdd(img, &color, &poly, tr)
- savepng("_testRasterizer.png", img)
+ draw2dimg.SaveToPngFile("../output/raster/TestRasterizer.png", img)
}
func TestRasterizerNonZeroWinding(t *testing.T) {
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
tr := [6]float64{1, 0, 0, 1, 0, 0}
@@ -124,14 +107,14 @@ func TestRasterizerNonZeroWinding(t *testing.T) {
//PolylineBresenham(img, image.Black, poly...)
r.RenderNonZeroWinding(img, &color, &poly, tr)
- savepng("_testRasterizerNonZeroWinding.png", img)
+ draw2dimg.SaveToPngFile("../output/raster/TestRasterizerNonZeroWinding.png", img)
}
func BenchmarkFreetype(b *testing.B) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
@@ -139,20 +122,25 @@ func BenchmarkFreetype(b *testing.B) {
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
rasterizer := raster.NewRasterizer(200, 200)
rasterizer.UseNonZeroWinding = false
- rasterizer.Start(raster.Point{raster.Fix32(10 * 256), raster.Fix32(190 * 256)})
+ rasterizer.Start(raster.Point{
+ X: raster.Fix32(10 * 256),
+ Y: raster.Fix32(190 * 256)})
for j := 0; j < len(poly); j = j + 2 {
- rasterizer.Add1(raster.Point{raster.Fix32(poly[j] * 256), raster.Fix32(poly[j+1] * 256)})
+ rasterizer.Add1(raster.Point{
+ X: raster.Fix32(poly[j] * 256),
+ Y: raster.Fix32(poly[j+1] * 256)})
}
painter := raster.NewRGBAPainter(img)
painter.SetColor(color)
rasterizer.Rasterize(painter)
}
}
+
func BenchmarkFreetypeNonZeroWinding(b *testing.B) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
@@ -160,9 +148,13 @@ func BenchmarkFreetypeNonZeroWinding(b *testing.B) {
img := image.NewRGBA(image.Rect(0, 0, 200, 200))
rasterizer := raster.NewRasterizer(200, 200)
rasterizer.UseNonZeroWinding = true
- rasterizer.Start(raster.Point{raster.Fix32(10 * 256), raster.Fix32(190 * 256)})
+ rasterizer.Start(raster.Point{
+ X: raster.Fix32(10 * 256),
+ Y: raster.Fix32(190 * 256)})
for j := 0; j < len(poly); j = j + 2 {
- rasterizer.Add1(raster.Point{raster.Fix32(poly[j] * 256), raster.Fix32(poly[j+1] * 256)})
+ rasterizer.Add1(raster.Point{
+ X: raster.Fix32(poly[j] * 256),
+ Y: raster.Fix32(poly[j+1] * 256)})
}
painter := raster.NewRGBAPainter(img)
painter.SetColor(color)
@@ -173,8 +165,8 @@ func BenchmarkFreetypeNonZeroWinding(b *testing.B) {
func BenchmarkRasterizerNonZeroWinding(b *testing.B) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
tr := [6]float64{1, 0, 0, 1, 0, 0}
@@ -188,8 +180,8 @@ func BenchmarkRasterizerNonZeroWinding(b *testing.B) {
func BenchmarkRasterizer(b *testing.B) {
var p Path
p.LineTo(10, 190)
- c := curve.CubicCurveFloat64{10, 190, 10, 10, 190, 10, 190, 190}
- c.Trace(&p, flattening_threshold)
+ draw2dbase.TraceCubic(&p, []float64{10, 190, 10, 10, 190, 10, 190, 190}, 0.5)
+
poly := Polygon(p.points)
color := color.RGBA{0, 0, 0, 0xff}
tr := [6]float64{1, 0, 0, 1, 0, 0}
diff --git a/resource/font/luximbi.json b/resource/font/luximbi.json
new file mode 100644
index 0000000..cc5cd35
--- /dev/null
+++ b/resource/font/luximbi.json
@@ -0,0 +1 @@
+{"Tp":"TrueType","Name":"LuxiMono-BoldOblique","Desc":{"Ascent":783,"Descent":-205,"CapHeight":783,"Flags":97,"FontBBox":{"Xmin":-29,"Ymin":-211,"Xmax":764,"Ymax":1012},"ItalicAngle":-8,"StemV":120,"MissingWidth":600},"Up":0,"Ut":0,"Cw":[600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,653,600,653,600,600,653,600,653,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600,600],"Enc":"cp1252","Diff":"","File":"luximbi.z","Size1":0,"Size2":0,"OriginalSize":69872,"I":0,"N":0,"DiffN":0}
\ No newline at end of file
diff --git a/resource/font/luximbi.z b/resource/font/luximbi.z
new file mode 100644
index 0000000..4dfb443
Binary files /dev/null and b/resource/font/luximbi.z differ
diff --git a/resource/image/geometry.pdf b/resource/image/geometry.pdf
new file mode 100644
index 0000000..755e4ea
Binary files /dev/null and b/resource/image/geometry.pdf differ
diff --git a/resource/image/gopher.png b/resource/image/gopher.png
new file mode 100644
index 0000000..8598723
Binary files /dev/null and b/resource/image/gopher.png differ
diff --git a/resource/image/postscript.pdf b/resource/image/postscript.pdf
new file mode 100644
index 0000000..d4b60be
Binary files /dev/null and b/resource/image/postscript.pdf differ
diff --git a/resource/image/tiger.ps b/resource/image/tiger.ps
new file mode 100644
index 0000000..24a9dce
--- /dev/null
+++ b/resource/image/tiger.ps
@@ -0,0 +1,2733 @@
+%!PS-Adobe-2.0 EPSF-1.2
+%%Creator: Adobe Illustrator(TM) 1.2d4
+%%For: OpenWindows Version 2
+%%Title: tiger.eps
+%%CreationDate: 4/12/90 3:20 AM
+%%DocumentProcSets: Adobe_Illustrator_1.2d1 0 0
+%%DocumentSuppliedProcSets: Adobe_Illustrator_1.2d1 0 0
+%%BoundingBox: 22 171 567 738
+%%EndComments
+
+%%BeginProcSet:Adobe_Illustrator_1.2d1 0 0
+
+/Adobe_Illustrator_1.2d1 dup 100 dict def load begin
+% definition operators
+/bdef {bind def} bind def
+/ldef {load def} bdef
+/xdef {exch def} bdef
+% graphic state operators
+/_K { 3 index add neg dup 0 lt {pop 0} if 3 1 roll } bdef
+/_k /setcmybcolor where {
+ /setcmybcolor get
+} {
+ { 1 sub 4 1 roll _K _K _K setrgbcolor pop } bind
+} ifelse def
+/g {/_b xdef /p {_b setgray} def} bdef
+/G {/_B xdef /P {_B setgray} def} bdef
+/k {/_b xdef /_y xdef /_m xdef /_c xdef /p {_c _m _y _b _k} def} bdef
+/K {/_B xdef /_Y xdef /_M xdef /_C xdef /P {_C _M _Y _B _k} def} bdef
+/d /setdash ldef
+/_i currentflat def
+/i {dup 0 eq {pop _i} if setflat} bdef
+/j /setlinejoin ldef
+/J /setlinecap ldef
+/M /setmiterlimit ldef
+/w /setlinewidth ldef
+% path construction operators
+/_R {.25 sub round .25 add} bdef
+/_r {transform _R exch _R exch itransform} bdef
+/c {_r curveto} bdef
+/C /c ldef
+/v {currentpoint 6 2 roll _r curveto} bdef
+/V /v ldef
+/y {_r 2 copy curveto} bdef
+/Y /y ldef
+/l {_r lineto} bdef
+/L /l ldef
+/m {_r moveto} bdef
+% path painting operators
+/n /newpath ldef
+/N /n ldef
+/F {p fill} bdef
+/f {closepath F} bdef
+/S {P stroke} bdef
+/s {closepath S} bdef
+/B {gsave F grestore S} bdef
+/b {closepath B} bdef
+end
+%%EndProcSet
+%%EndProlog
+
+%%Page: 1 1
+
+Adobe_Illustrator_1.2d1 begin
+
+.8 setgray
+clippath fill
+-110 -300 translate
+1.1 dup scale
+
+0 g
+0 G
+0 i
+0 J
+0 j
+0.172 w
+10 M
+[]0 d
+0 0 0 0 k
+
+177.696 715.715 m
+177.797 713.821 176.973 713.84 v
+176.149 713.859 159.695 761.934 139.167 759.691 C
+156.95 767.044 177.696 715.715 V
+b
+181.226 718.738 m
+180.677 716.922 179.908 717.221 v
+179.14 717.519 180.023 768.325 159.957 773.199 C
+179.18 774.063 181.226 718.738 V
+b
+208.716 676.41 m
+210.352 675.45 209.882 674.773 v
+209.411 674.096 160.237 686.898 150.782 668.541 C
+154.461 687.428 208.716 676.41 V
+b
+205.907 666.199 m
+207.763 665.803 207.529 665.012 v
+207.296 664.221 156.593 660.879 153.403 640.478 C
+150.945 659.563 205.907 666.199 V
+b
+201.696 671.724 m
+203.474 671.061 203.128 670.313 v
+202.782 669.565 152.134 673.654 146.002 653.936 C
+146.354 673.175 201.696 671.724 V
+b
+190.991 689.928 m
+192.299 688.554 191.66 688.033 v
+191.021 687.512 147.278 713.366 133.131 698.324 C
+141.872 715.467 190.991 689.928 V
+b
+183.446 685.737 m
+184.902 684.52 184.326 683.929 v
+183.75 683.339 137.362 704.078 125.008 687.531 C
+131.753 705.553 183.446 685.737 V
+b
+180.846 681.665 m
+182.454 680.657 181.964 679.994 v
+181.474 679.331 132.692 693.554 122.709 675.478 C
+126.934 694.251 180.846 681.665 V
+b
+191.58 681.051 m
+192.702 679.52 192.001 679.085 v
+191.3 678.65 151.231 709.898 135.273 696.793 C
+146.138 712.674 191.58 681.051 V
+b
+171.8 710 m
+172.4 708.2 171.6 708 v
+170.8 707.8 142.2 749.8 122.999 742.2 C
+138.2 754 171.8 710 V
+b
+172.495 703.021 m
+173.47 701.392 172.731 701.025 v
+171.993 700.657 135.008 735.501 117.899 723.939 C
+130.196 738.739 172.495 703.021 V
+b
+172.38 698.651 m
+173.502 697.12 172.801 696.685 v
+172.1 696.251 132.031 727.498 116.073 714.393 C
+126.938 730.274 172.38 698.651 V
+b
+0 J 1 w
+170.17 696.935 m
+170.673 690.887 171.661 684.318 173.4 681.199 C
+169.8 668.799 178.6 655.599 V
+178.2 648.399 179.8 645.199 V
+183.8 636.799 188.6 635.999 v
+192.484 635.352 201.207 632.283 211.068 630.879 c
+228.2 616.799 225 603.999 V
+224.6 587.599 221 585.999 V
+232.6 597.199 223 580.399 V
+218.6 561.599 l
+244.2 583.199 228.6 564.799 V
+218.6 538.799 l
+238.2 557.199 231 548.799 V
+227.8 539.999 l
+271 567.199 240.2 537.599 V
+248.2 541.199 252.6 538.399 V
+259.4 539.599 258.6 537.999 V
+237.8 527.599 234.2 509.199 V
+242.6 519.199 239.4 508.399 V
+239.8 496.799 l
+243.8 518.399 243.4 480.799 V
+262.6 498.799 251 477.999 V
+251 461.199 l
+266.2 477.599 259.8 464.799 V
+269.8 473.599 265.8 458.399 V
+265 447.999 269.4 459.199 V
+285.4 489.799 279.4 463.599 V
+278.6 444.399 283.4 459.199 V
+283.8 448.799 293 441.599 V
+291.8 492.399 304.6 456.399 V
+308.6 439.999 l
+311.4 449.199 311 454.399 V
+325.8 470.799 319 446.399 V
+334.2 469.199 331 455.999 V
+323.4 439.999 325 435.199 V
+341.8 469.999 343 471.599 V
+341 429.198 351.8 465.199 V
+357.4 453.199 354.6 448.799 V
+362.6 456.799 361.8 459.999 V
+366.4 468.199 369.2 454.599 V
+371 445.199 372.6 448.399 V
+376.6 424.398 377.8 447.199 V
+379.4 460.799 372.2 472.399 V
+373 475.599 370.2 479.599 v
+383.8 457.999 376.6 486.799 V
+387.801 478.799 389.001 478.799 V
+375.4 501.999 384.2 497.199 V
+379 507.599 397.001 495.599 V
+381 511.599 398.601 501.999 V
+406.601 495.599 399.001 505.599 V
+384.6 521.599 406.601 503.599 V
+418.201 487.199 419.001 484.399 V
+409.001 513.599 404.601 516.399 V
+413.001 552.799 454.201 537.199 V
+461.001 519.999 465.401 538.399 V
+478.201 544.799 489.401 517.199 V
+493.401 530.799 492.601 533.599 V
+499.401 532.399 498.601 533.599 V
+511.801 529.199 513.001 529.999 V
+519.801 523.199 520.201 526.799 V
+529.401 523.999 527.401 527.599 V
+536.201 511.999 536.601 508.399 V
+539.001 522.399 l
+541.001 519.599 l
+542.601 527.199 541.801 528.399 v
+541.001 529.599 561.801 521.599 566.601 500.799 C
+568.601 492.399 l
+574.601 507.199 573.001 511.199 V
+578.201 510.399 578.601 505.999 V
+582.601 529.199 577.801 535.199 V
+582.201 535.999 583.401 532.399 V
+583.401 539.599 l
+590.601 538.799 590.601 541.199 V
+595.001 545.199 597.001 540.399 V
+584.601 575.599 603.001 556.399 V
+610.201 545.599 606.601 564.399 v
+603.001 583.199 599.001 584.799 603.801 585.199 C
+604.601 588.799 602.601 590.399 v
+600.601 591.999 603.801 590.399 y
+608.601 586.399 603.401 608.399 V
+609.801 606.799 597.801 635.999 V
+600.601 638.399 596.601 646.799 V
+604.601 642.399 607.401 643.999 V
+607.001 645.599 603.801 649.599 V
+582.201 704.4 602.601 682.399 V
+614.451 668.849 608.051 691.649 V
+598.94 715.659 599.717 719.955 V
+170.17 696.935 l
+b
+0.2 0.55 0.85 0 k
+599.717 719.755 m
+600.345 719.574 602.551 718.45 603.801 716.8 C
+610.601 706 605.401 724.4 V
+596.201 753.2 605.001 742 V
+611.001 734.8 607.801 748.4 v
+603.936 764.827 601.401 771.2 y
+613.001 766.4 586.201 806 V
+595.001 802.4 l
+575.401 842 553.801 847.2 V
+545.801 853.2 l
+584.201 891.2 571.401 928 V
+564.601 933.2 555.001 924 V
+548.601 919.2 542.601 920.8 V
+511.801 919.6 509.801 919.6 v
+507.801 919.6 473.001 956.8 407.401 939.2 C
+402.201 937.2 397.801 938.4 V
+379.4 954.4 330.6 931.6 v
+320.6 929.6 319 929.6 v
+317.4 929.6 314.6 929.6 306.6 923.2 c
+298.6 916.8 298.2 916 296.2 914.4 C
+279.8 903.2 275 902.4 V
+263.4 896 259 886 V
+255.4 884.8 l
+253.8 877.6 253.4 876.4 V
+248.6 872.8 247.8 867.2 V
+239 861.2 239.4 856.8 V
+237.8 851.6 237 846.8 V
+229.8 842 230.6 839.2 V
+223 825.2 224.2 818.4 V
+217.8 818.8 215 816.4 V
+214.2 811.6 212.6 811.2 V
+209.8 810 212.2 806 V
+210.6 803.2 210.2 801.6 V
+211 798.8 206.6 793.2 V
+200.2 774.4 202.2 769.2 V
+202.6 764.4 199.8 762.8 V
+196.2 763.2 204.6 751.2 V
+205.4 750 202.2 747.6 V
+185 744 182.6 727.6 V
+169 712.8 169 707.6 v
+169 705.295 169.271 702.148 169.97 697.535 C
+169.4 689.199 197 688.399 v
+224.6 687.599 599.717 719.755 Y
+b
+184.4 697.4 m
+159.4 736.8 173.8 680.399 Y
+182.6 645.999 312.2 683.599 y
+481.001 714 492.201 718 v
+503.401 722 598.601 715.6 y
+593.001 732.4 L
+528.201 778.8 509.001 755.6 495.401 759.6 c
+481.801 763.6 484.201 754 481.001 753.2 c
+477.801 752.4 438.601 777.2 432.201 776.4 c
+425.801 775.6 400.459 799.351 415.401 767.6 c
+431.401 733.6 357 728.4 340.2 739.6 c
+323.4 750.8 347.4 721.2 Y
+365.8 701.2 331.4 718 y
+297 730.8 273 705.2 269.8 704.4 c
+266.6 703.6 261.8 700.4 261 706.8 c
+260.2 713.2 252.69 729.901 221 703.6 c
+201 686.999 187.2 709 Y
+184.4 697.4 L
+f
+0.09 0.5 0.772 0 k
+433.51 774.654 m
+427.11 773.854 401.743 797.593 416.71 765.854 c
+433.31 730.654 358.31 726.654 341.51 737.854 c
+324.709 749.054 348.71 719.454 Y
+367.11 699.454 332.709 716.254 y
+298.309 729.054 274.309 703.454 271.109 702.654 c
+267.909 701.854 263.109 698.654 262.309 705.054 c
+261.509 711.454 254.13 727.988 222.309 701.854 c
+201.073 684.508 187.582 705.963 Y
+184.382 695.854 L
+159.382 735.654 174.454 677.345 Y
+183.255 642.944 313.509 681.854 y
+482.31 712.254 493.51 716.254 v
+504.71 720.254 599.038 713.927 y
+593.51 731.236 L
+528.71 777.636 510.31 753.854 496.71 757.854 c
+483.11 761.854 485.51 752.254 482.31 751.454 c
+479.11 750.654 439.91 775.454 433.51 774.654 c
+f
+0.081 0.45 0.695 0 k
+434.819 772.909 m
+428.419 772.109 403.685 796.138 418.019 764.109 c
+434.219 727.908 359.619 724.908 342.819 736.108 c
+326.019 747.308 350.019 717.708 Y
+368.419 697.708 334.019 714.508 y
+299.619 727.308 275.618 701.708 272.418 700.908 c
+269.218 700.108 264.418 696.908 263.618 703.308 c
+262.818 709.708 255.57 726.075 223.618 700.108 c
+201.145 682.017 187.964 702.926 Y
+184.364 694.308 L
+160.564 733.308 175.109 674.29 Y
+183.909 639.89 314.819 680.108 y
+483.619 710.508 494.819 714.508 v
+506.019 718.508 599.474 712.254 y
+594.02 730.072 L
+529.219 776.472 511.619 752.109 498.019 756.109 c
+484.419 760.109 486.819 750.509 483.619 749.708 c
+480.419 748.908 441.219 773.709 434.819 772.909 c
+f
+0.072 0.4 0.618 0 k
+436.128 771.163 m
+429.728 770.363 404.999 794.395 419.328 762.363 c
+436.128 724.807 360.394 723.518 344.128 734.363 c
+327.328 745.563 351.328 715.963 Y
+369.728 695.963 335.328 712.763 y
+300.928 725.563 276.928 699.963 273.728 699.163 c
+270.528 698.363 265.728 695.163 264.928 701.563 c
+264.128 707.963 257.011 724.161 224.927 698.363 c
+201.218 679.526 188.345 699.89 Y
+184.345 692.763 L
+162.545 729.563 175.764 671.235 Y
+184.564 636.835 316.128 678.363 y
+484.928 708.763 496.129 712.763 v
+507.329 716.763 599.911 710.581 y
+594.529 728.908 L
+529.729 775.309 512.929 750.363 499.329 754.363 c
+485.728 758.363 488.128 748.763 484.928 747.963 c
+481.728 747.163 442.528 771.963 436.128 771.163 c
+f
+0.063 0.35 0.54 0 k
+437.438 769.417 m
+431.037 768.617 406.814 792.871 420.637 760.617 c
+437.438 721.417 362.237 721.417 345.437 732.617 c
+328.637 743.817 352.637 714.217 Y
+371.037 694.217 336.637 711.017 y
+302.237 723.817 278.237 698.217 275.037 697.417 c
+271.837 696.617 267.037 693.417 266.237 699.817 c
+265.437 706.217 258.452 722.248 226.237 696.617 c
+201.291 677.035 188.727 696.854 Y
+184.327 691.217 L
+164.527 726.018 176.418 668.181 Y
+185.218 633.78 317.437 676.617 y
+486.238 707.017 497.438 711.017 v
+508.638 715.017 600.347 708.908 y
+595.038 727.745 L
+530.238 774.145 514.238 748.617 500.638 752.617 c
+487.038 756.617 489.438 747.017 486.238 746.217 c
+483.038 745.417 443.838 770.217 437.438 769.417 c
+f
+0.054 0.3 0.463 0 k
+438.747 767.672 m
+432.347 766.872 406.383 790.323 421.947 758.872 c
+441.147 720.072 363.546 719.672 346.746 730.872 c
+329.946 742.072 353.946 712.472 Y
+372.346 692.472 337.946 709.272 y
+303.546 722.072 279.546 696.472 276.346 695.672 c
+273.146 694.872 268.346 691.672 267.546 698.072 c
+266.746 704.472 259.892 720.335 227.546 694.872 c
+201.364 674.544 189.109 693.817 Y
+184.309 689.672 L
+166.309 722.872 177.073 665.126 Y
+185.873 630.726 318.746 674.872 y
+487.547 705.272 498.747 709.272 v
+509.947 713.272 600.783 707.236 y
+595.547 726.581 L
+530.747 772.981 515.547 746.872 501.947 750.872 c
+488.347 754.872 490.747 745.272 487.547 744.472 c
+484.347 743.672 445.147 768.472 438.747 767.672 c
+f
+0.045 0.25 0.386 0 k
+440.056 765.927 m
+433.655 765.127 407.313 788.387 423.255 757.127 c
+443.656 717.126 364.855 717.926 348.055 729.126 c
+331.255 740.326 355.255 710.726 Y
+373.655 690.726 339.255 707.526 y
+304.855 720.326 280.855 694.726 277.655 693.926 c
+274.455 693.126 269.655 689.926 268.855 696.326 c
+268.055 702.726 261.332 718.422 228.855 693.126 c
+201.436 672.053 189.491 690.781 Y
+184.291 688.126 L
+168.291 718.326 177.727 662.071 Y
+186.527 627.671 320.055 673.126 y
+488.856 703.526 500.056 707.526 v
+511.256 711.526 601.22 705.563 y
+596.056 725.417 L
+531.256 771.817 516.856 745.126 503.256 749.126 c
+489.656 753.127 492.056 743.526 488.856 742.726 c
+485.656 741.926 446.456 766.727 440.056 765.927 c
+f
+0.036 0.2 0.309 0 k
+441.365 764.181 m
+434.965 763.381 407.523 786.056 424.565 755.381 c
+446.565 715.781 366.164 716.181 349.364 727.381 c
+332.564 738.581 356.564 708.981 Y
+374.964 688.981 340.564 705.781 y
+306.164 718.581 282.164 692.981 278.964 692.181 c
+275.764 691.381 270.964 688.181 270.164 694.581 c
+269.364 700.981 262.773 716.508 230.164 691.381 c
+201.509 669.562 189.873 687.744 Y
+184.273 686.581 L
+169.872 714.981 178.382 659.017 Y
+187.182 624.616 321.364 671.381 y
+490.165 701.781 501.365 705.781 v
+512.565 709.781 601.656 703.89 y
+596.565 724.254 L
+531.765 770.654 518.165 743.381 504.565 747.381 c
+490.965 751.381 493.365 741.781 490.165 740.981 c
+486.965 740.181 447.765 764.981 441.365 764.181 c
+f
+0.027 0.15 0.231 0 k
+442.674 762.435 m
+436.274 761.635 408.832 784.311 425.874 753.635 c
+447.874 714.035 367.474 714.435 350.674 725.635 c
+333.874 736.835 357.874 707.235 Y
+376.274 687.235 341.874 704.035 y
+307.473 716.835 283.473 691.235 280.273 690.435 c
+277.073 689.635 272.273 686.435 271.473 692.835 c
+270.673 699.235 264.214 714.595 231.473 689.635 c
+201.582 667.071 190.255 684.707 Y
+184.255 685.035 L
+170.654 711.436 179.037 655.962 Y
+187.837 621.562 322.673 669.635 y
+491.474 700.035 502.674 704.035 v
+513.874 708.035 602.093 702.217 y
+597.075 723.09 L
+532.274 769.49 519.474 741.635 505.874 745.635 c
+492.274 749.635 494.674 740.035 491.474 739.235 c
+488.274 738.435 449.074 763.235 442.674 762.435 c
+f
+0.018 0.1 0.154 0 k
+443.983 760.69 m
+437.583 759.89 410.529 782.777 427.183 751.89 c
+449.183 711.09 368.783 712.69 351.983 723.89 c
+335.183 735.09 359.183 705.49 Y
+377.583 685.49 343.183 702.29 y
+308.783 715.09 284.783 689.49 281.583 688.69 c
+278.382 687.89 273.582 684.69 272.782 691.09 c
+271.982 697.49 265.654 712.682 232.782 687.89 c
+201.655 664.58 190.637 681.671 Y
+184.236 683.49 L
+171.236 707.49 179.691 652.907 Y
+188.491 618.507 323.983 667.89 y
+492.783 698.29 503.983 702.29 v
+515.183 706.29 602.529 700.544 y
+597.583 721.926 L
+532.783 768.327 520.783 739.89 507.183 743.89 c
+493.583 747.89 495.983 738.29 492.783 737.49 c
+489.583 736.69 450.383 761.49 443.983 760.69 c
+f
+0.009 0.05 0.077 0 k
+445.292 758.945 m
+438.892 758.145 412.917 781.589 428.492 750.145 c
+449.692 707.344 370.092 710.944 353.292 722.144 c
+336.492 733.344 360.492 703.744 Y
+378.892 683.744 344.492 700.544 y
+310.092 713.344 286.092 687.744 282.892 686.944 c
+279.692 686.144 274.892 682.944 274.092 689.344 c
+273.292 695.744 267.095 710.768 234.092 686.144 c
+201.727 662.089 191.018 678.635 Y
+184.218 681.944 L
+171.418 705.144 180.346 649.853 Y
+189.146 615.453 325.292 666.144 y
+494.093 696.544 505.293 700.544 v
+516.493 704.544 602.965 698.872 y
+598.093 720.763 L
+533.292 767.163 522.093 738.144 508.493 742.144 c
+494.893 746.145 497.293 736.544 494.093 735.744 c
+490.892 734.944 451.692 759.745 445.292 758.945 c
+f
+1 g
+184.2 680.399 m
+171.4 702.4 181 646.799 Y
+189.8 612.399 326.6 664.399 y
+495.401 694.8 506.601 698.8 v
+517.801 702.8 603.401 697.2 y
+598.601 719.6 L
+533.801 766 523.401 736.4 509.801 740.4 c
+496.201 744.4 498.601 734.8 495.401 734 c
+492.201 733.2 453.001 758 446.601 757.2 c
+440.201 756.4 414.981 780.207 429.801 748.4 c
+452.028 700.693 369.041 710.773 354.6 720.4 c
+337.8 731.6 361.8 702 Y
+380.2 681.999 345.8 698.8 y
+311.4 711.6 287.4 685.999 284.2 685.199 c
+281 684.399 276.2 681.199 275.4 687.599 c
+274.6 694 268.535 708.856 235.4 684.399 c
+201.8 659.599 191.4 675.599 Y
+184.2 680.399 L
+f
+0 g
+225.8 650.399 m
+218.6 638.799 239.4 625.599 V
+240.8 624.199 222.8 628.399 V
+216.6 630.399 215 640.799 V
+210.2 645.199 205.4 650.799 v
+200.6 656.399 225.8 650.399 y
+f
+0.8 g
+365.8 698 m
+383.498 671.179 382.9 666.399 v
+381.6 655.999 381.4 646.399 384.6 642.399 c
+387.801 638.399 396.601 605.199 y
+396.201 603.999 408.601 641.999 V
+420.201 657.999 400.201 676.399 V
+365 705.2 365.8 698 v
+f
+0 g
+1 J 0.1 w
+245.8 623.599 m
+257 616.399 242.6 585.199 V
+249 587.599 l
+248.2 576.399 245 573.999 V
+252.2 577.199 l
+257 569.199 253 564.399 V
+269.8 556.399 269 549.999 V
+275.4 557.999 271.4 564.399 v
+267.4 570.799 260.2 566.799 261 585.199 C
+252.2 581.999 l
+257.8 590.799 257.8 597.199 V
+249.8 594.799 l
+265.269 621.377 254.6 622.799 v
+248.6 623.599 245.8 623.599 Y
+f
+0.8 g
+278.2 606.799 m
+281 611.199 278.2 610.399 v
+275.4 609.599 244.2 594.799 238.2 585.199 C
+272.6 609.599 278.2 606.799 V
+f
+288.6 598.799 m
+291.4 603.199 288.6 602.399 v
+285.8 601.599 254.6 586.799 248.6 577.199 C
+283 601.599 288.6 598.799 V
+f
+301.8 613.999 m
+304.6 618.399 301.8 617.599 v
+299 616.799 267.8 601.999 261.8 592.399 C
+296.2 616.799 301.8 613.999 V
+f
+278.6 570.399 m
+278.6 576.399 275.8 575.599 v
+273 574.799 237 557.199 231 547.599 C
+273 573.199 278.6 570.399 V
+f
+279.8 581.199 m
+281 585.999 278.2 585.199 V
+276.2 585.199 249.8 573.599 243.8 563.999 C
+273.4 585.599 279.8 581.199 V
+f
+265.4 533.599 m
+255.4 525.999 l
+265.8 533.599 269.4 532.399 V
+262.6 521.199 261.8 515.999 V
+272.2 528.799 277.8 528.399 V
+285.4 527.999 285.4 517.199 V
+291 527.599 294.2 527.199 V
+295.4 520.799 294.2 513.999 V
+298.2 521.599 302.2 519.999 V
+308.6 521.999 307.8 510.399 V
+307.8 499.999 307 497.199 V
+312.6 523.599 315 523.999 V
+323 525.199 327.8 516.399 V
+323.8 523.999 328.6 521.999 V
+339.4 520.399 342.6 513.599 V
+335.8 525.599 341.4 522.399 V
+348.2 522.399 349.4 515.999 V
+357.8 494.799 359.8 493.199 V
+352.2 514.799 353.8 514.799 V
+351.8 526.799 357 511.999 V
+353.8 525.999 359.4 525.199 v
+365 524.399 369.4 514.399 377.8 516.799 C
+387.401 511.199 389.401 580.399 V
+265.4 533.599 L
+f
+0 g
+0 J 1 w
+270.2 626.399 m
+285 632.399 325 626.399 V
+332.2 625.999 339 634.799 v
+345.8 643.599 372.6 650.799 379 648.799 C
+388.601 642.399 l
+389.401 641.199 l
+401.801 630.799 402.201 623.199 v
+402.601 615.599 387.801 567.599 378.2 551.599 c
+368.6 535.599 359 523.199 339.8 525.599 C
+319 529.599 293.4 525.599 v
+264.2 527.199 261.4 535.199 v
+258.6 543.199 272.6 558.399 y
+277 566.799 275.8 581.199 v
+274.6 595.599 275 623.599 270.2 626.399 c
+f
+0.1 0.6 0.45 0 k
+292.2 624.399 m
+300.6 605.999 271 540.799 y
+269 539.199 283.66 533.154 293.8 535.599 c
+304.746 538.237 345 533.999 Y
+368.6 549.599 381.4 593.999 y
+391.801 617.999 374.2 621.199 v
+356.6 624.399 292.2 624.399 y
+f
+0.1 0.6 0.45 0.2 k
+290.169 593.503 m
+293.495 606.293 295.079 618.094 292.2 624.399 c
+354.6 617.999 365.8 638.799 v
+370.041 646.674 384.801 615.999 384.4 606.399 c
+321.4 591.999 306.6 603.199 V
+290.169 593.503 L
+f
+0.1 0.6 0.45 0.25 k
+294.6 577.199 m
+296.6 569.999 294.2 565.999 V
+292.6 565.199 291.4 564.799 V
+292.6 561.199 298.6 559.599 V
+300.6 555.199 303 554.799 v
+305.4 554.399 310.2 548.799 314.2 549.999 c
+318.2 551.199 329.4 555.199 y
+335 558.399 343.8 554.799 V
+346.175 555.601 346.6 559.599 v
+347.1 564.299 350.2 567.999 352.2 569.999 c
+354.2 571.999 363.8 584.799 362.6 585.199 c
+361.4 585.599 294.6 577.199 Y
+f
+0 0.55 0.5 0 k
+290.2 625.599 m
+287.4 603.199 290.6 594.799 v
+293.8 586.399 293 584.399 292.2 580.399 c
+291.4 576.399 295.8 566.399 301.4 560.399 C
+313.4 558.799 l
+328.6 562.399 337.8 559.599 V
+346.794 558.256 350.2 573.199 V
+355 579.599 362.2 582.399 v
+369.4 585.199 376.6 626.799 372.6 634.799 c
+368.6 642.799 354.2 647.199 338.2 631.599 c
+322.2 615.999 320.2 632.799 290.2 625.599 C
+b
+0 0 0.2 0 k
+0.5 w
+291.8 550.799 m
+291 552.799 286.6 553.199 V
+264.2 556.799 255.8 569.199 V
+249 574.799 253.4 563.199 V
+263.8 542.799 270.6 539.999 V
+287 535.999 291.8 550.799 V
+b
+0 0.55 0.5 0.2 k
+1 w
+371.742 614.771 m
+372.401 622.677 374.354 631.291 372.6 634.799 c
+366.154 647.693 349.181 642.305 338.2 631.599 c
+322.2 615.999 320.2 632.799 290.2 625.599 C
+288.455 611.636 289.295 601.624 v
+326.6 613.199 327.4 607.599 V
+329 610.799 338.2 610.799 v
+347.4 610.799 370.142 611.971 371.742 614.771 C
+f
+0 g
+0 0.55 0.5 0.35 K
+2 w
+328.6 624.799 m
+333.4 619.999 329.8 610.399 V
+315.4 594.399 317.4 580.399 v
+S
+0 0 0.2 0 k
+0 G
+0.5 w
+280.6 539.999 m
+276.2 552.799 285 545.999 V
+289.8 543.999 288.6 542.399 v
+287.4 540.799 281.8 536.799 280.6 539.999 C
+b
+285.64 538.799 m
+282.12 549.039 289.16 543.599 V
+293.581 541.151 292.04 540.719 v
+287.48 539.439 292.04 536.879 285.64 538.799 C
+b
+290.44 538.799 m
+286.92 549.039 293.96 543.599 V
+298.335 541.289 296.84 540.719 v
+293.48 539.439 296.84 536.879 290.44 538.799 C
+b
+297.04 538.599 m
+293.52 548.839 300.56 543.399 V
+304.943 541.067 303.441 540.519 v
+300.48 539.439 303.441 536.679 297.04 538.599 C
+b
+303.52 538.679 m
+300 548.919 307.041 543.479 V
+310.881 541.879 309.921 540.599 v
+308.961 539.319 309.921 536.759 303.52 538.679 C
+b
+310.2 537.999 m
+305.4 550.399 314.6 543.999 V
+319.4 541.999 318.2 540.399 v
+317 538.799 318.2 535.599 310.2 537.999 C
+b
+0 g
+0.1 0.6 0.45 0.25 K
+2 w
+281.8 555.199 m
+295 557.999 301 554.799 V
+307 553.599 308.2 553.999 v
+309.4 554.399 312.6 554.799 y
+S
+315.8 546.399 m
+327.8 559.999 339.8 555.599 v
+346.816 553.026 345.8 556.399 346.6 559.199 c
+347.4 561.999 347.6 566.199 352.6 569.199 c
+S
+0 0 0.2 0 k
+0 G
+0.5 w
+333 562.399 m
+329 573.199 326.2 560.399 v
+323.4 547.599 320.2 543.999 318.6 541.199 C
+318.6 535.999 327 536.399 V
+337.8 536.799 338.2 539.599 v
+338.6 542.399 337 553.999 333 562.399 C
+b
+0 g
+0.1 0.6 0.45 0.25 K
+2 w
+347 555.199 m
+350.6 557.599 353 556.399 v
+S
+353.5 571.599 m
+356.4 576.499 361.2 577.299 v
+S
+0.7 g
+0 G
+1 w
+274.2 534.799 m
+292.2 531.599 296.6 533.199 V
+305.4 533.199 297 531.199 V
+284.2 531.199 276.2 532.399 V
+264.6 537.999 274.2 534.799 V
+f
+0 0 0.2 0 k
+0.5 w
+288.2 627.999 m
+305.8 627.999 307.8 627.199 V
+315 596.399 311.4 588.799 V
+310.2 585.999 307.4 591.599 V
+289 624.399 285.8 626.399 v
+282.6 628.399 287 627.999 288.2 627.999 C
+b
+211.1 630.699 m
+220 628.999 232.6 626.399 V
+237.4 603.999 240.6 599.199 v
+243.8 594.399 240.2 594.399 236.6 597.199 c
+233 599.999 218.2 613.999 216.2 618.399 c
+214.2 622.799 211.1 630.699 y
+b
+232.961 626.182 m
+238.761 624.634 239.77 622.419 v
+240.778 620.205 238.568 616.908 y
+237.568 613.603 236.366 615.765 v
+235.164 617.928 232.292 625.588 232.961 626.182 c
+b
+0 g
+233 626.399 m
+236.6 621.199 240.2 621.199 v
+243.8 621.199 244.182 621.612 247 620.999 c
+251.6 619.999 251.2 621.999 257.8 620.799 c
+260.44 620.319 263 621.199 265.8 619.999 c
+268.6 618.799 271.8 619.599 273 621.599 c
+274.2 623.599 279 627.799 Y
+266.2 625.999 263.4 625.199 V
+241 623.999 233 626.399 V
+f
+0 0 0.2 0 k
+277.6 626.199 m
+271.15 622.699 270.75 620.299 v
+270.35 617.899 276 614.199 y
+278.75 609.599 279.35 611.999 v
+279.95 614.399 278.4 625.799 277.6 626.199 c
+b
+240.115 620.735 m
+247.122 609.547 247.339 620.758 V
+247.896 622.016 246.136 622.038 v
+240.061 622.114 241.582 626.216 240.115 620.735 C
+b
+247.293 620.486 m
+255.214 609.299 254.578 620.579 V
+254.585 620.911 252.832 621.064 v
+248.085 621.478 248.43 625.996 247.293 620.486 C
+b
+254.506 620.478 m
+262.466 609.85 261.797 619.516 V
+261.916 620.749 260.262 621.05 v
+256.37 621.756 256.159 625.005 254.506 620.478 C
+b
+261.382 620.398 m
+269.282 608.837 269.63 618.618 V
+271.274 619.996 269.528 620.218 v
+263.71 620.958 264.508 625.412 261.382 620.398 C
+b
+0 0 0.2 0.1 k
+225.208 616.868 m
+217.55 618.399 l
+214.95 623.399 212.85 629.549 y
+219.2 628.549 231.7 625.749 V
+232.576 622.431 234.048 616.636 v
+225.208 616.868 l
+f
+290.276 621.53 m
+288.61 624.036 287.293 625.794 286.643 626.2 c
+283.63 628.083 287.773 627.706 288.902 627.706 C
+305.473 627.706 307.356 626.953 V
+307.88 624.711 308.564 621.32 V
+298.476 623.33 290.276 621.53 V
+f
+0.2 0.55 0.85 0 k
+1 w
+343.88 759.679 m
+371.601 755.719 397.121 791.359 398.881 801.04 c
+400.641 810.72 390.521 822.6 Y
+391.841 825.68 387.001 839.76 381.721 849 c
+376.441 858.24 360.54 857.266 343 858.24 c
+327.16 859.12 308.68 835.8 307.36 834.04 c
+306.04 832.28 312.2 793.999 313.52 788.279 c
+314.84 782.559 312.2 756.159 y
+346.44 765.259 316.16 763.639 343.88 759.679 c
+f
+0.08 0.44 0.68 0 k
+308.088 833.392 m
+306.792 831.664 312.84 794.079 314.136 788.463 c
+315.432 782.847 312.84 756.927 y
+345.512 765.807 316.728 764.271 343.944 760.383 c
+371.161 756.495 396.217 791.487 397.945 800.992 c
+399.673 810.496 389.737 822.16 Y
+391.033 825.184 386.281 839.008 381.097 848.08 c
+375.913 857.152 360.302 856.195 343.08 857.152 c
+327.528 858.016 309.384 835.12 308.088 833.392 c
+f
+0.06 0.33 0.51 0 k
+308.816 832.744 m
+307.544 831.048 313.48 794.159 314.752 788.647 c
+316.024 783.135 313.48 757.695 y
+344.884 766.855 317.296 764.903 344.008 761.087 c
+370.721 757.271 395.313 791.615 397.009 800.944 c
+398.705 810.272 388.953 821.72 Y
+390.225 824.688 385.561 838.256 380.473 847.16 c
+375.385 856.064 360.063 855.125 343.16 856.064 c
+327.896 856.912 310.088 834.44 308.816 832.744 c
+f
+0.04 0.22 0.34 0 k
+309.544 832.096 m
+308.296 830.432 314.12 794.239 315.368 788.831 c
+316.616 783.423 314.12 758.463 y
+343.556 767.503 317.864 765.535 344.072 761.791 c
+370.281 758.047 394.409 791.743 396.073 800.895 c
+397.737 810.048 388.169 821.28 Y
+389.417 824.192 384.841 837.504 379.849 846.24 c
+374.857 854.976 359.824 854.055 343.24 854.976 c
+328.264 855.808 310.792 833.76 309.544 832.096 c
+f
+0.02 0.11 0.17 0 k
+310.272 831.448 m
+309.048 829.816 314.76 794.319 315.984 789.015 c
+317.208 783.711 314.76 759.231 y
+342.628 768.151 318.432 766.167 344.136 762.495 c
+369.841 758.823 393.505 791.871 395.137 800.848 c
+396.769 809.824 387.385 820.84 Y
+388.609 823.696 384.121 836.752 379.225 845.32 c
+374.329 853.888 359.585 852.985 343.32 853.888 c
+328.632 854.704 311.496 833.08 310.272 831.448 c
+f
+1 g
+344.2 763.2 m
+369.4 759.6 392.601 792 394.201 800.8 c
+395.801 809.6 386.601 820.4 Y
+387.801 823.2 383.4 836 378.6 844.4 c
+373.8 852.8 359.346 851.914 343.4 852.8 c
+329 853.6 312.2 832.4 311 830.8 c
+309.8 829.2 315.4 794.4 316.6 789.2 c
+317.8 784 315.4 760 y
+340.9 768.6 319 766.8 344.2 763.2 c
+f
+0.8 g
+390.601 797.2 m
+362.8 789.6 351.2 791.2 V
+335.4 797.8 326.6 776 V
+323 768.8 321 766.8 v
+319 764.8 390.601 797.2 Y
+f
+0 g
+394.401 799.4 m
+365.4 787.2 355.4 787.6 v
+339 792.2 330.6 777.6 V
+322.2 768.4 319 766.8 V
+318.6 765.2 325 769.2 V
+335.4 764 l
+350.2 754.4 359.8 770.4 V
+363.8 781.6 363.8 783.6 v
+363.8 785.6 385 791.2 386.601 791.6 c
+388.201 792 394.801 796.2 394.401 799.4 C
+f
+0.4 0.2 0.8 0 k
+347 763.486 m
+340.128 763.486 331.755 767.351 331.755 773.6 c
+331.755 779.848 340.128 786.113 347 786.113 c
+353.874 786.113 359.446 781.048 359.446 774.8 c
+359.446 768.551 353.874 763.486 347 763.486 c
+f
+0.4 0.2 0.8 0.2 k
+343.377 780.17 m
+338.531 779.448 333.442 777.945 333.514 778.161 c
+335.054 782.78 341.415 786.113 347 786.113 c
+351.296 786.113 355.084 784.135 357.32 781.125 c
+352.004 781.455 343.377 780.17 v
+f
+1 g
+355.4 780.4 m
+351 783.6 351 781.4 V
+354.6 777 355.4 780.4 V
+f
+0 g
+345.4 772.274 m
+342.901 772.274 340.875 774.3 340.875 776.8 c
+340.875 779.299 342.901 781.325 345.4 781.325 c
+347.9 781.325 349.926 779.299 349.926 776.8 c
+349.926 774.3 347.9 772.274 345.4 772.274 c
+f
+0.2 0.55 0.85 0 k
+241.4 785.6 m
+238.2 806.8 240.6 811.2 V
+251.4 821.2 251 824.8 V
+250.6 842.8 249.4 843.6 v
+248.2 844.4 240.6 850.4 234.6 844 C
+224.2 826 225 819.6 V
+225 817.6 l
+217.4 818 215.8 816 V
+214.6 810.8 213.4 810.4 V
+210.6 808 212.6 805.2 V
+210.6 802.8 211 798.8 V
+218.6 794.8 L
+220.6 780.4 231.4 775.2 v
+236.236 772.871 239.4 779.6 241.4 785.6 c
+f
+1 g
+240.4 787.44 m
+237.52 806.52 239.68 810.48 V
+249.4 819.48 249.04 822.72 V
+248.68 838.92 247.6 839.64 v
+246.52 840.36 239.68 845.76 234.28 840 C
+224.92 823.8 225.64 818.04 V
+225.64 816.24 l
+218.8 816.6 217.36 814.8 V
+216.28 810.12 215.2 809.76 V
+212.68 807.6 214.48 805.08 V
+212.68 802.92 213.04 799.32 V
+219.88 795.72 L
+221.68 782.76 231.4 778.08 v
+235.752 775.985 238.6 782.04 240.4 787.44 c
+f
+0.075 0.412 0.637 0 k
+248.95 842.61 m
+247.86 843.47 240.37 849.24 234.52 843 C
+224.38 825.45 225.16 819.21 V
+225.16 817.26 l
+217.75 817.65 216.19 815.7 V
+215.02 810.63 213.85 810.24 V
+211.12 807.9 213.07 805.17 V
+211.12 802.83 211.51 798.93 V
+218.92 795.03 L
+220.87 780.99 231.4 775.92 v
+236.114 773.65 239.2 780.21 241.15 786.06 c
+238.03 806.73 240.37 811.02 V
+250.9 820.77 250.51 824.28 V
+250.12 841.83 248.95 842.61 V
+f
+0.05 0.275 0.425 0 k
+248.5 841.62 m
+247.52 842.54 240.14 848.08 234.44 842 C
+224.56 824.9 225.32 818.82 V
+225.32 816.92 l
+218.1 817.3 216.58 815.4 V
+215.44 810.46 214.3 810.08 V
+211.64 807.8 213.54 805.14 V
+211.64 802.86 212.02 799.06 V
+219.24 795.26 L
+221.14 781.58 231.4 776.64 v
+235.994 774.428 239 780.82 240.9 786.52 c
+237.86 806.66 240.14 810.84 V
+250.4 820.34 250.02 823.76 V
+249.64 840.86 248.5 841.62 V
+f
+0.025 0.137 0.212 0 k
+248.05 840.63 m
+247.18 841.61 239.91 846.92 234.36 841 C
+224.74 824.35 225.48 818.43 V
+225.48 816.58 l
+218.45 816.95 216.97 815.1 V
+215.86 810.29 214.75 809.92 V
+212.16 807.7 214.01 805.11 V
+212.16 802.89 212.53 799.19 V
+219.56 795.49 L
+221.41 782.17 231.4 777.36 v
+235.873 775.206 238.8 781.43 240.65 786.98 c
+237.69 806.59 239.91 810.66 V
+249.9 819.91 249.53 823.24 V
+249.16 839.89 248.05 840.63 V
+f
+1 g
+240.4 787.54 m
+237.52 806.52 239.68 810.48 V
+249.4 819.48 249.04 822.72 V
+248.68 838.92 247.6 839.64 V
+246.84 840.68 239.68 845.76 234.28 840 C
+224.92 823.8 225.64 818.04 V
+225.64 816.24 l
+218.8 816.6 217.36 814.8 V
+216.28 810.12 215.2 809.76 V
+212.68 807.6 214.48 805.08 V
+212.68 802.92 213.04 799.32 V
+219.88 795.72 L
+221.68 782.76 231.4 778.08 v
+235.752 775.985 238.6 782.14 240.4 787.54 c
+f
+0.8 g
+237.3 793.8 m
+215.7 804 214.8 804.8 V
+223.9 796.6 224.7 796.6 v
+225.5 796.6 237.3 793.8 Y
+f
+0 g
+220.2 800 m
+238.6 796.4 238.6 792 v
+238.6 789.088 238.357 775.669 233 777.2 c
+224.6 779.6 228.2 794 220.2 800 c
+f
+0.4 0.2 0.8 0 k
+228.6 796.2 m
+237.578 794.726 238.6 792 v
+239.2 790.4 239.863 782.092 234.4 781 c
+229.848 780.089 227.618 790.31 228.6 796.2 c
+f
+0 g
+314.595 753.651 m
+314.098 755.393 315.409 755.262 317.2 755.8 c
+319.2 756.4 331.4 760.2 332.2 762.8 c
+333 765.4 346.2 761 Y
+348 760.2 352.4 757.6 Y
+357.2 756.4 363.8 756 Y
+366.2 755 369.6 752.2 Y
+384.2 742 396.601 749.2 Y
+416.601 755.8 410.601 773 Y
+407.601 782 410.801 785.4 Y
+411.001 789.2 418.201 782.8 Y
+420.801 778.6 421.601 773.6 Y
+429.601 762.4 426.201 780.2 Y
+426.401 781.2 423.601 784.8 423.601 786 c
+423.601 787.2 421.801 790.6 Y
+418.801 794 421.201 801 Y
+423.001 814.8 420.801 813 Y
+419.601 814.8 410.401 804.8 Y
+408.201 801.4 402.201 799.8 Y
+399.401 798 396.001 799.4 Y
+393.401 799.8 387.801 792.8 Y
+390.601 793 393.001 788.6 395.401 788.4 c
+397.801 788.2 399.601 790.8 401.201 791.4 c
+402.801 792 405.601 786.2 Y
+406.001 783.6 400.401 778.8 Y
+400.001 774.2 398.401 775.8 Y
+395.401 776.4 394.201 772.6 393.201 768 c
+392.201 763.4 388.001 763 y
+386.401 755.6 385.2 758.6 Y
+385 764.2 379 758.4 Y
+377.8 756.4 373.2 758.6 Y
+366.4 760.6 368.8 762.6 Y
+370.6 764.8 381.8 762.6 Y
+384 764.2 376 768.2 Y
+375.4 770 376.4 774.4 Y
+377.6 777.6 384.4 783.2 Y
+393.801 784.4 391.001 786 Y
+384.801 791.2 379 783.6 Y
+376.8 777.4 359.4 762.4 Y
+354.6 759 357.2 765.8 353.2 762.4 c
+349.2 759 328.6 768 y
+317.038 769.193 314.306 753.451 310.777 756.571 c
+316.195 748.051 314.595 753.651 v
+f
+509.401 920 m
+483.801 912 481.001 893.2 V
+478.601 870.4 499.001 852.8 V
+499.401 846.4 501.401 843.2 v
+499.801 838.4 518.601 846 V
+545.801 854.4 l
+552.201 856.8 557.401 865.6 v
+562.601 874.4 577.801 893.2 574.201 918.4 C
+575.401 929.6 569.401 930 V
+561.001 931.6 553.801 924 V
+547.001 920.8 544.601 921.2 V
+509.401 920 L
+f
+564.022 920.99 m
+566.122 929.92 561.282 925.08 V
+554.242 919.36 546.761 919.36 V
+532.241 917.16 527.841 903.96 V
+523.881 877.12 531.801 871.4 V
+536.641 863.92 543.681 870.52 v
+550.722 877.12 566.222 907.35 564.022 920.99 C
+f
+0.2 g
+563.648 920.632 m
+565.738 929.376 560.986 924.624 V
+554.074 919.008 546.729 919.008 V
+532.473 916.848 528.153 903.888 V
+524.265 877.536 532.041 871.92 V
+536.793 864.576 543.705 871.056 v
+550.618 877.536 565.808 907.24 563.648 920.632 C
+f
+0.4 g
+563.274 920.274 m
+565.354 928.832 560.69 924.168 V
+553.906 918.656 546.697 918.656 V
+532.705 916.536 528.465 903.816 V
+524.649 877.952 532.281 872.44 V
+536.945 865.232 543.729 871.592 v
+550.514 877.952 565.394 907.13 563.274 920.274 C
+f
+0.6 g
+562.9 919.916 m
+564.97 928.288 560.394 923.712 V
+553.738 918.304 546.665 918.304 V
+532.937 916.224 528.777 903.744 V
+525.033 878.368 532.521 872.96 V
+537.097 865.888 543.753 872.128 v
+550.41 878.368 564.98 907.02 562.9 919.916 C
+f
+0.8 g
+562.526 919.558 m
+564.586 927.744 560.098 923.256 V
+553.569 917.952 546.633 917.952 V
+533.169 915.912 529.089 903.672 V
+525.417 878.784 532.761 873.48 V
+537.249 866.544 543.777 872.664 v
+550.305 878.784 564.566 906.91 562.526 919.558 C
+f
+1 g
+562.151 919.2 m
+564.201 927.2 559.801 922.8 V
+553.401 917.6 546.601 917.6 V
+533.401 915.6 529.401 903.6 V
+525.801 879.2 533.001 874 V
+537.401 867.2 543.801 873.2 v
+550.201 879.2 564.151 906.8 562.151 919.2 C
+f
+0.1 0.55 0.85 0.3 k
+350.6 716 m
+330.2 735.2 322.2 736 V
+287.8 740 273 722 V
+290.6 742.4 318.2 736.8 V
+296.6 741.2 284.2 738 V
+267.4 738 257.8 724 V
+255 719.2 l
+259 734 277.4 740 V
+300.2 744.8 311 740 V
+289.4 746.8 279.4 744.8 V
+249 747.2 236.2 720.8 V
+240.2 735.2 255 742.4 V
+268.6 751.2 289 748.4 V
+303.4 745.2 308.6 742.8 v
+313.8 740.4 312.6 743.2 304.2 748 C
+298.6 758 284.6 757.6 V
+241.8 754 231.4 742 V
+245 753.2 255.4 756 V
+277.8 764 286.2 763.2 V
+311 762.2 318.6 766.2 V
+307.4 761.2 310.6 758 v
+313.8 754.8 320.6 747.2 320.6 746 c
+320.6 744.8 344.8 722.7 348.4 718.3 C
+350.6 716 l
+f
+0.8 g
+1 J 0.1 w
+489 522 m
+473.5 558.5 461 568 V
+487 552 490.5 534 V
+490.5 524 489 522 V
+f
+536 514.5 m
+509.5 569.5 491 593.5 V
+534.5 556 539.5 529.5 V
+540 524 l
+537 526.5 l
+536.5 517.5 536 514.5 V
+f
+592.5 563 m
+530 622.5 528.5 625 V
+589 559 592 551.5 V
+590 560.5 592.5 563 V
+f
+404 519.5 m
+423.5 571.5 442.5 549 V
+457.5 539 457 536 V
+453 542.5 435 542 V
+416 545 404 519.5 V
+f
+594.5 647 m
+549.5 675.5 542 677 v
+530.193 679.361 591.5 648 596.5 637.5 C
+598.5 640 594.5 647 V
+f
+0 g
+0 J 1 w
+443.801 540.399 m
+464.201 542.399 471.001 549.199 V
+475.401 545.599 l
+493.001 583.999 l
+496.601 578.799 l
+511.001 593.599 510.201 601.599 v
+509.401 609.599 523.001 595.599 y
+522.201 607.199 529.401 600.399 V
+527.001 615.999 535.401 607.999 V
+524.864 638.156 547.401 612.399 v
+553.001 605.999 548.601 612.799 y
+522.601 660.799 544.201 646.399 v
+546.201 669.199 545.001 673.599 v
+543.801 677.999 541.801 700.4 537.001 705.6 c
+532.201 710.8 537.401 712.4 543.001 707.2 C
+531.801 731.2 545.001 719.2 V
+541.401 734.4 537.001 737.2 V
+531.401 754.4 546.601 743.6 V
+542.201 756 539.001 759.2 V
+527.401 786.8 534.601 782 V
+539.001 778.4 l
+532.201 792.4 538.601 788 v
+545.001 783.6 545.001 784 y
+523.801 817.2 544.201 799.6 V
+536.042 813.518 532.601 820.4 V
+513.801 840.8 528.201 834.4 V
+533.001 832.8 l
+524.201 842.8 516.201 844.4 v
+508.201 846 518.601 852.4 525.001 850.4 c
+531.401 848.4 547.001 840.8 y
+559.801 822 563.801 821.6 V
+543.801 829.2 549.801 821.2 V
+564.201 807.2 557.001 807.6 V
+551.001 800.4 555.801 791.6 V
+537.342 809.991 552.201 784.4 v
+559.001 768 l
+534.601 792.8 545.801 770.8 V
+563.001 747.2 565.001 746.8 v
+567.001 746.4 571.401 737.6 y
+567.001 739.6 l
+572.201 730.8 l
+561.001 742.8 567.001 729.6 V
+572.601 715.2 l
+552.201 737.2 565.801 707.6 V
+549.401 712.8 558.201 695.6 V
+556.601 679.599 557.001 674.399 v
+557.401 669.199 558.601 640.799 554.201 632.799 c
+549.801 624.799 560.201 605.599 562.201 601.599 c
+564.201 597.599 567.801 586.799 559.001 595.999 c
+550.201 605.199 554.601 599.599 556.601 590.799 c
+558.601 581.999 564.601 566.399 563.801 560.799 C
+562.601 559.599 559.401 563.199 V
+544.601 585.999 546.201 571.599 V
+545.001 563.599 541.801 554.799 V
+538.601 543.999 538.601 552.799 V
+535.401 569.599 532.601 561.999 v
+529.801 554.399 526.201 548.399 523.401 545.999 c
+520.601 543.599 515.401 566.399 514.201 555.999 C
+502.201 568.399 497.401 551.999 V
+485.801 535.599 l
+485.401 547.999 484.201 541.999 V
+454.201 535.999 443.801 540.399 V
+f
+409.401 897.2 m
+397.801 905.2 393.801 904.8 v
+389.801 904.4 421.401 913.6 462.601 886 C
+467.401 883.2 471.001 883.6 V
+474.201 881.2 471.401 877.6 V
+462.601 868 473.801 856.8 V
+492.201 850 486.601 858.8 V
+497.401 854.8 499.801 850.8 v
+502.201 846.8 501.001 850.8 y
+494.601 858 488.601 863.2 V
+483.401 865.2 480.601 873.6 v
+477.801 882 475.401 892 479.801 895.2 C
+475.801 890.8 476.601 894.8 v
+477.401 898.8 481.001 902.4 482.601 902.8 c
+484.201 903.2 500.601 919 507.401 919.4 C
+498.201 918 495.201 919 v
+492.201 920 465.601 931.4 459.601 932.6 C
+442.801 939.2 454.801 937.2 V
+490.601 933.4 508.801 920.2 V
+501.601 928.6 483.201 935.6 V
+461.001 948.2 425.801 943.2 V
+408.001 940 400.201 938.2 V
+397.601 938.8 397.001 939.2 v
+396.401 939.6 384.6 948.6 357 941.6 C
+340 937 331.4 932.2 V
+316.2 931 312.6 927.8 V
+294 913.2 292 912.4 v
+290 911.6 278.6 904 277.8 903.6 C
+302.4 910.2 304.8 912.6 v
+307.2 915 324.6 917.6 327 916.2 c
+329.4 914.8 337.8 915.4 328.2 914.8 C
+403.801 900 404.601 898 v
+405.401 896 409.401 897.2 y
+f
+0.2 0.55 0.85 0 k
+480.801 906.4 m
+470.601 913.8 468.601 913.8 v
+466.601 913.8 454.201 924 450.001 923.6 c
+445.801 923.2 433.601 933.2 406.201 925 C
+405.601 927 409.201 927.8 V
+415.601 930 416.001 930.6 V
+436.201 934.8 443.401 931.2 V
+452.601 928.6 458.801 922.4 V
+470.001 919.2 473.201 920.2 V
+482.001 918 482.401 916.2 V
+488.201 913.2 486.401 910.6 V
+486.801 909 480.801 906.4 V
+f
+468.33 908.509 m
+469.137 907.877 470.156 907.779 470.761 906.97 c
+470.995 906.656 470.706 906.33 470.391 906.233 c
+469.348 905.916 468.292 906.486 467.15 905.898 c
+466.748 905.691 466.106 905.873 465.553 906.022 c
+463.921 906.463 462.092 906.488 460.401 905.8 C
+458.416 906.929 456.056 906.345 453.975 907.346 c
+453.917 907.373 453.695 907.027 453.621 907.054 c
+450.575 908.199 446.832 907.916 444.401 910.2 C
+441.973 910.612 439.616 911.074 437.188 911.754 c
+435.37 912.263 433.961 913.252 432.341 914.084 c
+430.964 914.792 429.507 915.314 427.973 915.686 c
+426.11 916.138 424.279 916.026 422.386 916.546 c
+422.293 916.571 422.101 916.227 422.019 916.254 c
+421.695 916.362 421.405 916.945 421.234 916.892 c
+419.553 916.37 418.065 917.342 416.401 917 C
+415.223 918.224 413.495 917.979 411.949 918.421 c
+408.985 919.269 405.831 917.999 402.801 919 C
+406.914 920.842 411.601 919.61 415.663 921.679 c
+417.991 922.865 420.653 921.763 423.223 922.523 c
+423.71 922.667 424.401 922.869 424.801 922.2 C
+424.935 922.335 425.117 922.574 425.175 922.546 c
+427.625 921.389 429.94 920.115 432.422 919.049 c
+432.763 918.903 433.295 919.135 433.547 918.933 c
+435.067 917.717 437.01 917.82 438.401 916.6 C
+440.099 917.102 441.892 916.722 443.621 917.346 c
+443.698 917.373 443.932 917.032 443.965 917.054 c
+445.095 917.802 446.25 917.531 447.142 917.227 c
+447.48 917.112 448.143 916.865 448.448 916.791 c
+449.574 916.515 450.43 916.035 451.609 915.852 c
+451.723 915.834 451.908 916.174 451.98 916.146 c
+453.103 915.708 454.145 915.764 454.801 914.6 C
+454.936 914.735 455.101 914.973 455.183 914.946 c
+456.21 914.608 456.859 913.853 457.96 913.612 c
+458.445 913.506 459.057 912.88 459.633 912.704 c
+462.025 911.973 463.868 910.444 466.062 909.549 c
+466.821 909.239 467.697 909.005 468.33 908.509 c
+f
+391.696 922.739 m
+389.178 924.464 386.81 925.57 384.368 927.356 c
+384.187 927.489 383.827 927.319 383.625 927.441 c
+382.618 928.05 381.73 928.631 380.748 929.327 c
+380.209 929.709 379.388 929.698 378.88 929.956 c
+376.336 931.248 373.707 931.806 371.2 933 C
+371.882 933.638 373.004 933.394 373.6 934.2 C
+373.795 933.92 374.033 933.636 374.386 933.827 c
+376.064 934.731 377.914 934.884 379.59 934.794 c
+381.294 934.702 383.014 934.397 384.789 934.125 c
+385.096 934.078 385.295 933.555 385.618 933.458 c
+387.846 932.795 390.235 933.32 392.354 932.482 c
+393.945 931.853 395.515 931.03 396.754 929.755 c
+397.006 929.495 396.681 929.194 396.401 929 C
+396.789 929.109 397.062 928.903 397.173 928.59 c
+397.257 928.351 397.257 928.049 397.173 927.81 c
+397.061 927.498 396.782 927.397 396.408 927.346 c
+395.001 927.156 396.773 928.536 396.073 928.088 c
+394.8 927.274 395.546 925.868 394.801 924.6 C
+394.521 924.794 394.291 925.012 394.401 925.4 C
+394.635 924.878 394.033 924.588 393.865 924.272 c
+393.48 923.547 392.581 922.132 391.696 922.739 c
+f
+359.198 915.391 m
+356.044 916.185 352.994 916.07 349.978 917.346 c
+349.911 917.374 349.688 917.027 349.624 917.054 c
+348.258 917.648 347.34 918.614 346.264 919.66 c
+345.351 920.548 343.693 920.161 342.419 920.648 c
+342.095 920.772 341.892 921.284 341.591 921.323 c
+340.372 921.48 339.445 922.429 338.4 923 C
+340.736 923.795 343.147 923.764 345.609 924.148 c
+345.722 924.166 345.867 923.845 346 923.845 c
+346.136 923.845 346.266 924.066 346.4 924.2 C
+346.595 923.92 346.897 923.594 347.154 923.848 c
+347.702 924.388 348.258 924.198 348.798 924.158 c
+348.942 924.148 349.067 923.845 349.2 923.845 c
+349.336 923.845 349.467 924.156 349.6 924.156 c
+349.736 924.155 349.867 923.845 350 923.845 c
+350.136 923.845 350.266 924.066 350.4 924.2 C
+351.092 923.418 351.977 923.972 352.799 923.793 c
+353.837 923.566 354.104 922.418 355.178 922.12 c
+359.893 920.816 364.03 918.671 368.393 916.584 c
+368.7 916.437 368.91 916.189 368.8 915.8 C
+369.067 915.8 369.38 915.888 369.57 915.756 c
+370.628 915.024 371.669 914.476 372.366 913.378 c
+372.582 913.039 372.253 912.632 372.02 912.684 c
+367.591 913.679 363.585 914.287 359.198 915.391 c
+f
+345.338 871.179 m
+343.746 872.398 343.162 874.429 342.034 876.221 c
+341.82 876.561 342.094 876.875 342.411 876.964 c
+342.971 877.123 343.514 876.645 343.923 876.443 c
+345.668 875.581 347.203 874.339 349.2 874.2 C
+351.19 871.966 355.45 871.581 355.457 868.2 c
+355.458 867.341 354.03 868.259 353.6 867.4 C
+351.149 868.403 348.76 868.3 346.38 869.767 c
+345.763 870.148 346.093 870.601 345.338 871.179 c
+f
+317.8 923.756 m
+317.935 923.755 324.966 923.522 324.949 923.408 c
+324.904 923.099 317.174 922.05 316.81 922.22 c
+316.646 922.296 309.134 919.866 309 920 C
+309.268 920.135 317.534 923.756 317.8 923.756 c
+f
+0 g
+333.2 914 m
+318.4 912.2 314 911 v
+309.6 909.8 291 902.2 288 900.2 C
+274.6 894.8 257.6 874.8 V
+265.2 878.2 267.4 881 V
+281 893.6 280.8 891 V
+293 899.6 292.4 897.4 V
+316.8 908.6 314.8 905.4 V
+336.4 910 335.4 908 V
+354.2 903.6 351.4 903.4 V
+345.6 902.2 352 898.6 V
+348.6 894.2 343.2 898.2 v
+337.8 902.2 340.8 900 335.8 899 C
+333.2 898.2 328.6 902.2 V
+323 906.8 314.2 903.2 V
+283.6 890.6 281.6 890 V
+278 887.2 275.6 883.6 V
+269.8 879.2 266.8 877.8 V
+254 866.2 252.8 864.8 V
+249.4 859.6 248.6 859.2 V
+255 863 257 865 V
+271 875 276.4 875.8 V
+280.8 878.8 281.6 880.2 V
+296 889.4 300.2 889.4 V
+309.4 884.2 311.8 891.2 V
+317.6 893 323.2 891.8 V
+326.4 894.4 325.6 896.6 V
+327.2 898.4 328.2 894.6 V
+331.6 891 336.4 893 V
+340.4 893.2 338.4 890.8 V
+334 887 322.2 886.8 V
+309.8 886.2 293.4 878.6 V
+263.6 868.2 254.4 857.8 V
+248 849 242.6 847.8 V
+236.8 847 230.8 839.6 V
+240.6 845.4 249.6 845.4 V
+253.6 847.8 249.8 844.2 V
+246.2 836.6 247.8 831.2 V
+247.2 826 246.4 824.4 V
+238.6 811.6 238.6 809.2 v
+238.6 806.8 239.8 797 240.2 796.4 c
+240.6 795.8 239.2 798 243 795.6 c
+246.8 793.2 249.6 791.6 250.4 788.8 c
+251.2 786 248.4 794.2 248.2 796 c
+248 797.8 243.8 805 244.6 807.4 C
+245.6 806.4 246.4 805 V
+245.8 805.6 246.4 809.2 V
+247.2 814.4 248.6 817.6 v
+250 820.8 252 824.6 252.4 825.4 c
+252.8 826.2 252.8 832 254.2 829.4 C
+257.6 826.8 l
+254.8 829.4 257 831.6 V
+256 837.2 257.8 839.8 V
+264.8 848.2 266.4 849.2 v
+268 850.2 266.6 849.8 y
+272.6 854 266.8 852.4 V
+262.8 850.8 259.8 850.8 V
+252.2 848.8 256.2 853 v
+260.2 857.2 270.2 862.6 274 862.4 C
+274.8 860.8 l
+286 863.2 l
+284.8 862.4 l
+284.6 862.6 288.8 863 v
+293 863.4 298.8 862 300.2 863.8 c
+301.6 865.6 305 866.6 304.6 865.2 c
+304.2 863.8 304 861.8 y
+309 867.6 308.4 865.4 v
+307.8 863.2 299.6 858 298.2 851.8 C
+308.6 860 l
+312.2 863 l
+315.8 860.8 316 862.4 v
+316.2 864 320.8 869.8 322 869.6 c
+323.2 869.4 325.2 872.2 325 869.6 c
+324.8 867 332.4 861.6 y
+335.6 863.4 337 862 v
+338.4 860.6 342.6 881.8 y
+367.6 892.4 l
+411.201 895.8 l
+394.201 902.6 l
+333.2 914 l
+f
+0.2 0.55 0.85 0.5 K
+1 J 2 w
+351.4 715 m
+336.4 731.8 328 734.4 V
+314.6 741.2 290 733.4 v
+S
+324.8 735.8 m
+299.6 743.8 284.2 739.6 V
+265.8 737.6 257.4 723.8 v
+S
+321.2 737 m
+304.2 744.2 289.4 746.4 V
+272.8 749 256.2 741.8 V
+244 735.8 238.6 725.6 v
+S
+322.2 736.6 m
+306.8 747.6 305.8 749 V
+298.8 760 285.8 760.4 V
+264.4 759.6 247.2 751.6 v
+S
+0 G
+0 J 1 w
+320.895 745.593 m
+322.437 744.13 349.4 715.2 Y
+384.6 678.599 356.6 712.8 Y
+349 717.6 339.8 736.4 Y
+338.6 739.2 353.8 729.2 Y
+357.8 728.4 371.4 709.2 Y
+364.6 711.6 369.4 704.4 Y
+372.2 702.4 392.601 686.799 Y
+396.201 682.799 400.201 681.199 Y
+414.201 686.399 407.801 673.199 Y
+410.201 666.399 415.801 677.999 Y
+427.001 694.8 410.601 692.399 Y
+380.6 689.599 373.8 705.6 Y
+371.4 708 380.2 705.6 Y
+388.601 703.6 373 718 Y
+375.4 718 384.6 711.2 Y
+395.001 702 397.001 704 Y
+415.001 712.8 425.401 705.2 Y
+427.401 703.6 421.801 696.8 423.401 691.599 c
+425.001 686.399 429.801 673.999 Y
+427.401 672.399 427.801 661.599 Y
+444.601 638.399 435.001 640.399 Y
+419.401 640.799 434.201 633.199 Y
+437.401 631.199 446.201 623.999 Y
+443.401 625.199 441.801 619.999 Y
+446.601 615.999 443.801 611.199 Y
+437.801 609.999 436.601 605.999 Y
+443.401 597.999 433.401 597.599 Y
+437.001 593.199 432.201 581.199 Y
+427.401 581.199 421.001 575.599 Y
+423.401 570.799 413.001 565.199 Y
+404.601 563.599 407.401 556.799 Y
+399.401 550.799 397.001 534.799 Y
+396.201 524.399 393.801 521.199 399.001 523.199 c
+404.201 525.199 403.401 537.599 Y
+398.601 553.199 441.401 569.199 Y
+445.401 570.799 446.201 575.999 Y
+448.201 575.599 457.001 567.999 Y
+464.601 556.799 465.001 565.999 Y
+466.201 569.599 464.601 575.599 Y
+470.601 597.199 456.601 603.599 Y
+446.601 637.199 460.601 628.799 Y
+463.401 623.199 474.201 617.999 y
+477.801 620.399 L
+476.201 625.199 484.601 631.199 Y
+487.401 624.799 493.401 632.799 Y
+497.001 657.199 509.401 642.799 Y
+513.401 641.599 514.601 648.399 Y
+518.201 658.799 514.601 672.399 Y
+518.201 672.799 527.801 666.799 Y
+530.601 670.399 521.401 687.199 525.401 684.799 c
+529.401 682.399 533.801 680.799 Y
+534.601 682.799 524.601 695.199 Y
+520.201 698 515.001 718.4 Y
+522.201 714.8 512.201 730 Y
+512.201 733.2 518.201 744.4 Y
+517.401 751.2 518.201 750.8 Y
+521.001 749.6 529.001 748 522.201 754.4 c
+515.401 760.8 523.001 765.6 Y
+527.401 768.4 513.801 768 Y
+508.601 772.4 509.001 776.4 Y
+517.001 774.4 502.601 788.8 500.201 792.4 c
+497.801 796 507.401 801.2 Y
+520.601 804.8 509.001 808 Y
+489.401 807.6 500.201 818.4 Y
+506.201 818 504.601 820.4 Y
+499.401 821.6 489.801 828 Y
+485.801 831.6 489.401 830.8 Y
+506.201 829.6 477.401 840.8 Y
+485.401 840.8 467.401 851.2 Y
+465.401 852.8 462.201 860.4 Y
+456.201 865.6 451.401 872.4 Y
+451.001 876.8 446.201 881.6 Y
+434.601 895.2 429.001 894.8 Y
+414.201 898.4 409.001 897.6 Y
+356.2 893.2 l
+329.8 880.4 337.6 859.4 Y
+344 851 353.2 854.8 Y
+357.8 861 369.4 858.8 Y
+389.801 855.6 387.201 859.2 Y
+384.801 863.8 368.6 870 368.4 870.6 c
+368.2 871.2 359.4 874.6 Y
+356.4 875.8 352 885 Y
+348.8 888.4 364.6 882.6 Y
+363.4 881.6 370.8 877.6 Y
+388.201 878.6 398.801 867.8 Y
+409.601 851.2 409.801 859.4 Y
+412.601 868.8 400.801 890 Y
+401.201 892 409.401 885.4 Y
+410.801 887.4 411.601 881.6 Y
+411.801 879.2 415.601 871.2 Y
+418.401 858.2 422.001 865.6 Y
+426.601 856.2 L
+428.001 853.6 422.001 846 Y
+421.801 843.2 422.601 843.4 417.001 835.8 c
+411.401 828.2 414.801 823.8 Y
+413.401 817.2 422.201 817.6 Y
+424.801 815.4 428.201 815.4 Y
+430.001 813.4 432.401 814 Y
+434.001 817.8 440.201 815.8 Y
+441.601 818.2 449.801 818.6 Y
+450.801 821.2 451.201 822.8 454.601 823.4 c
+458.001 824 433.401 867 Y
+439.801 867.8 431.601 880.2 Y
+429.401 886.8 440.801 872.2 443.001 870.8 c
+445.201 869.4 446.201 867.2 444.601 867.4 c
+443.001 867.6 441.201 865.4 442.601 865.2 c
+444.001 865 457.001 850 460.401 839.8 c
+463.801 829.6 469.801 825.6 476.001 819.6 c
+482.201 813.6 481.401 789.4 Y
+481.001 780.6 487.001 770 Y
+489.001 766.2 484.801 748 Y
+482.801 745.8 484.201 745 Y
+485.201 743.8 492.001 730.6 Y
+490.201 730.8 493.801 727.2 Y
+499.001 721.2 492.601 724.2 Y
+486.601 725.8 493.601 716 Y
+494.801 714.2 485.801 718.8 Y
+476.601 719.4 488.201 712.2 Y
+496.801 705 485.401 709.4 Y
+480.801 711.2 484.001 704.4 Y
+487.201 702.8 504.401 695.8 Y
+504.801 691.999 501.801 686.999 Y
+502.201 682.999 500.001 679.599 Y
+498.801 671.399 498.201 670.599 Y
+494.001 670.399 486.601 656.599 Y
+484.801 653.999 474.601 641.999 Y
+472.601 634.999 454.601 642.199 Y
+448.001 638.799 450.001 642.199 Y
+449.601 644.399 454.401 650.399 Y
+461.401 652.999 458.801 663.799 Y
+462.801 665.199 451.601 667.999 451.801 669.199 c
+452.001 670.399 457.801 671.799 Y
+465.801 673.799 461.401 676.199 Y
+460.801 680.199 463.801 685.799 Y
+475.401 686.599 463.801 702.8 Y
+453.001 710.4 452.001 716.2 Y
+464.601 724.4 456.401 736.8 456.601 740.4 c
+456.801 744 458.001 765.6 Y
+456.001 771.8 453.001 785.4 Y
+455.201 790.6 462.601 803.2 Y
+465.401 807.4 474.201 812.2 472.001 815.2 c
+469.801 818.2 462.001 816.4 Y
+454.201 817.8 454.801 812.6 Y
+453.201 811.6 452.401 806.6 Y
+451.68 798.667 442.801 792.4 Y
+431.601 786.2 440.801 782.2 Y
+446.801 775.6 437.001 775.4 Y
+426.001 777.2 434.201 767 Y
+445.001 754.2 442.001 751.4 Y
+431.801 750.4 444.401 741.2 y
+443.601 743.2 443.801 741.4 v
+444.001 739.6 447.001 735.4 447.801 733.4 c
+448.601 731.4 444.601 731.2 Y
+445.201 721.6 429.801 725.8 y
+429.801 725.8 428.201 725.6 v
+426.601 725.4 415.401 726.2 409.601 728.4 c
+403.801 730.6 397.001 730.6 y
+393.001 728.8 385.4 729 v
+377.8 729.2 369.8 726.4 Y
+365.4 726.8 374 731.2 374.2 731 c
+374.4 730.8 380 736.4 372 735.8 c
+350.203 734.165 339.4 744.4 Y
+337.4 745.8 334.8 748.6 Y
+324.8 750.6 336.2 736.2 Y
+337.4 734.8 336 733.8 Y
+335.2 735.4 327.4 740.8 Y
+324.589 741.773 323.226 743.107 320.895 745.593 C
+f
+0.2 0.55 0.85 0.5 k
+1 J 2 w
+297 757.2 m
+308.6 751.6 311.2 748.8 v
+313.8 746 327.8 734.6 y
+322.4 736.6 319.8 738.4 v
+317.2 740.2 306.4 748.4 y
+302.6 754.4 297 757.2 v
+f
+0.4 0.2 0.8 0 k
+0 J 1 w
+238.991 788.397 m
+239.328 788.545 238.804 791.257 238.6 791.8 c
+237.578 794.526 228.6 796 y
+228.373 794.635 228.318 793.039 228.424 791.401 c
+233.292 785.882 238.991 788.397 v
+f
+0.4 0.2 0.8 0.2 k
+238.991 788.597 m
+238.542 788.439 238.976 791.331 238.8 791.8 c
+237.778 794.526 228.6 796.1 y
+228.373 794.735 228.318 793.139 228.424 791.501 c
+232.692 786.382 238.991 788.597 v
+f
+0 g
+234.6 788.454 m
+233.975 788.454 233.469 789.594 233.469 791 c
+233.469 792.405 233.975 793.545 234.6 793.545 c
+235.225 793.545 235.732 792.405 235.732 791 c
+235.732 789.594 235.225 788.454 234.6 788.454 c
+f
+234.6 791 m
+F
+189 690.399 m
+183.4 680.399 208.2 686.399 V
+222.2 687.599 224.6 689.999 V
+225.8 689.199 234.166 686.266 237 685.599 c
+243.8 683.999 252.2 694 y
+256.8 704.5 259.6 704.5 v
+262.4 704.5 259.2 702.9 y
+252.6 692.799 253 691.199 V
+247.8 671.199 231.8 670.399 V
+215.65 669.449 217 663.599 V
+225.8 665.999 228.2 663.599 V
+239 663.999 231 657.599 V
+224.2 645.999 l
+224.34 642.081 214.2 645.599 v
+204.4 648.999 194.1 661.899 y
+178.15 676.449 189 690.399 V
+f
+0.1 0.4 0.4 0 k
+187.8 686.399 m
+185.8 676.799 222.6 687.199 V
+227 687.199 229.4 686.399 v
+231.8 685.599 243.8 682.799 245.8 683.999 C
+238.6 670.399 227 671.999 V
+213.8 670.399 214.2 665.599 V
+218.2 658.399 223 655.999 V
+225.8 653.599 225.4 650.399 v
+225 647.199 222.2 645.599 220.2 644.799 c
+218.2 643.999 215 647.199 213.4 647.199 c
+211.8 647.199 203.4 653.599 199 658.399 c
+194.6 663.199 186.2 675.199 186.6 677.999 c
+187 680.799 187.8 686.399 Y
+f
+0.1 0.4 0.4 0.2 k
+191 668.949 m
+193.6 664.999 196.8 660.799 199 658.399 c
+203.4 653.599 211.8 647.199 213.4 647.199 c
+215 647.199 218.2 643.999 220.2 644.799 c
+222.2 645.599 225 647.199 225.4 650.399 c
+225.8 653.599 223 655.999 Y
+219.934 657.532 217.194 661.024 215.615 663.347 C
+215.8 660.799 210.6 661.599 v
+205.4 662.399 200.2 665.199 198.6 668.399 c
+197 671.599 194.6 673.999 196.2 670.399 c
+197.8 666.799 200.2 663.199 201.8 662.799 c
+203.4 662.399 203 661.199 200.6 661.599 c
+198.2 661.999 195.4 662.399 191 667.599 c
+F
+0.1 0.55 0.85 0.3 k
+188.4 689.999 m
+190.2 703.6 191.4 707.6 V
+190.6 714.4 193 718.6 v
+195.4 722.8 197.4 729 200.4 734.4 c
+203.4 739.8 203.6 743.8 207.6 745.4 c
+211.6 747 217.6 755.6 220.4 756.6 c
+223.2 757.6 223 756.8 y
+229.8 771.6 243.4 767.6 V
+227.2 770.4 243 779.8 V
+238.2 778.7 241.5 785.7 v
+243.701 790.368 243.2 783.6 232.2 771.8 C
+227.2 763.2 222 760.2 v
+216.8 757.2 204.8 750.2 203.6 746.4 c
+202.4 742.6 199.2 736.8 197.2 735.2 c
+195.2 733.6 192.4 729.4 192 726 C
+190.8 722 189.4 720.8 v
+188 719.6 187.8 716.4 187.8 714.4 c
+187.8 712.4 185.8 709.6 186 707.2 C
+186.8 688.199 186.4 686.199 V
+188.4 689.999 L
+f
+1 g
+179.8 685.399 m
+177.8 686.799 173.4 680.799 V
+180.7 647.799 180.7 646.399 V
+181.8 648.499 180.5 655.699 v
+179.2 662.899 178.3 675.599 y
+179.8 685.399 l
+f
+0.1 0.55 0.85 0.3 k
+201.4 746 m
+183.8 742.8 184.2 713.6 V
+183.4 688.799 l
+182.2 714.4 181 716 v
+179.8 717.6 183.8 728.8 180.6 722.8 C
+166.6 708.8 174.6 687.599 V
+176.1 684.299 173.1 688.899 V
+168.5 701.5 169.6 707.9 V
+169.8 710.1 171.7 712.9 V
+180.3 724.6 183 726.9 V
+184.8 741.3 200.2 746.5 V
+205.9 748.8 201.4 746 V
+f
+0 g
+340.8 812.2 m
+341.46 812.554 341.451 813.524 342.031 813.697 c
+343.18 814.041 343.344 815.108 343.862 815.892 c
+344.735 817.211 344.928 818.744 345.51 820.235 c
+345.782 820.935 345.809 821.89 345.496 822.55 c
+344.322 825.031 343.62 827.48 342.178 829.906 c
+341.91 830.356 341.648 831.15 341.447 831.748 c
+340.984 833.132 339.727 834.123 338.867 835.443 c
+338.579 835.884 339.104 836.809 338.388 836.893 c
+337.491 836.998 336.042 837.578 335.809 836.552 c
+335.221 833.965 336.232 831.442 337.2 829 C
+336.418 828.308 336.752 827.387 336.904 826.62 c
+337.614 823.014 336.416 819.662 335.655 816.188 c
+335.632 816.084 335.974 815.886 335.946 815.824 c
+334.724 813.138 333.272 810.693 331.453 808.312 c
+330.695 807.32 329.823 806.404 329.326 805.341 c
+328.958 804.554 328.55 803.588 328.8 802.6 C
+325.365 799.82 323.115 795.975 320.504 792.129 c
+320.042 791.449 320.333 790.24 320.884 789.971 c
+321.697 789.573 322.653 790.597 323.123 791.443 c
+323.512 792.141 323.865 792.791 324.356 793.434 c
+324.489 793.609 324.31 794.028 324.445 794.149 c
+327.078 796.496 328.747 799.432 331.2 801.8 C
+333.15 802.129 334.687 803.127 336.435 804.14 c
+336.743 804.319 337.267 804.07 337.557 804.265 c
+339.31 805.442 339.308 807.478 339.414 809.388 c
+339.464 810.272 339.66 811.589 340.8 812.2 c
+f
+331.959 816.666 m
+332.083 816.743 331.928 817.166 332.037 817.382 c
+332.199 817.706 332.602 817.894 332.764 818.218 c
+332.873 818.434 332.71 818.814 332.846 818.956 c
+335.179 821.403 335.436 824.427 334.4 827.4 C
+335.424 828.02 335.485 829.282 335.06 830.129 c
+334.207 831.829 334.014 833.755 333.039 835.298 c
+332.237 836.567 330.659 837.811 329.288 836.508 c
+328.867 836.108 328.546 835.321 328.824 834.609 c
+328.888 834.446 329.173 834.3 329.146 834.218 c
+329.039 833.894 328.493 833.67 328.487 833.398 c
+328.457 831.902 327.503 830.391 328.133 829.062 c
+328.905 827.433 329.724 825.576 330.4 823.8 C
+329.166 821.684 330.199 819.235 328.446 817.358 c
+328.31 817.212 328.319 816.826 328.441 816.624 c
+328.733 816.138 329.139 815.732 329.625 815.44 c
+329.827 815.319 330.175 815.317 330.375 815.441 c
+330.953 815.803 331.351 816.29 331.959 816.666 c
+f
+394.771 826.977 m
+396.16 825.185 396.45 822.39 394.401 821 C
+394.951 817.691 398.302 819.67 400.401 820.2 C
+400.292 820.588 400.519 820.932 400.802 820.937 c
+401.859 820.952 402.539 821.984 403.601 821.8 C
+404.035 823.357 405.673 824.059 406.317 825.439 c
+408.043 829.134 407.452 833.407 404.868 836.653 c
+404.666 836.907 404.883 837.424 404.759 837.786 c
+404.003 839.997 401.935 840.312 400.001 841 C
+398.824 844.875 398.163 848.906 396.401 852.6 C
+394.787 852.85 394.089 854.589 392.752 855.309 c
+391.419 856.028 390.851 854.449 390.892 853.403 c
+390.899 853.198 391.351 852.974 391.181 852.609 c
+391.105 852.445 390.845 852.334 390.845 852.2 c
+390.846 852.065 391.067 851.934 391.201 851.8 C
+390.283 850.98 388.86 850.503 388.565 849.358 c
+387.611 845.648 390.184 842.523 391.852 839.322 c
+392.443 838.187 391.707 836.916 390.947 835.708 c
+390.509 835.013 390.617 833.886 390.893 833.03 c
+391.645 830.699 393.236 828.96 394.771 826.977 c
+f
+357.611 808.591 m
+356.124 806.74 352.712 804.171 355.629 802.243 c
+355.823 802.114 356.193 802.11 356.366 802.244 c
+358.387 803.809 360.39 804.712 362.826 805.294 c
+362.95 805.323 363.224 804.856 363.593 805.017 c
+365.206 805.72 367.216 805.662 368.4 807 C
+372.167 806.776 375.732 807.892 379.123 809.2 c
+380.284 809.648 381.554 810.207 382.755 810.709 c
+384.131 811.285 385.335 812.213 386.447 813.354 c
+386.58 813.49 386.934 813.4 387.201 813.4 C
+387.161 814.263 388.123 814.39 388.37 815.012 c
+388.462 815.244 388.312 815.64 388.445 815.742 c
+390.583 817.372 391.503 819.39 390.334 821.767 c
+390.049 822.345 389.8 822.963 389.234 823.439 c
+388.149 824.35 387.047 823.496 386 823.8 C
+385.841 823.172 385.112 823.344 384.726 823.146 c
+383.867 822.707 382.534 823.292 381.675 822.854 c
+380.313 822.159 379.072 821.99 377.65 821.613 c
+377.338 821.531 376.56 821.627 376.4 821 C
+376.266 821.134 376.118 821.368 376.012 821.346 c
+374.104 820.95 372.844 820.736 371.543 819.044 c
+371.44 818.911 370.998 819.09 370.839 818.955 c
+369.882 818.147 369.477 816.913 368.376 816.241 c
+368.175 816.118 367.823 816.286 367.629 816.157 c
+366.983 815.726 366.616 815.085 365.974 814.638 c
+365.645 814.409 365.245 814.734 365.277 814.99 c
+365.522 816.937 366.175 818.724 365.6 820.6 C
+367.677 823.12 370.194 825.069 372 827.8 C
+372.015 829.966 372.707 832.112 372.594 834.189 c
+372.584 834.382 372.296 835.115 372.17 835.462 c
+371.858 836.316 372.764 837.382 371.92 838.106 c
+370.516 839.309 369.224 838.433 368.4 837 C
+366.562 836.61 364.496 835.917 362.918 837.151 c
+361.911 837.938 361.333 838.844 360.534 839.9 c
+359.549 841.202 359.884 842.638 359.954 844.202 c
+359.96 844.33 359.645 844.466 359.645 844.6 c
+359.646 844.735 359.866 844.866 360 845 C
+359.294 845.626 359.019 846.684 358 847 C
+358.305 848.092 357.629 848.976 356.758 849.278 c
+354.763 849.969 353.086 848.057 351.194 847.984 c
+350.68 847.965 350.213 849.003 349.564 849.328 c
+349.132 849.544 348.428 849.577 348.066 849.311 c
+347.378 848.807 346.789 848.693 346.031 848.488 c
+344.414 848.052 343.136 846.958 341.656 846.103 c
+340.171 845.246 339.216 843.809 338.136 842.489 c
+337.195 841.337 337.059 838.923 338.479 838.423 c
+340.322 837.773 341.626 840.476 343.592 840.15 c
+343.904 840.099 344.11 839.788 344 839.4 C
+344.389 839.291 344.607 839.52 344.8 839.8 C
+345.658 838.781 346.822 838.444 347.76 837.571 c
+348.73 836.667 350.476 837.085 351.491 836.088 c
+353.02 834.586 352.461 831.905 354.4 830.6 C
+353.814 829.287 353.207 828.01 352.872 826.583 c
+352.59 825.377 353.584 824.18 354.795 824.271 c
+356.053 824.365 356.315 825.124 356.8 826.2 C
+357.067 825.933 357.536 825.636 357.495 825.42 c
+357.038 823.033 356.011 821.04 355.553 818.609 c
+355.494 818.292 355.189 818.09 354.8 818.2 C
+354.332 814.051 350.28 811.657 347.735 808.492 c
+347.332 807.99 347.328 806.741 347.737 806.338 c
+349.14 804.951 351.1 806.497 352.8 807 C
+353.013 808.206 353.872 809.148 355.204 809.092 c
+355.46 809.082 355.695 809.624 356.019 809.754 c
+356.367 809.892 356.869 809.668 357.155 809.866 c
+358.884 811.061 360.292 812.167 362.03 813.356 c
+362.222 813.487 362.566 813.328 362.782 813.436 c
+363.107 813.598 363.294 813.985 363.617 814.17 c
+363.965 814.37 364.207 814.08 364.4 813.8 C
+363.754 813.451 363.75 812.494 363.168 812.292 c
+362.393 812.024 361.832 811.511 361.158 811.064 c
+360.866 810.871 360.207 811.119 360.103 810.94 c
+359.505 809.912 358.321 809.474 357.611 808.591 c
+f
+302.2 858 m
+292.962 860.872 281.8 835.2 V
+279.4 830 277 828 v
+274.6 826 263.4 822.4 261.4 818.4 C
+251 802.4 L
+265.8 818.4 269 820.8 V
+277 829.2 273.8 822.4 V
+259.8 811.6 261 802.4 V
+255.4 788 254.6 786 V
+270.6 818 273 819.2 v
+275.4 820.4 276.6 820.4 275.4 816.8 c
+274.2 813.2 273.8 796.8 271 794.8 C
+279 815.2 278.2 818.4 V
+281.4 822 283.8 816.8 V
+282.6 800.8 l
+287 788.8 l
+284.6 800 286.2 815.6 V
+284.2 826 288.2 820.4 v
+292.2 814.8 301.8 808.8 301.8 804 C
+296.6 821.6 287.4 826.4 V
+283.4 820.4 l
+282.2 822.4 l
+278.6 823.2 283 830 v
+287.4 836.8 287 837.6 y
+293.4 830.4 295 830.4 V
+308.2 838 309.4 813.6 V
+316.2 828 307 834.8 V
+292.2 836.8 293.4 842 V
+300.6 854.4 L
+304.2 859.6 302.6 856.8 y
+F
+282.2 841.6 m
+269.4 841.6 266.2 836.4 V
+259 826.8 l
+276.2 836.8 280.2 838 v
+284.2 839.2 282.2 841.6 Y
+f
+242.2 835.2 m
+240.2 834 239.8 831.2 v
+239.4 828.4 237 828 237.8 825.2 c
+238.6 822.4 240.6 820 240.6 824 c
+240.6 828 242.2 830 243 831.2 c
+243.8 832.4 245.4 836.8 242.2 835.2 c
+f
+233.4 774 m
+225 778 221.8 781.6 v
+218.6 785.2 219.052 780.034 214.2 780.4 c
+208.353 780.841 209.4 796.8 y
+205.4 789.2 l
+204.2 774.8 212.2 777.2 v
+216.107 778.372 217.4 776.8 215.8 776 c
+214.2 775.2 221.4 774.8 218.6 773.2 c
+215.8 771.6 230.2 776.8 227.8 766.4 C
+233.4 774 L
+f
+220.8 759.6 m
+205.4 755.2 201.8 764.8 V
+197 762.4 199.2 759.4 v
+201.4 756.4 202.6 756 y
+208 754.8 207.4 754 v
+206.8 753.2 204.4 749.8 y
+214.6 755.8 220.8 759.6 v
+f
+1 g
+449.201 681.399 m
+448.774 679.265 447.103 678.464 445.201 677.799 C
+443.284 678.757 440.686 681.863 438.801 679.799 C
+438.327 680.279 437.548 680.339 437.204 681.001 c
+436.739 681.899 437.011 682.945 436.669 683.743 c
+436.124 685.015 435.415 686.381 435.601 687.799 C
+437.407 688.511 438.002 690.417 437.528 692.18 c
+437.459 692.437 437.03 692.634 437.23 692.983 c
+437.416 693.306 437.734 693.533 438.001 693.8 C
+437.866 693.665 437.721 693.432 437.61 693.452 c
+437 693.558 437.124 694.195 437.254 694.582 c
+437.839 696.328 439.853 696.592 441.201 695.4 C
+441.457 695.965 441.966 695.771 442.401 695.8 C
+442.351 696.379 442.759 696.906 442.957 697.326 c
+443.475 698.424 445.104 697.318 445.901 697.93 c
+446.977 698.755 448.04 699.454 449.118 698.851 c
+450.927 697.838 452.636 696.626 453.835 694.885 c
+454.41 694.051 454.65 692.77 454.592 691.812 c
+454.554 691.165 453.173 691.517 452.83 690.588 c
+452.185 688.84 454.016 688.321 454.772 686.983 c
+454.97 686.634 454.706 686.33 454.391 686.232 c
+453.98 686.104 453.196 686.293 453.334 685.84 c
+454.306 682.647 451.55 681.969 449.201 681.399 C
+f
+439.6 661.799 m
+439.593 663.537 437.992 665.293 439.201 666.999 C
+439.336 666.865 439.467 666.644 439.601 666.644 c
+439.736 666.644 439.867 666.865 440.001 666.999 C
+441.496 664.783 445.148 663.855 445.006 661.009 c
+444.984 660.562 443.897 659.644 444.801 658.999 C
+442.988 657.651 442.933 655.281 442.001 653.399 C
+440.763 653.685 439.551 654.048 438.401 654.599 C
+438.753 656.085 438.636 657.769 439.456 659.089 c
+439.89 659.787 439.603 660.866 439.6 661.799 c
+f
+0.8 g
+273.4 670.799 m
+256.542 660.663 270.6 675.999 v
+279.4 685.599 289.4 691.199 y
+299.8 695.6 303.4 696.8 v
+307 698 322.2 703.2 325.4 703.6 c
+328.6 704 338.2 708 345 704 c
+351.8 700 359.8 695.6 y
+343.4 704 339.8 701.6 v
+336.2 699.2 329 699.6 323 696.4 C
+308.2 691.999 305 689.999 v
+301.8 687.999 291.4 676.399 289.8 677.199 c
+288.2 677.999 290.2 678.399 291.4 681.199 c
+292.6 683.999 290.6 685.599 282.6 679.199 c
+274.6 672.799 273.4 670.799 Y
+f
+0 g
+280.805 676.766 m
+282.215 689.806 290.693 688.141 V
+298.919 692.311 301.641 694.279 V
+309.78 695.981 311.09 696.598 v
+329.569 705.298 344.288 700.779 344.835 701.899 c
+345.381 703.018 365.006 695.901 368.615 691.815 c
+369.006 691.372 358.384 697.412 348.686 699.303 c
+340.413 700.917 318.811 699.056 307.905 693.52 c
+304.932 692.011 295.987 686.227 293.456 686.338 c
+290.925 686.45 280.805 676.766 Y
+f
+0.8 g
+277 651.199 m
+261.8 653.599 278.6 655.199 V
+296.6 657.199 300.6 662.399 V
+314.2 671.599 317 671.999 v
+319.8 672.399 349.8 679.599 350.2 681.999 c
+350.6 684.399 356.2 684.399 357.8 683.599 c
+359.4 682.799 358.6 681.599 355.8 680.799 c
+353 679.999 321.8 663.599 315.4 662.399 c
+309 661.199 297.4 653.599 292.6 652.399 c
+287.8 651.199 277 651.199 Y
+f
+0 g
+296.52 658.597 m
+287.938 659.426 296.539 660.245 V
+305.355 663.669 307.403 666.332 V
+314.367 671.043 315.8 671.247 v
+317.234 671.452 331.194 675.139 331.399 676.367 c
+331.604 677.596 365.67 690.177 370.09 686.987 c
+373.001 684.886 363.1 686.563 353.466 682.153 c
+352.111 681.533 318.258 666.946 314.981 666.332 c
+311.704 665.717 305.765 661.826 303.307 661.212 c
+300.85 660.597 296.52 658.597 Y
+f
+288.6 656.399 m
+293.8 656.799 292.6 655.199 v
+291.4 653.599 289 654.399 y
+288.6 656.399 l
+f
+281.4 654.799 m
+286.6 655.199 285.4 653.599 v
+284.2 651.999 281.8 652.799 y
+281.4 654.799 l
+f
+271 653.199 m
+276.2 653.599 275 651.999 v
+273.8 650.399 271.4 651.199 y
+271 653.199 l
+f
+263.4 652.399 m
+268.6 652.799 267.4 651.199 v
+266.2 649.599 263.8 650.399 y
+263.4 652.399 l
+f
+301.8 691.999 m
+306.2 691.999 305 690.399 v
+303.8 688.799 300.6 689.199 y
+301.8 691.999 l
+f
+291.8 686.399 m
+298.306 688.54 295.8 685.199 v
+294.6 683.599 292.2 684.399 y
+291.8 686.399 l
+f
+280.6 681.599 m
+285.8 681.999 284.6 680.399 v
+283.4 678.799 281 679.599 y
+280.6 681.599 l
+f
+273 675.599 m
+278.2 675.999 277 674.399 v
+275.8 672.799 273.4 673.599 y
+273 675.599 l
+f
+266.2 670.799 m
+271.4 671.199 270.2 669.599 v
+269 667.999 266.6 668.799 y
+266.2 670.799 l
+f
+305.282 664.402 m
+312.203 664.934 310.606 662.805 v
+309.009 660.675 305.814 661.74 y
+305.282 664.402 l
+f
+315.682 669.202 m
+322.603 669.734 321.006 667.605 v
+319.409 665.475 316.214 666.54 y
+315.682 669.202 l
+f
+326.482 673.602 m
+333.403 674.134 331.806 672.005 v
+330.209 669.875 327.014 670.94 y
+326.482 673.602 l
+f
+336.882 678.402 m
+343.803 678.934 342.206 676.805 v
+340.609 674.675 337.414 675.74 y
+336.882 678.402 l
+f
+309.282 696.402 m
+316.203 696.934 314.606 694.805 v
+313.009 692.675 309.014 692.94 y
+309.282 696.402 l
+f
+319.282 699.602 m
+326.203 700.134 324.606 698.005 v
+323.009 695.875 318.614 696.14 y
+319.282 699.602 l
+f
+296.6 659.599 m
+301.8 659.999 300.6 658.399 v
+299.4 656.799 297 657.599 y
+296.6 659.599 l
+f
+0.1 0.55 0.85 0.3 k
+223.4 758.8 m
+219 750 218.6 746.8 V
+219.4 755.6 220.6 757.6 v
+221.8 759.6 223.4 758.8 y
+f
+205 744.8 m
+201.8 730.4 202.2 727.6 V
+201 739.2 201.4 740.4 v
+201.8 741.6 205 744.8 y
+f
+0.8 g
+225.8 819.4 m
+225.6 816.2 l
+223.4 816 l
+237.6 803.4 238.2 795.8 V
+239 804 225.8 819.4 V
+f
+0 g
+229.784 818.135 m
+229.353 818.551 229.572 819.296 229.164 819.556 c
+228.355 820.072 230.462 820.129 230.234 820.845 c
+229.851 822.051 230.038 822.072 229.916 823.348 c
+229.859 823.946 230.447 825.486 230.832 825.926 c
+232.278 827.578 230.954 830.51 232.594 832.061 c
+232.898 832.35 233.274 832.902 233.559 833.32 c
+234.218 834.283 235.402 834.771 236.352 835.599 c
+236.67 835.875 236.469 836.702 237.038 836.61 c
+237.752 836.495 238.993 836.625 238.948 835.784 c
+238.835 833.664 237.506 831.944 236.226 830.276 C
+236.677 829.572 236.219 828.937 235.935 828.38 c
+234.6 825.76 234.789 822.919 234.615 820.079 c
+234.61 819.994 234.303 819.916 234.311 819.863 c
+234.664 817.528 235.248 815.329 236.127 813.1 c
+236.493 812.17 236.964 811.275 237.114 810.348 c
+237.225 809.662 237.328 808.829 236.92 808.124 C
+238.955 805.234 237.646 802.583 238.815 799.052 c
+239.022 798.427 240.714 796.513 240.251 796.674 c
+237.738 797.545 237.626 797.943 237.449 798.696 c
+237.303 799.319 236.973 800.696 236.736 801.298 c
+236.672 801.462 236.501 803.346 236.423 803.468 c
+234.91 805.85 236.268 805.674 234.898 808.032 C
+233.47 808.712 232.504 809.816 231.381 810.978 c
+231.183 811.182 232.326 811.906 232.145 812.119 c
+231.053 813.408 229.9 814.175 230.236 815.668 c
+230.391 816.358 230.528 817.415 229.784 818.135 c
+f
+226.2 816.4 m
+226.6 809.6 229 808 v
+231.4 806.4 230.2 807.2 227 808.4 c
+223.8 809.6 225 810.4 y
+222.2 810 224.6 808 v
+227 806 230.6 803.6 229 803.6 c
+227.4 803.6 219.8 807.6 219.8 810.4 c
+219.8 813.2 218.8 817.3 y
+219.9 818.1 224.7 818 V
+226.1 817.3 226.2 816.4 V
+f
+1 g
+1 J 0.1 w
+225.4 797.8 m
+216.88 800.591 198.4 797.2 V
+207.431 799.278 226.2 797 v
+236.5 795.75 225.4 797.8 Y
+b
+227.498 797.871 m
+219.252 801.389 200.547 799.608 V
+209.725 800.897 228.226 797.005 v
+238.38 794.869 227.498 797.871 Y
+b
+229.286 797.778 m
+221.324 801.899 202.539 801.514 V
+211.787 802.118 229.948 796.86 v
+239.914 793.975 229.286 797.778 Y
+b
+230.556 797.555 m
+223.732 801.862 206.858 802.96 V
+215.197 802.79 231.078 796.681 v
+239.794 793.328 230.556 797.555 Y
+b
+345.84 787.039 m
+344.91 786.395 345.124 787.576 v
+345.339 788.757 373.547 801.927 377.161 801.677 C
+346.913 788.471 345.84 787.039 V
+b
+342.446 786.4 m
+341.57 785.685 341.691 786.879 v
+341.812 788.073 368.899 803.418 372.521 803.452 C
+343.404 787.911 342.446 786.4 V
+b
+339.16 785.025 m
+338.332 784.253 338.374 785.453 v
+338.416 786.652 358.233 802.149 368.045 804.023 C
+350.015 795.896 339.16 785.025 V
+b
+336.284 783.162 m
+335.539 782.468 335.577 783.547 v
+335.615 784.627 353.449 798.574 362.28 800.26 C
+346.054 792.946 336.284 783.162 V
+b
+0.8 g
+0 J 1 w
+304.6 635.199 m
+289.4 637.599 306.2 639.199 V
+324.2 641.199 328.2 646.399 V
+341.8 655.599 344.6 655.999 v
+347.4 656.399 363.8 659.999 364.2 662.399 c
+364.6 664.799 370.6 667.199 372.2 666.399 c
+373.8 665.599 373.8 656.399 371 655.599 c
+368.2 654.799 349.4 647.599 343 646.399 c
+336.6 645.199 325 637.599 320.2 636.399 c
+315.4 635.199 304.6 635.199 Y
+f
+0 g
+377.6 672.599 m
+374.6 670.999 373.4 668.399 V
+367 657.799 352.8 654.599 V
+329.8 645.599 322 643.599 V
+308.6 638.599 301.2 639.399 V
+294.2 639.199 300.4 637.599 V
+320.6 639.599 324 641.399 V
+339.6 646.599 342.6 649.199 v
+345.6 651.799 363.8 656.799 366 658.799 c
+368.2 660.799 378 669.199 377.6 672.599 C
+f
+318.882 641.089 m
+324.111 641.315 322.958 639.766 v
+321.805 638.216 319.357 639.09 y
+318.882 641.089 l
+f
+311.68 639.737 m
+316.908 639.963 315.756 638.414 v
+314.603 636.864 312.155 637.737 y
+311.68 639.737 l
+f
+301.251 638.489 m
+306.48 638.716 305.327 637.166 v
+304.174 635.617 301.726 636.49 y
+301.251 638.489 l
+f
+293.617 637.945 m
+298.846 638.171 297.693 636.622 v
+296.54 635.072 294.092 635.946 y
+293.617 637.945 l
+f
+335.415 648.487 m
+342.375 648.788 340.84 646.726 v
+339.306 644.664 336.047 645.826 y
+335.415 648.487 l
+f
+345.73 652.912 m
+351.689 656.213 351.155 651.151 v
+350.885 648.595 346.362 650.251 y
+345.73 652.912 l
+f
+354.862 655.726 m
+362.021 659.427 360.287 653.965 v
+359.509 651.515 355.493 653.065 y
+354.862 655.726 l
+f
+364.376 660.551 m
+368.735 665.452 369.801 658.79 v
+370.207 656.252 365.008 657.89 y
+364.376 660.551 l
+f
+326.834 644.003 m
+332.062 644.23 330.91 642.68 v
+329.757 641.131 327.308 642.004 y
+326.834 644.003 l
+f
+1 g
+1 J 0.1 w
+362.434 765.397 m
+361.708 764.732 361.707 765.803 v
+361.707 766.873 379.191 780.137 388.034 781.521 C
+371.935 774.792 362.434 765.397 V
+b
+0 g
+0 J 1 w
+365.4 701.6 m
+387.401 679.199 396.601 675.599 V
+405.801 664.399 401.801 638.399 V
+398.601 630.799 395.401 651.599 V
+398.601 676.799 387.401 660.799 V
+379 670.699 385.4 670.399 V
+388.601 668.399 389.001 669.999 v
+389.401 671.599 381.4 685.199 364.2 699.6 c
+347 714 365.4 701.6 Y
+f
+1 g
+1 J 0.1 w
+307 662.799 m
+306.8 664.599 308.6 663.799 v
+310.4 662.999 404.601 656.799 436.201 632.799 C
+391.001 655.999 307 662.799 V
+b
+317.4 667.199 m
+317.2 668.999 319 668.199 v
+320.8 667.399 457.401 668.399 481.001 635.999 C
+459.001 661.199 317.4 667.199 V
+b
+329 671.199 m
+328.8 672.999 330.6 672.199 v
+332.4 671.399 505.801 684.399 529.401 651.999 C
+519.801 677.599 329 671.199 V
+b
+339 675.999 m
+338.8 677.799 340.6 676.999 v
+342.4 676.199 464.601 714.8 488.201 682.399 C
+474.801 707 339 675.999 V
+b
+281 653.199 m
+280.8 654.999 282.6 654.199 v
+284.4 653.399 302.2 651.199 304.2 612.399 C
+297 654.399 281 653.199 V
+b
+272.2 651.599 m
+272 653.399 273.8 652.599 v
+275.6 651.799 289.8 656.399 287 617.599 C
+288.2 652.799 272.2 651.599 V
+b
+264.2 651.199 m
+264 652.999 265.8 652.199 v
+267.6 651.399 283 650.799 270.6 628.399 C
+280.2 652.399 264.2 651.199 V
+b
+311.526 695.535 m
+311.082 693.536 312.631 694.753 v
+328.699 707.378 361.141 766.28 416.826 771.914 C
+378.518 784.024 311.526 695.535 V
+b
+322.726 697.335 m
+321.363 698.528 323.231 699.153 v
+325.099 699.778 437.541 772.28 476.826 764.314 C
+449.719 771.824 322.726 697.335 V
+b
+301.885 691.233 m
+301.376 689.634 303.087 690.61 v
+312.062 695.73 315.677 752.941 359.254 754.196 C
+326.843 768.91 301.885 691.233 V
+b
+281.962 680.207 m
+280.885 678.921 282.838 679.175 v
+293.084 680.507 314.489 721.778 358.928 716.699 C
+326.962 731.045 281.962 680.207 V
+b
+293.2 686.333 m
+292.389 684.864 294.258 685.489 v
+304.057 688.763 317.141 733.375 361.729 736.922 C
+327.603 744.865 293.2 686.333 V
+b
+274.922 675.088 m
+274.049 674.046 275.631 674.252 v
+283.93 675.331 301.268 708.76 337.264 704.646 C
+311.371 716.266 274.922 675.088 V
+b
+267.323 669.179 m
+266.318 668.134 267.909 668.252 v
+272.077 668.561 302.715 701.64 321.183 686.138 C
+309.168 704.861 267.323 669.179 V
+b
+336.855 701.102 m
+335.654 702.457 337.586 702.842 v
+339.518 703.226 460.221 760.939 498.184 748.073 C
+472.243 758.947 336.855 701.102 V
+b
+303.4 636.799 m
+303.2 638.599 305 637.799 v
+306.8 636.999 322.2 636.399 309.8 613.999 C
+319.4 637.999 303.4 636.799 V
+b
+313.8 638.399 m
+313.6 640.199 315.4 639.399 v
+317.2 638.599 335 636.399 337 597.599 C
+329.8 639.599 313.8 638.399 V
+b
+320.6 639.999 m
+320.4 641.799 322.2 640.999 v
+324 640.199 348.6 636.799 372.2 604.399 C
+336.6 641.199 320.6 639.999 V
+b
+328.225 642.028 m
+327.788 643.786 329.678 643.232 v
+331.568 642.678 352.002 644.577 390.099 610.401 C
+343.924 645.344 328.225 642.028 V
+b
+338.625 646.428 m
+338.188 648.186 340.078 647.632 v
+341.968 647.078 376.802 642.577 428.499 607.601 C
+354.324 649.744 338.625 646.428 V
+b
+298.2 657.999 m
+298 659.799 299.8 658.999 v
+301.6 658.199 355 655.599 385.4 628.799 C
+350.499 653.574 298.2 657.999 V
+b
+288.2 653.999 m
+288 655.799 289.8 654.999 v
+291.6 654.199 316.2 650.799 339.8 618.399 C
+304.2 655.199 288.2 653.999 V
+b
+349.503 651.038 m
+348.938 652.759 350.864 652.345 v
+352.79 651.932 387.86 649.996 441.981 618.902 C
+364.317 653.296 349.503 651.038 V
+b
+357.903 653.438 m
+357.338 655.159 359.264 654.745 v
+361.19 654.332 396.26 652.396 450.381 621.302 C
+373.317 656.096 357.903 653.438 V
+b
+367.503 658.438 m
+366.938 660.159 368.864 659.745 v
+370.79 659.332 413.86 654.996 503.582 620.702 C
+382.917 661.096 367.503 658.438 V
+b
+0 g
+0 J 1 w
+256.2 651.599 m
+261.4 651.999 260.2 650.399 v
+259 648.799 256.6 649.599 y
+256.2 651.599 l
+f
+287 637.599 m
+292.2 637.999 291 636.399 v
+289.8 634.799 287.4 635.599 y
+287 637.599 l
+f
+278.2 637.999 m
+283.4 638.399 282.2 636.799 v
+281 635.199 278.6 635.999 y
+278.2 637.999 l
+f
+182.831 649.818 m
+187.876 648.495 186.218 647.376 v
+184.561 646.256 182.554 647.798 y
+182.831 649.818 l
+f
+184.831 659.418 m
+189.876 658.095 188.218 656.976 v
+186.561 655.856 184.554 657.398 y
+184.831 659.418 l
+f
+177.631 663.818 m
+182.676 662.495 181.018 661.376 v
+179.361 660.256 177.354 661.798 y
+177.631 663.818 l
+f
+0.8 g
+1 J 0.1 w
+257.4 588.799 m
+255.8 588.799 251.8 586.799 V
+249.8 586.799 238.6 583.199 233 573.199 C
+245.4 582.799 257.4 588.799 V
+f
+345.116 496.153 m
+345.257 495.895 345.312 495.475 345.604 495.458 c
+346.262 495.418 347.495 495.117 347.37 495.753 c
+346.522 500.059 345.648 504.996 341.515 506.803 c
+340.876 507.082 339.434 506.669 339.36 505.785 c
+339.233 504.261 339.116 502.912 339.425 501.446 c
+339.725 500.025 341.883 500.015 342.8 501.399 C
+343.736 499.727 344.168 497.884 345.116 496.153 c
+f
+334.038 491.419 m
+334.786 490.006 334.659 488.147 336.074 487.584 c
+336.814 487.29 338.664 488.265 338.246 489.339 c
+337.444 491.4 337.056 493.639 335.667 495.45 c
+335.467 495.712 335.707 496.245 335.547 496.573 c
+334.953 497.793 333.808 498.528 332.4 498.199 C
+331.285 495.996 332.433 493.867 333.955 492.158 c
+334.091 492.006 333.925 491.63 334.038 491.419 c
+f
+294.436 496.609 m
+294.328 496.986 294.29 497.449 294.455 497.77 c
+294.986 498.803 295.779 499.925 295.442 500.947 c
+295.094 502.003 293.978 501.821 293.328 501.252 c
+292.193 500.258 292.144 498.432 291.453 497.073 c
+291.257 496.687 291.308 496.114 290.867 495.723 c
+290.393 495.302 289.953 493.778 290.049 493.207 c
+290.102 492.894 289.919 482.986 290.141 483.249 c
+290.76 483.982 293.81 493.716 293.879 494.608 c
+293.936 495.339 294.668 495.804 294.436 496.609 c
+f
+268.798 503.401 m
+271.432 505.9 274.222 508.861 273.78 512.573 c
+273.664 513.549 271.889 513.022 271.702 512.176 c
+270.9 508.551 268.861 505.89 266.293 503.498 c
+264.097 501.451 262.235 495.107 262 494.599 C
+265.697 499.855 267.954 502.601 268.798 503.401 c
+f
+255.224 509.365 m
+255.747 509.735 255.445 510.226 255.662 510.558 c
+256.615 512.016 257.916 513.262 257.934 515 c
+257.937 515.277 257.559 515.586 257.224 515.362 c
+256.947 515.178 256.605 515.048 256.497 514.918 c
+254.467 512.469 253.067 509.798 251.624 506.986 c
+251.441 506.629 250.297 502.138 250.61 502.027 c
+250.849 501.942 252.569 506.123 252.779 506.237 c
+254.042 506.923 254.054 508.538 255.224 509.365 c
+f
+271.957 489.821 m
+272.401 490.69 273.977 491.892 273.864 492.781 c
+273.746 493.709 274.214 495.152 273.302 494.464 c
+272.045 493.516 268.596 492.167 268.326 486.359 c
+268.3 485.788 271.274 488.481 271.957 489.821 c
+f
+286.4 506.999 m
+286.8 507.667 287.508 507.194 287.967 507.457 c
+288.615 507.829 289.226 508.387 289.518 509.036 c
+290.488 511.185 292.257 513.005 292.4 515.399 C
+290.909 516.804 290.23 514.764 289.6 513.799 C
+288.277 515.446 287.278 513.572 285.978 513.053 c
+285.908 513.025 285.695 513.372 285.62 513.345 c
+284.443 512.905 283.763 511.824 282.765 511.043 c
+282.594 510.909 282.189 511.089 282.042 510.953 c
+281.39 510.35 280.417 510.025 280.137 509.343 c
+279.027 506.636 275.887 504.541 274 496.999 C
+274.381 496.09 278.512 503.641 278.999 504.339 c
+279.835 505.535 279.953 502.678 281.229 503.344 c
+281.28 503.371 281.466 503.133 281.6 502.999 C
+281.794 503.279 282.012 503.508 282.4 503.399 C
+282.4 503.799 282.266 504.355 282.467 504.514 c
+283.704 505.491 283.62 506.559 284.4 507.799 C
+284.858 507.01 285.919 507.729 286.4 506.999 C
+f
+346.2 452.599 m
+353.6 472.999 349.2 484.199 V
+360.6 462.599 356 451.399 V
+355.6 461.799 351.6 466.799 V
+347.6 453.999 346.2 452.599 V
+f
+331.4 455.199 m
+336.8 463.999 328.8 482.399 V
+328 461.999 321.2 450.999 V
+335.4 471.199 331.4 455.199 V
+f
+321.4 457.199 m
+321.2 477.199 321.6 480.199 V
+317.8 463.599 307.6 453.999 V
+322 465.999 321.4 457.199 V
+f
+311.8 489.199 m
+317.8 475.599 307.8 457.199 V
+314.2 469.399 309.4 476.399 V
+312 479.799 311.8 489.199 V
+f
+292.6 457.599 m
+291.6 473.199 293.4 475.399 V
+293.6 481.799 293.2 482.799 V
+297.2 488.999 297.4 481.599 V
+298.8 473.799 301.6 469.199 V
+305.2 463.799 305 457.399 V
+295 487.599 292.6 457.599 V
+f
+289 485.199 m
+282.4 474.399 280.6 455.399 V
+279.2 461.599 283 475.999 V
+287.2 491.399 289 485.199 V
+f
+267.2 465.399 m
+272.2 470.799 273.6 475.799 V
+277.2 491.599 270.8 482.999 V
+271 474.999 262.8 467.599 V
+267.6 469.999 267.2 465.399 V
+f
+261.4 470.399 m
+264.8 487.799 265.6 488.599 V
+267.4 491.999 264.6 488.799 V
+255.8 469.599 251.8 462.999 V
+259.8 472.199 261.4 470.399 V
+f
+255.6 486.999 m
+267.2 509.399 245.4 483.599 V
+256.4 493.399 255.6 486.999 V
+f
+240.2 501.599 m
+245 520.399 247.6 520.199 V
+255.8 529.199 249.2 518.599 V
+243.2 508.999 243.8 499.199 V
+243.2 508.799 240.2 501.599 V
+f
+570.5 513 m
+558.5 523 556 526.5 V
+569.5 508 569.5 501 V
+572 508.5 570.5 513 V
+f
+576 535 m
+555 550 551.5 557.5 V
+578 528 578 523.5 V
+578.5 532.5 576 535 V
+f
+593 689 m
+581 697 579.5 695 V
+590 688.5 592.5 680 V
+591 689 593 689 V
+f
+601.5 608.5 m
+584 620.5 l
+603 603.5 603.5 599.5 V
+601.5 608.5 L
+f
+0 g
+1 w
+210.75 631 m
+232.75 626.25 l
+S
+261 469 m
+260.5 472.5 251.5 462 v
+S
+266.5 464 m
+268.5 470.5 262 466 v
+S
+320.5 455.5 m
+322 466.5 310.5 453.5 v
+S
+
+showpage
+
+%%Trailer
diff --git a/samples/README.md b/samples/README.md
new file mode 100644
index 0000000..939c475
--- /dev/null
+++ b/samples/README.md
@@ -0,0 +1,88 @@
+draw2d samples
+==============
+
+Various samples for using draw2d
+
+Using the image backend
+-----------------------
+
+The following Go code draws the android sample on a png image:
+
+```
+import (
+ "image"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/samples/android"
+)
+
+function main(){}
+ // Initialize the graphic context on an RGBA image
+ dest := image.NewRGBA(image.Rect(0, 0, 297, 210.0))
+ gc := draw2d.NewGraphicContext(dest)
+ // Draw Android logo
+ fn, err := android.Main(gc, "png")
+ if err != nil {
+ t.Errorf("Drawing %q failed: %v", fn, err)
+ return
+ }
+ // Save to png
+ err = draw2d.SaveToPngFile(fn, dest)
+ if err != nil {
+ t.Errorf("Saving %q failed: %v", fn, err)
+ }
+}
+```
+
+Using the pdf backend
+---------------------
+
+The following Go code draws the android sample on a pdf document:
+
+```
+import (
+ "image"
+
+ "github.com/llgcode/draw2d/draw2dpdf"
+ "github.com/llgcode/draw2d/samples/android"
+)
+
+function main(){}
+ // Initialize the graphic context on a pdf document
+ dest := draw2dpdf.NewPdf("L", "mm", "A4")
+ gc := draw2dpdf.NewGraphicContext(dest)
+ // Draw Android logo
+ fn, err := android.Main(gc, "png")
+ if err != nil {
+ t.Errorf("Drawing %q failed: %v", fn, err)
+ return
+ }
+ // Save to pdf
+ err = draw2dpdf.SaveToPdfFile(fn, dest)
+ if err != nil {
+ t.Errorf("Saving %q failed: %v", fn, err)
+ }
+}
+```
+
+Testing
+-------
+
+These samples are run as tests from the root package folder `draw2d` by:
+```
+go test ./...
+```
+Or if you want to run with test coverage:
+```
+go test -cover ./... | grep -v "no test"
+```
+The following files are responsible to run the image tests:
+```
+draw2d/test_test.go
+draw2d/samples_test.go
+```
+The following files are responsible to run the pdf tests:
+```
+draw2d/pdf/test_test.go
+draw2dpdf/samples_test.go
+```
diff --git a/samples/android/android.go b/samples/android/android.go
new file mode 100644
index 0000000..3d96c88
--- /dev/null
+++ b/samples/android/android.go
@@ -0,0 +1,76 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff
+
+// Package android draws an android avatar.
+package android
+
+import (
+ "image/color"
+ "math"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws a droid and returns the filename. This should only be
+// used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ // Draw the droid
+ Draw(gc, 65, 0)
+
+ // Return the output filename
+ return samples.Output("android", ext), nil
+}
+
+// Draw the droid on a certain position.
+func Draw(gc draw2d.GraphicContext, x, y float64) {
+ // set the fill and stroke color of the droid
+ gc.SetFillColor(color.RGBA{0x44, 0xff, 0x44, 0xff})
+ gc.SetStrokeColor(color.RGBA{0x44, 0x44, 0x44, 0xff})
+
+ // set line properties
+ gc.SetLineCap(draw2d.RoundCap)
+ gc.SetLineWidth(5)
+
+ // head
+ gc.MoveTo(x+30, y+70)
+ gc.ArcTo(x+80, y+70, 50, 50, 180*(math.Pi/180), 180*(math.Pi/180))
+ gc.Close()
+ gc.FillStroke()
+ gc.MoveTo(x+60, y+25)
+ gc.LineTo(x+50, y+10)
+ gc.MoveTo(x+100, y+25)
+ gc.LineTo(x+110, y+10)
+ gc.Stroke()
+
+ // left eye
+ draw2dkit.Circle(gc, x+60, y+45, 5)
+ gc.FillStroke()
+
+ // right eye
+ draw2dkit.Circle(gc, x+100, y+45, 5)
+ gc.FillStroke()
+
+ // body
+ draw2dkit.RoundedRectangle(gc, x+30, y+75, x+30+100, y+75+90, 10, 10)
+ gc.FillStroke()
+ draw2dkit.Rectangle(gc, x+30, y+75, x+30+100, y+75+80)
+ gc.FillStroke()
+
+ // left arm
+ draw2dkit.RoundedRectangle(gc, x+5, y+80, x+5+20, y+80+70, 10, 10)
+ gc.FillStroke()
+
+ // right arm
+ draw2dkit.RoundedRectangle(gc, x+135, y+80, x+135+20, y+80+70, 10, 10)
+ gc.FillStroke()
+
+ // left leg
+ draw2dkit.RoundedRectangle(gc, x+50, y+150, x+50+20, y+150+50, 10, 10)
+ gc.FillStroke()
+
+ // right leg
+ draw2dkit.RoundedRectangle(gc, x+90, y+150, x+90+20, y+150+50, 10, 10)
+ gc.FillStroke()
+}
diff --git a/samples/appengine/app.yaml b/samples/appengine/app.yaml
new file mode 100644
index 0000000..361cfca
--- /dev/null
+++ b/samples/appengine/app.yaml
@@ -0,0 +1,8 @@
+application: draw2d-test
+version: 1
+runtime: go
+api_version: go1
+
+handlers:
+- url: /.*
+ script: _go_app
\ No newline at end of file
diff --git a/samples/appengine/server.go b/samples/appengine/server.go
new file mode 100644
index 0000000..bbfa584
--- /dev/null
+++ b/samples/appengine/server.go
@@ -0,0 +1,73 @@
+// +build appengine
+
+// Package gae demonstrates draw2d on a Google appengine server.
+package gae
+
+import (
+ "fmt"
+ "image"
+ "image/png"
+ "net/http"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dpdf"
+ "github.com/llgcode/draw2d/samples/android"
+
+ "appengine"
+)
+
+type appError struct {
+ Error error
+ Message string
+ Code int
+}
+
+type appHandler func(http.ResponseWriter, *http.Request) *appError
+
+func (fn appHandler) ServeHTTP(w http.ResponseWriter, r *http.Request) {
+ if e := fn(w, r); e != nil { // e is *appError, not os.Error.
+ c := appengine.NewContext(r)
+ c.Errorf("%v", e.Error)
+ http.Error(w, e.Message, e.Code)
+ }
+}
+
+func init() {
+ http.Handle("/pdf", appHandler(pdf))
+ http.Handle("/png", appHandler(imgPng))
+}
+
+func pdf(w http.ResponseWriter, r *http.Request) *appError {
+ w.Header().Set("Content-type", "application/pdf")
+
+ // Initialize the graphic context on an pdf document
+ dest := draw2dpdf.NewPdf("L", "mm", "A4")
+ gc := draw2dpdf.NewGraphicContext(dest)
+
+ // Draw sample
+ android.Draw(gc, 65, 0)
+
+ err := dest.Output(w)
+ if err != nil {
+ return &appError{err, fmt.Sprintf("Can't write: %s", err), 500}
+ }
+ return nil
+}
+
+func imgPng(w http.ResponseWriter, r *http.Request) *appError {
+ w.Header().Set("Content-type", "image/png")
+
+ // Initialize the graphic context on an RGBA image
+ dest := image.NewRGBA(image.Rect(0, 0, 297, 210.0))
+ gc := draw2d.NewGraphicContext(dest)
+
+ // Draw sample
+ android.Draw(gc, 65, 0)
+
+ err := png.Encode(w, dest)
+ if err != nil {
+ return &appError{err, fmt.Sprintf("Can't encode: %s", err), 500}
+ }
+
+ return nil
+}
diff --git a/samples/frameimage/frameimage.go b/samples/frameimage/frameimage.go
new file mode 100644
index 0000000..b584db0
--- /dev/null
+++ b/samples/frameimage/frameimage.go
@@ -0,0 +1,60 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff, Stani Michiels
+
+// Package frameimage centers a png image and rotates it.
+package frameimage
+
+import (
+ "math"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dimg"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws the image frame and returns the filename.
+// This should only be used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ // Margin between the image and the frame
+ const margin = 30
+ // Line width od the frame
+ const lineWidth = 3
+
+ // Gopher image
+ gopher := samples.Resource("image", "gopher.png", ext)
+
+ // Draw gopher
+ err := Draw(gc, gopher, 297, 210, margin, lineWidth)
+
+ // Return the output filename
+ return samples.Output("frameimage", ext), err
+}
+
+// Draw the image frame with certain parameters.
+func Draw(gc draw2d.GraphicContext, png string,
+ dw, dh, margin, lineWidth float64) error {
+ // Draw frame
+ draw2dkit.RoundedRectangle(gc, lineWidth, lineWidth, dw-lineWidth, dh-lineWidth, 100, 100)
+ gc.SetLineWidth(lineWidth)
+ gc.FillStroke()
+
+ // load the source image
+ source, err := draw2dimg.LoadFromPngFile(png)
+ if err != nil {
+ return err
+ }
+ // Size of source image
+ sw, sh := float64(source.Bounds().Dx()), float64(source.Bounds().Dy())
+ // Draw image to fit in the frame
+ // TODO Seems to have a transform bug here on draw image
+ scale := math.Min((dw-margin*2)/sw, (dh-margin*2)/sh)
+ gc.Save()
+ gc.Translate((dw-sw*scale)/2, (dh-sh*scale)/2)
+ gc.Scale(scale, scale)
+ gc.Rotate(0.2)
+
+ gc.DrawImage(source)
+ gc.Restore()
+ return nil
+}
diff --git a/samples/geometry/geometry.go b/samples/geometry/geometry.go
new file mode 100644
index 0000000..99f1731
--- /dev/null
+++ b/samples/geometry/geometry.go
@@ -0,0 +1,334 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff
+
+// Package geometry draws some geometric tests.
+package geometry
+
+import (
+ "image"
+ "image/color"
+ "math"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+ "github.com/llgcode/draw2d/samples/gopher2"
+)
+
+// Main draws geometry and returns the filename. This should only be
+// used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ // Draw the droid
+ Draw(gc, 297, 210)
+
+ // Return the output filename
+ return samples.Output("geometry", ext), nil
+}
+
+// Bubble draws a text balloon.
+func Bubble(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/100, height/100
+ gc.MoveTo(x+sx*50, y)
+ gc.QuadCurveTo(x, y, x, y+sy*37.5)
+ gc.QuadCurveTo(x, y+sy*75, x+sx*25, y+sy*75)
+ gc.QuadCurveTo(x+sx*25, y+sy*95, x+sx*5, y+sy*100)
+ gc.QuadCurveTo(x+sx*35, y+sy*95, x+sx*40, y+sy*75)
+ gc.QuadCurveTo(x+sx*100, y+sy*75, x+sx*100, y+sy*37.5)
+ gc.QuadCurveTo(x+sx*100, y, x+sx*50, y)
+ gc.Stroke()
+}
+
+// CurveRectangle draws a rectangle with bezier curves (not rounded rectangle).
+func CurveRectangle(gc draw2d.GraphicContext, x0, y0,
+ rectWidth, rectHeight float64, stroke, fill color.Color) {
+ radius := (rectWidth + rectHeight) / 4
+
+ x1 := x0 + rectWidth
+ y1 := y0 + rectHeight
+ if rectWidth/2 < radius {
+ if rectHeight/2 < radius {
+ gc.MoveTo(x0, (y0+y1)/2)
+ gc.CubicCurveTo(x0, y0, x0, y0, (x0+x1)/2, y0)
+ gc.CubicCurveTo(x1, y0, x1, y0, x1, (y0+y1)/2)
+ gc.CubicCurveTo(x1, y1, x1, y1, (x1+x0)/2, y1)
+ gc.CubicCurveTo(x0, y1, x0, y1, x0, (y0+y1)/2)
+ } else {
+ gc.MoveTo(x0, y0+radius)
+ gc.CubicCurveTo(x0, y0, x0, y0, (x0+x1)/2, y0)
+ gc.CubicCurveTo(x1, y0, x1, y0, x1, y0+radius)
+ gc.LineTo(x1, y1-radius)
+ gc.CubicCurveTo(x1, y1, x1, y1, (x1+x0)/2, y1)
+ gc.CubicCurveTo(x0, y1, x0, y1, x0, y1-radius)
+ }
+ } else {
+ if rectHeight/2 < radius {
+ gc.MoveTo(x0, (y0+y1)/2)
+ gc.CubicCurveTo(x0, y0, x0, y0, x0+radius, y0)
+ gc.LineTo(x1-radius, y0)
+ gc.CubicCurveTo(x1, y0, x1, y0, x1, (y0+y1)/2)
+ gc.CubicCurveTo(x1, y1, x1, y1, x1-radius, y1)
+ gc.LineTo(x0+radius, y1)
+ gc.CubicCurveTo(x0, y1, x0, y1, x0, (y0+y1)/2)
+ } else {
+ gc.MoveTo(x0, y0+radius)
+ gc.CubicCurveTo(x0, y0, x0, y0, x0+radius, y0)
+ gc.LineTo(x1-radius, y0)
+ gc.CubicCurveTo(x1, y0, x1, y0, x1, y0+radius)
+ gc.LineTo(x1, y1-radius)
+ gc.CubicCurveTo(x1, y1, x1, y1, x1-radius, y1)
+ gc.LineTo(x0+radius, y1)
+ gc.CubicCurveTo(x0, y1, x0, y1, x0, y1-radius)
+ }
+ }
+ gc.Close()
+ gc.SetStrokeColor(stroke)
+ gc.SetFillColor(fill)
+ gc.SetLineWidth(10.0)
+ gc.FillStroke()
+}
+
+// Dash draws a line with a dash pattern
+func Dash(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/162, height/205
+ gc.SetStrokeColor(image.Black)
+ gc.SetLineDash([]float64{height / 10, height / 50, height / 50, height / 50}, -50.0)
+ gc.SetLineCap(draw2d.ButtCap)
+ gc.SetLineJoin(draw2d.RoundJoin)
+ gc.SetLineWidth(height / 50)
+
+ gc.MoveTo(x+sx*60.0, y)
+ gc.LineTo(x+sx*60.0, y)
+ gc.LineTo(x+sx*162, y+sy*205)
+ rLineTo(gc, sx*-102.4, 0)
+ gc.CubicCurveTo(x+sx*-17, y+sy*205, x+sx*-17, y+sy*103, x+sx*60.0, y+sy*103.0)
+ gc.Stroke()
+ gc.SetLineDash(nil, 0.0)
+}
+
+// Arc draws an arc with a positive angle (clockwise)
+func Arc(gc draw2d.GraphicContext, xc, yc, width, height float64) {
+ // draw an arc
+ xc += width / 2
+ yc += height / 2
+ radiusX, radiusY := width/2, height/2
+ startAngle := 45 * (math.Pi / 180.0) /* angles are specified */
+ angle := 135 * (math.Pi / 180.0) /* clockwise in radians */
+ gc.SetLineWidth(width / 10)
+ gc.SetLineCap(draw2d.ButtCap)
+ gc.SetStrokeColor(image.Black)
+ gc.MoveTo(xc+math.Cos(startAngle)*radiusX, yc+math.Sin(startAngle)*radiusY)
+ gc.ArcTo(xc, yc, radiusX, radiusY, startAngle, angle)
+ gc.Stroke()
+
+ // fill a circle
+ gc.SetStrokeColor(color.NRGBA{255, 0x33, 0x33, 0x80})
+ gc.SetFillColor(color.NRGBA{255, 0x33, 0x33, 0x80})
+ gc.SetLineWidth(width / 20)
+
+ gc.MoveTo(xc+math.Cos(startAngle)*radiusX, yc+math.Sin(startAngle)*radiusY)
+ gc.LineTo(xc, yc)
+ gc.LineTo(xc-radiusX, yc)
+ gc.Stroke()
+
+ gc.MoveTo(xc, yc)
+ gc.ArcTo(xc, yc, width/10.0, height/10.0, 0, 2*math.Pi)
+ gc.Fill()
+}
+
+// ArcNegative draws an arc with a negative angle (anti clockwise).
+func ArcNegative(gc draw2d.GraphicContext, xc, yc, width, height float64) {
+ xc += width / 2
+ yc += height / 2
+ radiusX, radiusY := width/2, height/2
+ startAngle := 45.0 * (math.Pi / 180.0) /* angles are specified */
+ angle := -225 * (math.Pi / 180.0) /* clockwise in radians */
+ gc.SetLineWidth(width / 10)
+ gc.SetLineCap(draw2d.ButtCap)
+ gc.SetStrokeColor(image.Black)
+
+ gc.ArcTo(xc, yc, radiusX, radiusY, startAngle, angle)
+ gc.Stroke()
+ // fill a circle
+ gc.SetStrokeColor(color.NRGBA{255, 0x33, 0x33, 0x80})
+ gc.SetFillColor(color.NRGBA{255, 0x33, 0x33, 0x80})
+ gc.SetLineWidth(width / 20)
+
+ gc.MoveTo(xc+math.Cos(startAngle)*radiusX, yc+math.Sin(startAngle)*radiusY)
+ gc.LineTo(xc, yc)
+ gc.LineTo(xc-radiusX, yc)
+ gc.Stroke()
+
+ gc.ArcTo(xc, yc, width/10.0, height/10.0, 0, 2*math.Pi)
+ gc.Fill()
+}
+
+// CubicCurve draws a cubic curve with its control points.
+func CubicCurve(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/162, height/205
+ x0, y0 := x, y+sy*100.0
+ x1, y1 := x+sx*75, y+sy*205
+ x2, y2 := x+sx*125, y
+ x3, y3 := x+sx*205, y+sy*100
+
+ gc.SetStrokeColor(image.Black)
+ gc.SetFillColor(color.NRGBA{0xAA, 0xAA, 0xAA, 0xFF})
+ gc.SetLineWidth(width / 10)
+ gc.MoveTo(x0, y0)
+ gc.CubicCurveTo(x1, y1, x2, y2, x3, y3)
+ gc.Stroke()
+
+ gc.SetStrokeColor(color.NRGBA{0xFF, 0x33, 0x33, 0x88})
+
+ gc.SetLineWidth(width / 20)
+ // draw segment of curve
+ gc.MoveTo(x0, y0)
+ gc.LineTo(x1, y1)
+ gc.LineTo(x2, y2)
+ gc.LineTo(x3, y3)
+ gc.Stroke()
+}
+
+// FillString draws a filled and stroked string.
+func FillString(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/100, height/100
+ gc.Save()
+ gc.SetStrokeColor(image.Black)
+ gc.SetLineWidth(1)
+ draw2dkit.RoundedRectangle(gc, x+sx*5, y+sy*5, x+sx*95, y+sy*95, sx*10, sy*10)
+ gc.FillStroke()
+ gc.SetFillColor(image.Black)
+ gc.SetFontSize(height / 6)
+ gc.Translate(x+sx*6, y+sy*52)
+ gc.SetFontData(draw2d.FontData{
+ Name: "luxi",
+ Family: draw2d.FontFamilyMono,
+ Style: draw2d.FontStyleBold | draw2d.FontStyleItalic})
+ w := gc.FillString("Hug")
+ gc.Translate(w+sx, 0)
+ left, top, right, bottom := gc.GetStringBounds("cou")
+ gc.SetStrokeColor(color.NRGBA{255, 0x33, 0x33, 0x80})
+ draw2dkit.Rectangle(gc, left, top, right, bottom)
+ gc.SetLineWidth(height / 50)
+ gc.Stroke()
+ gc.SetFillColor(color.NRGBA{0x33, 0x33, 0xff, 0xff})
+ gc.SetStrokeColor(color.NRGBA{0x33, 0x33, 0xff, 0xff})
+ gc.SetLineWidth(height / 100)
+ gc.StrokeString("Hug")
+ gc.Restore()
+}
+
+// FillStroke first fills and afterwards strokes a path.
+func FillStroke(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/210, height/215
+ gc.MoveTo(x+sx*113.0, y)
+ gc.LineTo(x+sx*215.0, y+sy*215)
+ rLineTo(gc, sx*-100, 0)
+ gc.CubicCurveTo(x+sx*35, y+sy*215, x+sx*35, y+sy*113, x+sx*113.0, y+sy*113)
+ gc.Close()
+
+ gc.MoveTo(x+sx*50.0, y)
+ rLineTo(gc, sx*51.2, sy*51.2)
+ rLineTo(gc, sx*-51.2, sy*51.2)
+ rLineTo(gc, sx*-51.2, sy*-51.2)
+ gc.Close()
+
+ gc.SetLineWidth(width / 20.0)
+ gc.SetFillColor(color.NRGBA{0, 0, 0xFF, 0xFF})
+ gc.SetStrokeColor(image.Black)
+ gc.FillStroke()
+}
+
+func rLineTo(path draw2d.PathBuilder, x, y float64) {
+ x0, y0 := path.LastPoint()
+ path.LineTo(x0+x, y0+y)
+}
+
+// FillStyle demonstrates the difference between even odd and non zero winding rule.
+func FillStyle(gc draw2d.GraphicContext, x, y, width, height float64) {
+ sx, sy := width/232, height/220
+ gc.SetLineWidth(width / 40)
+
+ draw2dkit.Rectangle(gc, x+sx*0, y+sy*12, x+sx*232, y+sy*70)
+
+ var wheel1, wheel2 draw2d.Path
+ wheel1.ArcTo(x+sx*52, y+sy*70, sx*40, sy*40, 0, 2*math.Pi)
+ wheel2.ArcTo(x+sx*180, y+sy*70, sx*40, sy*40, 0, -2*math.Pi)
+
+ gc.SetFillRule(draw2d.FillRuleEvenOdd)
+ gc.SetFillColor(color.NRGBA{0, 0xB2, 0, 0xFF})
+
+ gc.SetStrokeColor(image.Black)
+ gc.FillStroke(&wheel1, &wheel2)
+
+ draw2dkit.Rectangle(gc, x, y+sy*140, x+sx*232, y+sy*198)
+ wheel1.Clear()
+ wheel1.ArcTo(x+sx*52, y+sy*198, sx*40, sy*40, 0, 2*math.Pi)
+ wheel2.Clear()
+ wheel2.ArcTo(x+sx*180, y+sy*198, sx*40, sy*40, 0, -2*math.Pi)
+
+ gc.SetFillRule(draw2d.FillRuleWinding)
+ gc.SetFillColor(color.NRGBA{0, 0, 0xE5, 0xFF})
+ gc.FillStroke(&wheel1, &wheel2)
+}
+
+// PathTransform scales a path differently in horizontal and vertical direction.
+func PathTransform(gc draw2d.GraphicContext, x, y, width, height float64) {
+ gc.Save()
+ gc.SetLineWidth(width / 10)
+ gc.Translate(x+width/2, y+height/2)
+ gc.Scale(1, 4)
+ gc.ArcTo(0, 0, width/8, height/8, 0, math.Pi*2)
+ gc.Close()
+ gc.Stroke()
+ gc.Restore()
+}
+
+// Star draws many lines from a center.
+func Star(gc draw2d.GraphicContext, x, y, width, height float64) {
+ gc.Save()
+ gc.Translate(x+width/2, y+height/2)
+ gc.SetLineWidth(width / 40)
+ for i := 0.0; i < 360; i = i + 10 { // Go from 0 to 360 degrees in 10 degree steps
+ gc.Save() // Keep rotations temporary
+ gc.Rotate(i * (math.Pi / 180.0)) // Rotate by degrees on stack from 'for'
+ gc.MoveTo(0, 0)
+ gc.LineTo(width/2, 0)
+ gc.Stroke()
+ gc.Restore()
+ }
+ gc.Restore()
+}
+
+// Draw all figures in a nice 4x3 grid.
+func Draw(gc draw2d.GraphicContext, width, height float64) {
+ mx, my := width*0.025, height*0.025 // margin
+ dx, dy := (width-2*mx)/4, (height-2*my)/3
+ w, h := dx-2*mx, dy-2*my
+ x0, y := 2*mx, 2*my
+ x := x0
+ Bubble(gc, x, y, w, h)
+ x += dx
+ CurveRectangle(gc, x, y, w, h, color.NRGBA{0x80, 0, 0, 0x80}, color.NRGBA{0x80, 0x80, 0xFF, 0xFF})
+ x += dx
+ Dash(gc, x, y, w, h)
+ x += dx
+ Arc(gc, x, y, w, h)
+ x = x0
+ y += dy
+ ArcNegative(gc, x, y, w, h)
+ x += dx
+ CubicCurve(gc, x, y, w, h)
+ x += dx
+ FillString(gc, x, y, w, h)
+ x += dx
+ FillStroke(gc, x, y, w, h)
+ x = x0
+ y += dy
+ FillStyle(gc, x, y, w, h)
+ x += dx
+ PathTransform(gc, x, y, w, h)
+ x += dx
+ Star(gc, x, y, w, h)
+ x += dx
+ gopher2.Draw(gc, x, y, w, h/2)
+}
diff --git a/samples/gopher/gopher.go b/samples/gopher/gopher.go
new file mode 100644
index 0000000..f24bf6f
--- /dev/null
+++ b/samples/gopher/gopher.go
@@ -0,0 +1,73 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff
+
+// Package gopher draws a gopher avatar based on a svg of:
+// https://github.com/golang-samples/gopher-vector/
+package gopher
+
+import (
+ "image/color"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws a left hand and ear of a gopher. Afterwards it returns
+// the filename. This should only be used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ gc.Save()
+ gc.Scale(0.5, 0.5)
+ // Draw a (partial) gopher
+ Draw(gc)
+ gc.Restore()
+
+ // Return the output filename
+ return samples.Output("gopher", ext), nil
+}
+
+// Draw a left hand and ear of a gopher using a gc thanks to
+// https://github.com/golang-samples/gopher-vector/
+func Draw(gc draw2d.GraphicContext) {
+ // Initialize Stroke Attribute
+ gc.SetLineWidth(3)
+ gc.SetLineCap(draw2d.RoundCap)
+ gc.SetStrokeColor(color.Black)
+
+ // Left hand
+ //
+ gc.SetFillColor(color.RGBA{0xF6, 0xD2, 0xA2, 0xff})
+ gc.MoveTo(10.634, 300.493)
+ rCubicCurveTo(gc, 0.764, 15.751, 16.499, 8.463, 23.626, 3.539)
+ rCubicCurveTo(gc, 6.765, -4.675, 8.743, -0.789, 9.337, -10.015)
+ rCubicCurveTo(gc, 0.389, -6.064, 1.088, -12.128, 0.744, -18.216)
+ rCubicCurveTo(gc, -10.23, -0.927, -21.357, 1.509, -29.744, 7.602)
+ gc.CubicCurveTo(10.277, 286.542, 2.177, 296.561, 10.634, 300.493)
+ gc.FillStroke()
+
+ //
+ gc.MoveTo(10.634, 300.493)
+ rCubicCurveTo(gc, 2.29, -0.852, 4.717, -1.457, 6.271, -3.528)
+ gc.Stroke()
+
+ // Left Ear
+ //
+ gc.MoveTo(46.997, 112.853)
+ gc.CubicCurveTo(-13.3, 95.897, 31.536, 19.189, 79.956, 50.74)
+ gc.LineTo(46.997, 112.853)
+ gc.Close()
+ gc.Stroke()
+}
+
+func rQuadCurveTo(path draw2d.PathBuilder, dcx, dcy, dx, dy float64) {
+ x, y := path.LastPoint()
+ path.QuadCurveTo(x+dcx, y+dcy, x+dx, y+dy)
+}
+
+func rCubicCurveTo(path draw2d.PathBuilder, dcx1, dcy1, dcx2, dcy2, dx, dy float64) {
+ x, y := path.LastPoint()
+ path.CubicCurveTo(x+dcx1, y+dcy1, x+dcx2, y+dcy2, x+dx, y+dy)
+}
diff --git a/samples/gopher2/gopher2.go b/samples/gopher2/gopher2.go
new file mode 100644
index 0000000..3327cb4
--- /dev/null
+++ b/samples/gopher2/gopher2.go
@@ -0,0 +1,122 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff
+
+// Package gopher2 draws a gopher avatar based on a svg of:
+// https://github.com/golang-samples/gopher-vector/
+package gopher2
+
+import (
+ "image"
+ "image/color"
+ "math"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws a rotated face of the gopher. Afterwards it returns
+// the filename. This should only be used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ gc.SetStrokeColor(image.Black)
+ gc.SetFillColor(image.White)
+ gc.Save()
+ // Draw a (partial) gopher
+ gc.Translate(-60, 65)
+ gc.Rotate(-30 * (math.Pi / 180.0))
+ Draw(gc, 48, 48, 240, 72)
+ gc.Restore()
+
+ // Return the output filename
+ return samples.Output("gopher2", ext), nil
+}
+
+// Draw a gopher head (not rotated)
+func Draw(gc draw2d.GraphicContext, x, y, w, h float64) {
+ h23 := (h * 2) / 3
+
+ blf := color.RGBA{0, 0, 0, 0xff} // black
+ wf := color.RGBA{0xff, 0xff, 0xff, 0xff} // white
+ nf := color.RGBA{0x8B, 0x45, 0x13, 0xff} // brown opaque
+ brf := color.RGBA{0x8B, 0x45, 0x13, 0x99} // brown transparant
+ brb := color.RGBA{0x8B, 0x45, 0x13, 0xBB} // brown transparant
+
+ // round head top
+ gc.MoveTo(x, y+h*1.002)
+ gc.CubicCurveTo(x+w/4, y-h/3, x+3*w/4, y-h/3, x+w, y+h*1.002)
+ gc.Close()
+ gc.SetFillColor(brb)
+ gc.Fill()
+
+ // rectangle head bottom
+ draw2dkit.RoundedRectangle(gc, x, y+h, x+w, y+h+h, h/5, h/5)
+ gc.Fill()
+
+ // left ear outside
+ draw2dkit.Circle(gc, x, y+h, w/12)
+ gc.SetFillColor(brf)
+ gc.Fill()
+
+ // left ear inside
+ draw2dkit.Circle(gc, x, y+h, 0.5*w/12)
+ gc.SetFillColor(nf)
+ gc.Fill()
+
+ // right ear outside
+ draw2dkit.Circle(gc, x+w, y+h, w/12)
+ gc.SetFillColor(brf)
+ gc.Fill()
+
+ // right ear inside
+ draw2dkit.Circle(gc, x+w, y+h, 0.5*w/12)
+ gc.SetFillColor(nf)
+ gc.Fill()
+
+ // left eye outside white
+ draw2dkit.Circle(gc, x+w/3, y+h23, w/9)
+ gc.SetFillColor(wf)
+ gc.Fill()
+
+ // left eye black
+ draw2dkit.Circle(gc, x+w/3+w/24, y+h23, 0.5*w/9)
+ gc.SetFillColor(blf)
+ gc.Fill()
+
+ // left eye inside white
+ draw2dkit.Circle(gc, x+w/3+w/24+w/48, y+h23, 0.2*w/9)
+ gc.SetFillColor(wf)
+ gc.Fill()
+
+ // right eye outside white
+ draw2dkit.Circle(gc, x+w-w/3, y+h23, w/9)
+ gc.Fill()
+
+ // right eye black
+ draw2dkit.Circle(gc, x+w-w/3+w/24, y+h23, 0.5*w/9)
+ gc.SetFillColor(blf)
+ gc.Fill()
+
+ // right eye inside white
+ draw2dkit.Circle(gc, x+w-(w/3)+w/24+w/48, y+h23, 0.2*w/9)
+ gc.SetFillColor(wf)
+ gc.Fill()
+
+ // left tooth
+ gc.SetFillColor(wf)
+ draw2dkit.RoundedRectangle(gc, x+w/2-w/8, y+h+h/2.5, x+w/2-w/8+w/8, y+h+h/2.5+w/6, w/10, w/10)
+ gc.Fill()
+
+ // right tooth
+ draw2dkit.RoundedRectangle(gc, x+w/2, y+h+h/2.5, x+w/2+w/8, y+h+h/2.5+w/6, w/10, w/10)
+ gc.Fill()
+
+ // snout
+ draw2dkit.Ellipse(gc, x+(w/2), y+h+h/2.5, w/6, w/12)
+ gc.SetFillColor(nf)
+ gc.Fill()
+
+ // nose
+ draw2dkit.Ellipse(gc, x+(w/2), y+h+h/7, w/10, w/12)
+ gc.SetFillColor(blf)
+ gc.Fill()
+}
diff --git a/samples/helloworld/helloworld.go b/samples/helloworld/helloworld.go
new file mode 100644
index 0000000..f9bf410
--- /dev/null
+++ b/samples/helloworld/helloworld.go
@@ -0,0 +1,40 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff, Stani Michiels
+
+// Package helloworld displays multiple "Hello World" (one rotated)
+// in a rounded rectangle.
+package helloworld
+
+import (
+ "fmt"
+ "image"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws "Hello World" and returns the filename. This should only be
+// used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ // Draw hello world
+ Draw(gc, fmt.Sprintf("Hello World %d dpi", gc.GetDPI()))
+
+ // Return the output filename
+ return samples.Output("helloworld", ext), nil
+}
+
+// Draw "Hello World"
+func Draw(gc draw2d.GraphicContext, text string) {
+ // Draw a rounded rectangle using default colors
+ draw2dkit.RoundedRectangle(gc, 5, 5, 135, 95, 10, 10)
+ gc.FillStroke()
+
+ // Set the font luximbi.ttf
+ gc.SetFontData(draw2d.FontData{"luxi", draw2d.FontFamilyMono, draw2d.FontStyleBold | draw2d.FontStyleItalic})
+ // Set the fill text color to black
+ gc.SetFillColor(image.Black)
+ gc.SetFontSize(14)
+ // Display Hello World
+ gc.FillStringAt("Hello World", 8, 52)
+}
diff --git a/samples/helloworldgl/helloworldgl.go b/samples/helloworldgl/helloworldgl.go
new file mode 100644
index 0000000..be89db5
--- /dev/null
+++ b/samples/helloworldgl/helloworldgl.go
@@ -0,0 +1,119 @@
+// Open an OpenGl window and display a rectangle using a OpenGl GraphicContext
+package main
+
+import (
+ "image/color"
+ "log"
+ "runtime"
+
+ "github.com/go-gl/gl/v2.1/gl"
+ "github.com/go-gl/glfw/v3.1/glfw"
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dgl"
+ "github.com/llgcode/draw2d/draw2dkit"
+)
+
+var (
+ // global rotation
+ rotate int
+ width, height int
+ redraw = true
+ font draw2d.FontData
+)
+
+func reshape(window *glfw.Window, w, h int) {
+ gl.ClearColor(1, 1, 1, 1)
+ /* Establish viewing area to cover entire window. */
+ gl.Viewport(0, 0, int32(w), int32(h))
+ /* PROJECTION Matrix mode. */
+ gl.MatrixMode(gl.PROJECTION)
+ /* Reset project matrix. */
+ gl.LoadIdentity()
+ /* Map abstract coords directly to window coords. */
+ gl.Ortho(0, float64(w), 0, float64(h), -1, 1)
+ /* Invert Y axis so increasing Y goes down. */
+ gl.Scalef(1, -1, 1)
+ /* Shift origin up to upper-left corner. */
+ gl.Translatef(0, float32(-h), 0)
+ gl.Enable(gl.BLEND)
+ gl.BlendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA)
+ gl.Disable(gl.DEPTH_TEST)
+ width, height = w, h
+ redraw = true
+}
+
+// Ask to refresh
+func invalidate() {
+ redraw = true
+}
+
+func display() {
+ gl.Clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT)
+
+ gl.LineWidth(1)
+ gc := draw2dgl.NewGraphicContext(width, height)
+ gc.SetFontData(draw2d.FontData{
+ Name: "luxi",
+ Family: draw2d.FontFamilyMono,
+ Style: draw2d.FontStyleBold | draw2d.FontStyleItalic})
+
+ gc.BeginPath()
+ draw2dkit.RoundedRectangle(gc, 200, 200, 600, 600, 100, 100)
+
+ gc.SetFillColor(color.RGBA{0, 0, 0, 0xff})
+ gc.Fill()
+
+ gl.Flush() /* Single buffered, so needs a flush. */
+}
+
+func init() {
+ runtime.LockOSThread()
+}
+
+func main() {
+ err := glfw.Init()
+ if err != nil {
+ panic(err)
+ }
+ defer glfw.Terminate()
+ width, height = 800, 800
+ window, err := glfw.CreateWindow(width, height, "Show RoundedRect", nil, nil)
+ if err != nil {
+ panic(err)
+ }
+
+ window.MakeContextCurrent()
+ window.SetSizeCallback(reshape)
+ window.SetKeyCallback(onKey)
+ window.SetCharCallback(onChar)
+
+ glfw.SwapInterval(1)
+
+ err = gl.Init()
+ if err != nil {
+ panic(err)
+ }
+
+ reshape(window, width, height)
+ for !window.ShouldClose() {
+ if redraw {
+ display()
+ window.SwapBuffers()
+ redraw = false
+ }
+ glfw.PollEvents()
+ // time.Sleep(2 * time.Second)
+ }
+}
+
+func onChar(w *glfw.Window, char rune) {
+ log.Println(char)
+}
+
+func onKey(w *glfw.Window, key glfw.Key, scancode int, action glfw.Action, mods glfw.ModifierKey) {
+ switch {
+ case key == glfw.KeyEscape && action == glfw.Press,
+ key == glfw.KeyQ && action == glfw.Press:
+ w.SetShouldClose(true)
+ }
+}
diff --git a/samples/line/line.go b/samples/line/line.go
new file mode 100644
index 0000000..f106cc4
--- /dev/null
+++ b/samples/line/line.go
@@ -0,0 +1,39 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff, Stani Michiels
+
+// Package line draws vertically spaced lines.
+package line
+
+import (
+ "image/color"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dkit"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws vertically spaced lines and returns the filename.
+// This should only be used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ gc.SetFillRule(draw2d.FillRuleWinding)
+ gc.Clear()
+ // Draw the line
+ for x := 5.0; x < 297; x += 10 {
+ Draw(gc, x, 0, x, 210)
+ }
+ gc.ClearRect(100, 75, 197, 135)
+ draw2dkit.Ellipse(gc, 148.5, 105, 35, 25)
+ gc.SetFillColor(color.RGBA{0xff, 0xff, 0x44, 0xff})
+ gc.FillStroke()
+
+ // Return the output filename
+ return samples.Output("line", ext), nil
+}
+
+// Draw vertically spaced lines
+func Draw(gc draw2d.GraphicContext, x0, y0, x1, y1 float64) {
+ // Draw a line
+ gc.MoveTo(x0, y0)
+ gc.LineTo(x1, y1)
+ gc.Stroke()
+}
diff --git a/samples/linecapjoin/linecapjoin.go b/samples/linecapjoin/linecapjoin.go
new file mode 100644
index 0000000..38e80e8
--- /dev/null
+++ b/samples/linecapjoin/linecapjoin.go
@@ -0,0 +1,52 @@
+// Copyright 2010 The draw2d Authors. All rights reserved.
+// created: 21/11/2010 by Laurent Le Goff
+
+// Package linecapjoin demonstrates the different line caps and joins.
+package linecapjoin
+
+import (
+ "image/color"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws the different line caps and joins.
+// This should only be used during testing.
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ // Draw the line
+ const offset = 75.0
+ x := 35.0
+ caps := []draw2d.LineCap{draw2d.ButtCap, draw2d.SquareCap, draw2d.RoundCap}
+ joins := []draw2d.LineJoin{draw2d.BevelJoin, draw2d.MiterJoin, draw2d.RoundJoin}
+ for i := range caps {
+ Draw(gc, caps[i], joins[i], x, 50, x, 160, offset)
+ x += offset
+ }
+
+ // Return the output filename
+ return samples.Output("linecapjoin", ext), nil
+}
+
+// Draw a line with an angle with specified line cap and join
+func Draw(gc draw2d.GraphicContext, cap draw2d.LineCap, join draw2d.LineJoin,
+ x0, y0, x1, y1, offset float64) {
+ gc.SetLineCap(cap)
+ gc.SetLineJoin(join)
+
+ // Draw thick line
+ gc.SetStrokeColor(color.NRGBA{0x33, 0x33, 0x33, 0xFF})
+ gc.SetLineWidth(30.0)
+ gc.MoveTo(x0, y0)
+ gc.LineTo((x0+x1)/2+offset, (y0+y1)/2)
+ gc.LineTo(x1, y1)
+ gc.Stroke()
+
+ // Draw thin helping line
+ gc.SetStrokeColor(color.NRGBA{0xFF, 0x33, 0x33, 0xFF})
+ gc.SetLineWidth(2.56)
+ gc.MoveTo(x0, y0)
+ gc.LineTo((x0+x1)/2+offset, (y0+y1)/2)
+ gc.LineTo(x1, y1)
+ gc.Stroke()
+}
diff --git a/samples/postscript/postscript.go b/samples/postscript/postscript.go
new file mode 100644
index 0000000..f1d88c1
--- /dev/null
+++ b/samples/postscript/postscript.go
@@ -0,0 +1,49 @@
+// Package postscript reads the tiger.ps file and draws it to a backend.
+package postscript
+
+import (
+ "io/ioutil"
+ "os"
+ "strings"
+
+ "github.com/llgcode/ps"
+
+ "github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/samples"
+)
+
+// Main draws the tiger
+func Main(gc draw2d.GraphicContext, ext string) (string, error) {
+ gc.Save()
+
+ // flip the image
+ gc.Translate(0, 200)
+ gc.Scale(0.35, -0.35)
+ gc.Translate(70, -200)
+
+ // Tiger postscript drawing
+ tiger := samples.Resource("image", "tiger.ps", ext)
+
+ // Draw tiger
+ Draw(gc, tiger)
+ gc.Restore()
+
+ // Return the output filename
+ return samples.Output("postscript", ext), nil
+}
+
+// Draw a tiger
+func Draw(gc draw2d.GraphicContext, filename string) {
+ // Open the postscript
+ src, err := os.OpenFile(filename, 0, 0)
+ if err != nil {
+ panic(err)
+ }
+ defer src.Close()
+ bytes, err := ioutil.ReadAll(src)
+ reader := strings.NewReader(string(bytes))
+
+ // Initialize and interpret the postscript
+ interpreter := ps.NewInterpreter(gc)
+ interpreter.Execute(reader)
+}
diff --git a/samples/postscriptgl/postscriptgl.go b/samples/postscriptgl/postscriptgl.go
new file mode 100644
index 0000000..9e75adb
--- /dev/null
+++ b/samples/postscriptgl/postscriptgl.go
@@ -0,0 +1,114 @@
+// Open a OpenGL window and display a tiger interpreting a postscript file
+package main
+
+import (
+ "io/ioutil"
+ "log"
+ "math"
+ "os"
+ "runtime"
+ "strings"
+ "time"
+
+ "github.com/go-gl/gl/v2.1/gl"
+ "github.com/go-gl/glfw/v3.1/glfw"
+ "github.com/llgcode/draw2d/draw2dgl"
+ "github.com/llgcode/ps"
+)
+
+var postscriptContent string
+
+var (
+ width, height int
+ rotate int
+ window *glfw.Window
+)
+
+func reshape(window *glfw.Window, w, h int) {
+ gl.ClearColor(1, 1, 1, 1)
+ //fmt.Println(gl.GetString(gl.EXTENSIONS))
+ gl.Viewport(0, 0, int32(w), int32(h)) /* Establish viewing area to cover entire window. */
+ gl.MatrixMode(gl.PROJECTION) /* Start modifying the projection matrix. */
+ gl.LoadIdentity() /* Reset project matrix. */
+ gl.Ortho(0, float64(w), 0, float64(h), -1, 1) /* Map abstract coords directly to window coords. */
+ gl.Scalef(1, -1, 1) /* Invert Y axis so increasing Y goes down. */
+ gl.Translatef(0, float32(-h), 0) /* Shift origin up to upper-left corner. */
+ gl.Enable(gl.BLEND)
+ gl.BlendFunc(gl.SRC_ALPHA, gl.ONE_MINUS_SRC_ALPHA)
+ gl.Disable(gl.DEPTH_TEST)
+ width, height = w, h
+}
+
+func display() {
+
+ gl.Clear(gl.COLOR_BUFFER_BIT | gl.DEPTH_BUFFER_BIT)
+
+ lastTime := time.Now()
+ gl.LineWidth(1)
+ gc := draw2dgl.NewGraphicContext(width, height)
+
+ gc.Translate(380, 400)
+ gc.Scale(1, -1)
+ rotate = (rotate + 1) % 360
+ gc.Rotate(float64(rotate) * math.Pi / 180)
+ gc.Translate(-380, -400)
+
+ interpreter := ps.NewInterpreter(gc)
+ reader := strings.NewReader(postscriptContent)
+
+ interpreter.Execute(reader)
+ dt := time.Now().Sub(lastTime)
+ log.Printf("Redraw in : %f ms\n", float64(dt)*1e-6)
+ gl.Flush() /* Single buffered, so needs a flush. */
+}
+
+func main() {
+ src, err := os.OpenFile("tiger.ps", 0, 0)
+ if err != nil {
+ log.Println("can't find postscript file.")
+ return
+ }
+ defer src.Close()
+ bytes, err := ioutil.ReadAll(src)
+ postscriptContent = string(bytes)
+ err = glfw.Init()
+ if err != nil {
+ panic(err)
+ }
+ defer glfw.Terminate()
+
+ window, err = glfw.CreateWindow(800, 800, "Show Tiger in OpenGL", nil, nil)
+ if err != nil {
+ panic(err)
+ }
+
+ window.MakeContextCurrent()
+ window.SetSizeCallback(reshape)
+ window.SetKeyCallback(onKey)
+
+ glfw.SwapInterval(1)
+
+ err = gl.Init()
+ if err != nil {
+ panic(err)
+ }
+ reshape(window, 800, 800)
+ for !window.ShouldClose() {
+ display()
+ window.SwapBuffers()
+ glfw.PollEvents()
+ // time.Sleep(2 * time.Second)
+ }
+}
+
+func onKey(w *glfw.Window, key glfw.Key, scancode int, action glfw.Action, mods glfw.ModifierKey) {
+ switch {
+ case key == glfw.KeyEscape && action == glfw.Press,
+ key == glfw.KeyQ && action == glfw.Press:
+ w.SetShouldClose(true)
+ }
+}
+
+func init() {
+ runtime.LockOSThread()
+}
diff --git a/samples/postscriptgl/tiger.ps b/samples/postscriptgl/tiger.ps
new file mode 100644
index 0000000..24a9dce
--- /dev/null
+++ b/samples/postscriptgl/tiger.ps
@@ -0,0 +1,2733 @@
+%!PS-Adobe-2.0 EPSF-1.2
+%%Creator: Adobe Illustrator(TM) 1.2d4
+%%For: OpenWindows Version 2
+%%Title: tiger.eps
+%%CreationDate: 4/12/90 3:20 AM
+%%DocumentProcSets: Adobe_Illustrator_1.2d1 0 0
+%%DocumentSuppliedProcSets: Adobe_Illustrator_1.2d1 0 0
+%%BoundingBox: 22 171 567 738
+%%EndComments
+
+%%BeginProcSet:Adobe_Illustrator_1.2d1 0 0
+
+/Adobe_Illustrator_1.2d1 dup 100 dict def load begin
+% definition operators
+/bdef {bind def} bind def
+/ldef {load def} bdef
+/xdef {exch def} bdef
+% graphic state operators
+/_K { 3 index add neg dup 0 lt {pop 0} if 3 1 roll } bdef
+/_k /setcmybcolor where {
+ /setcmybcolor get
+} {
+ { 1 sub 4 1 roll _K _K _K setrgbcolor pop } bind
+} ifelse def
+/g {/_b xdef /p {_b setgray} def} bdef
+/G {/_B xdef /P {_B setgray} def} bdef
+/k {/_b xdef /_y xdef /_m xdef /_c xdef /p {_c _m _y _b _k} def} bdef
+/K {/_B xdef /_Y xdef /_M xdef /_C xdef /P {_C _M _Y _B _k} def} bdef
+/d /setdash ldef
+/_i currentflat def
+/i {dup 0 eq {pop _i} if setflat} bdef
+/j /setlinejoin ldef
+/J /setlinecap ldef
+/M /setmiterlimit ldef
+/w /setlinewidth ldef
+% path construction operators
+/_R {.25 sub round .25 add} bdef
+/_r {transform _R exch _R exch itransform} bdef
+/c {_r curveto} bdef
+/C /c ldef
+/v {currentpoint 6 2 roll _r curveto} bdef
+/V /v ldef
+/y {_r 2 copy curveto} bdef
+/Y /y ldef
+/l {_r lineto} bdef
+/L /l ldef
+/m {_r moveto} bdef
+% path painting operators
+/n /newpath ldef
+/N /n ldef
+/F {p fill} bdef
+/f {closepath F} bdef
+/S {P stroke} bdef
+/s {closepath S} bdef
+/B {gsave F grestore S} bdef
+/b {closepath B} bdef
+end
+%%EndProcSet
+%%EndProlog
+
+%%Page: 1 1
+
+Adobe_Illustrator_1.2d1 begin
+
+.8 setgray
+clippath fill
+-110 -300 translate
+1.1 dup scale
+
+0 g
+0 G
+0 i
+0 J
+0 j
+0.172 w
+10 M
+[]0 d
+0 0 0 0 k
+
+177.696 715.715 m
+177.797 713.821 176.973 713.84 v
+176.149 713.859 159.695 761.934 139.167 759.691 C
+156.95 767.044 177.696 715.715 V
+b
+181.226 718.738 m
+180.677 716.922 179.908 717.221 v
+179.14 717.519 180.023 768.325 159.957 773.199 C
+179.18 774.063 181.226 718.738 V
+b
+208.716 676.41 m
+210.352 675.45 209.882 674.773 v
+209.411 674.096 160.237 686.898 150.782 668.541 C
+154.461 687.428 208.716 676.41 V
+b
+205.907 666.199 m
+207.763 665.803 207.529 665.012 v
+207.296 664.221 156.593 660.879 153.403 640.478 C
+150.945 659.563 205.907 666.199 V
+b
+201.696 671.724 m
+203.474 671.061 203.128 670.313 v
+202.782 669.565 152.134 673.654 146.002 653.936 C
+146.354 673.175 201.696 671.724 V
+b
+190.991 689.928 m
+192.299 688.554 191.66 688.033 v
+191.021 687.512 147.278 713.366 133.131 698.324 C
+141.872 715.467 190.991 689.928 V
+b
+183.446 685.737 m
+184.902 684.52 184.326 683.929 v
+183.75 683.339 137.362 704.078 125.008 687.531 C
+131.753 705.553 183.446 685.737 V
+b
+180.846 681.665 m
+182.454 680.657 181.964 679.994 v
+181.474 679.331 132.692 693.554 122.709 675.478 C
+126.934 694.251 180.846 681.665 V
+b
+191.58 681.051 m
+192.702 679.52 192.001 679.085 v
+191.3 678.65 151.231 709.898 135.273 696.793 C
+146.138 712.674 191.58 681.051 V
+b
+171.8 710 m
+172.4 708.2 171.6 708 v
+170.8 707.8 142.2 749.8 122.999 742.2 C
+138.2 754 171.8 710 V
+b
+172.495 703.021 m
+173.47 701.392 172.731 701.025 v
+171.993 700.657 135.008 735.501 117.899 723.939 C
+130.196 738.739 172.495 703.021 V
+b
+172.38 698.651 m
+173.502 697.12 172.801 696.685 v
+172.1 696.251 132.031 727.498 116.073 714.393 C
+126.938 730.274 172.38 698.651 V
+b
+0 J 1 w
+170.17 696.935 m
+170.673 690.887 171.661 684.318 173.4 681.199 C
+169.8 668.799 178.6 655.599 V
+178.2 648.399 179.8 645.199 V
+183.8 636.799 188.6 635.999 v
+192.484 635.352 201.207 632.283 211.068 630.879 c
+228.2 616.799 225 603.999 V
+224.6 587.599 221 585.999 V
+232.6 597.199 223 580.399 V
+218.6 561.599 l
+244.2 583.199 228.6 564.799 V
+218.6 538.799 l
+238.2 557.199 231 548.799 V
+227.8 539.999 l
+271 567.199 240.2 537.599 V
+248.2 541.199 252.6 538.399 V
+259.4 539.599 258.6 537.999 V
+237.8 527.599 234.2 509.199 V
+242.6 519.199 239.4 508.399 V
+239.8 496.799 l
+243.8 518.399 243.4 480.799 V
+262.6 498.799 251 477.999 V
+251 461.199 l
+266.2 477.599 259.8 464.799 V
+269.8 473.599 265.8 458.399 V
+265 447.999 269.4 459.199 V
+285.4 489.799 279.4 463.599 V
+278.6 444.399 283.4 459.199 V
+283.8 448.799 293 441.599 V
+291.8 492.399 304.6 456.399 V
+308.6 439.999 l
+311.4 449.199 311 454.399 V
+325.8 470.799 319 446.399 V
+334.2 469.199 331 455.999 V
+323.4 439.999 325 435.199 V
+341.8 469.999 343 471.599 V
+341 429.198 351.8 465.199 V
+357.4 453.199 354.6 448.799 V
+362.6 456.799 361.8 459.999 V
+366.4 468.199 369.2 454.599 V
+371 445.199 372.6 448.399 V
+376.6 424.398 377.8 447.199 V
+379.4 460.799 372.2 472.399 V
+373 475.599 370.2 479.599 v
+383.8 457.999 376.6 486.799 V
+387.801 478.799 389.001 478.799 V
+375.4 501.999 384.2 497.199 V
+379 507.599 397.001 495.599 V
+381 511.599 398.601 501.999 V
+406.601 495.599 399.001 505.599 V
+384.6 521.599 406.601 503.599 V
+418.201 487.199 419.001 484.399 V
+409.001 513.599 404.601 516.399 V
+413.001 552.799 454.201 537.199 V
+461.001 519.999 465.401 538.399 V
+478.201 544.799 489.401 517.199 V
+493.401 530.799 492.601 533.599 V
+499.401 532.399 498.601 533.599 V
+511.801 529.199 513.001 529.999 V
+519.801 523.199 520.201 526.799 V
+529.401 523.999 527.401 527.599 V
+536.201 511.999 536.601 508.399 V
+539.001 522.399 l
+541.001 519.599 l
+542.601 527.199 541.801 528.399 v
+541.001 529.599 561.801 521.599 566.601 500.799 C
+568.601 492.399 l
+574.601 507.199 573.001 511.199 V
+578.201 510.399 578.601 505.999 V
+582.601 529.199 577.801 535.199 V
+582.201 535.999 583.401 532.399 V
+583.401 539.599 l
+590.601 538.799 590.601 541.199 V
+595.001 545.199 597.001 540.399 V
+584.601 575.599 603.001 556.399 V
+610.201 545.599 606.601 564.399 v
+603.001 583.199 599.001 584.799 603.801 585.199 C
+604.601 588.799 602.601 590.399 v
+600.601 591.999 603.801 590.399 y
+608.601 586.399 603.401 608.399 V
+609.801 606.799 597.801 635.999 V
+600.601 638.399 596.601 646.799 V
+604.601 642.399 607.401 643.999 V
+607.001 645.599 603.801 649.599 V
+582.201 704.4 602.601 682.399 V
+614.451 668.849 608.051 691.649 V
+598.94 715.659 599.717 719.955 V
+170.17 696.935 l
+b
+0.2 0.55 0.85 0 k
+599.717 719.755 m
+600.345 719.574 602.551 718.45 603.801 716.8 C
+610.601 706 605.401 724.4 V
+596.201 753.2 605.001 742 V
+611.001 734.8 607.801 748.4 v
+603.936 764.827 601.401 771.2 y
+613.001 766.4 586.201 806 V
+595.001 802.4 l
+575.401 842 553.801 847.2 V
+545.801 853.2 l
+584.201 891.2 571.401 928 V
+564.601 933.2 555.001 924 V
+548.601 919.2 542.601 920.8 V
+511.801 919.6 509.801 919.6 v
+507.801 919.6 473.001 956.8 407.401 939.2 C
+402.201 937.2 397.801 938.4 V
+379.4 954.4 330.6 931.6 v
+320.6 929.6 319 929.6 v
+317.4 929.6 314.6 929.6 306.6 923.2 c
+298.6 916.8 298.2 916 296.2 914.4 C
+279.8 903.2 275 902.4 V
+263.4 896 259 886 V
+255.4 884.8 l
+253.8 877.6 253.4 876.4 V
+248.6 872.8 247.8 867.2 V
+239 861.2 239.4 856.8 V
+237.8 851.6 237 846.8 V
+229.8 842 230.6 839.2 V
+223 825.2 224.2 818.4 V
+217.8 818.8 215 816.4 V
+214.2 811.6 212.6 811.2 V
+209.8 810 212.2 806 V
+210.6 803.2 210.2 801.6 V
+211 798.8 206.6 793.2 V
+200.2 774.4 202.2 769.2 V
+202.6 764.4 199.8 762.8 V
+196.2 763.2 204.6 751.2 V
+205.4 750 202.2 747.6 V
+185 744 182.6 727.6 V
+169 712.8 169 707.6 v
+169 705.295 169.271 702.148 169.97 697.535 C
+169.4 689.199 197 688.399 v
+224.6 687.599 599.717 719.755 Y
+b
+184.4 697.4 m
+159.4 736.8 173.8 680.399 Y
+182.6 645.999 312.2 683.599 y
+481.001 714 492.201 718 v
+503.401 722 598.601 715.6 y
+593.001 732.4 L
+528.201 778.8 509.001 755.6 495.401 759.6 c
+481.801 763.6 484.201 754 481.001 753.2 c
+477.801 752.4 438.601 777.2 432.201 776.4 c
+425.801 775.6 400.459 799.351 415.401 767.6 c
+431.401 733.6 357 728.4 340.2 739.6 c
+323.4 750.8 347.4 721.2 Y
+365.8 701.2 331.4 718 y
+297 730.8 273 705.2 269.8 704.4 c
+266.6 703.6 261.8 700.4 261 706.8 c
+260.2 713.2 252.69 729.901 221 703.6 c
+201 686.999 187.2 709 Y
+184.4 697.4 L
+f
+0.09 0.5 0.772 0 k
+433.51 774.654 m
+427.11 773.854 401.743 797.593 416.71 765.854 c
+433.31 730.654 358.31 726.654 341.51 737.854 c
+324.709 749.054 348.71 719.454 Y
+367.11 699.454 332.709 716.254 y
+298.309 729.054 274.309 703.454 271.109 702.654 c
+267.909 701.854 263.109 698.654 262.309 705.054 c
+261.509 711.454 254.13 727.988 222.309 701.854 c
+201.073 684.508 187.582 705.963 Y
+184.382 695.854 L
+159.382 735.654 174.454 677.345 Y
+183.255 642.944 313.509 681.854 y
+482.31 712.254 493.51 716.254 v
+504.71 720.254 599.038 713.927 y
+593.51 731.236 L
+528.71 777.636 510.31 753.854 496.71 757.854 c
+483.11 761.854 485.51 752.254 482.31 751.454 c
+479.11 750.654 439.91 775.454 433.51 774.654 c
+f
+0.081 0.45 0.695 0 k
+434.819 772.909 m
+428.419 772.109 403.685 796.138 418.019 764.109 c
+434.219 727.908 359.619 724.908 342.819 736.108 c
+326.019 747.308 350.019 717.708 Y
+368.419 697.708 334.019 714.508 y
+299.619 727.308 275.618 701.708 272.418 700.908 c
+269.218 700.108 264.418 696.908 263.618 703.308 c
+262.818 709.708 255.57 726.075 223.618 700.108 c
+201.145 682.017 187.964 702.926 Y
+184.364 694.308 L
+160.564 733.308 175.109 674.29 Y
+183.909 639.89 314.819 680.108 y
+483.619 710.508 494.819 714.508 v
+506.019 718.508 599.474 712.254 y
+594.02 730.072 L
+529.219 776.472 511.619 752.109 498.019 756.109 c
+484.419 760.109 486.819 750.509 483.619 749.708 c
+480.419 748.908 441.219 773.709 434.819 772.909 c
+f
+0.072 0.4 0.618 0 k
+436.128 771.163 m
+429.728 770.363 404.999 794.395 419.328 762.363 c
+436.128 724.807 360.394 723.518 344.128 734.363 c
+327.328 745.563 351.328 715.963 Y
+369.728 695.963 335.328 712.763 y
+300.928 725.563 276.928 699.963 273.728 699.163 c
+270.528 698.363 265.728 695.163 264.928 701.563 c
+264.128 707.963 257.011 724.161 224.927 698.363 c
+201.218 679.526 188.345 699.89 Y
+184.345 692.763 L
+162.545 729.563 175.764 671.235 Y
+184.564 636.835 316.128 678.363 y
+484.928 708.763 496.129 712.763 v
+507.329 716.763 599.911 710.581 y
+594.529 728.908 L
+529.729 775.309 512.929 750.363 499.329 754.363 c
+485.728 758.363 488.128 748.763 484.928 747.963 c
+481.728 747.163 442.528 771.963 436.128 771.163 c
+f
+0.063 0.35 0.54 0 k
+437.438 769.417 m
+431.037 768.617 406.814 792.871 420.637 760.617 c
+437.438 721.417 362.237 721.417 345.437 732.617 c
+328.637 743.817 352.637 714.217 Y
+371.037 694.217 336.637 711.017 y
+302.237 723.817 278.237 698.217 275.037 697.417 c
+271.837 696.617 267.037 693.417 266.237 699.817 c
+265.437 706.217 258.452 722.248 226.237 696.617 c
+201.291 677.035 188.727 696.854 Y
+184.327 691.217 L
+164.527 726.018 176.418 668.181 Y
+185.218 633.78 317.437 676.617 y
+486.238 707.017 497.438 711.017 v
+508.638 715.017 600.347 708.908 y
+595.038 727.745 L
+530.238 774.145 514.238 748.617 500.638 752.617 c
+487.038 756.617 489.438 747.017 486.238 746.217 c
+483.038 745.417 443.838 770.217 437.438 769.417 c
+f
+0.054 0.3 0.463 0 k
+438.747 767.672 m
+432.347 766.872 406.383 790.323 421.947 758.872 c
+441.147 720.072 363.546 719.672 346.746 730.872 c
+329.946 742.072 353.946 712.472 Y
+372.346 692.472 337.946 709.272 y
+303.546 722.072 279.546 696.472 276.346 695.672 c
+273.146 694.872 268.346 691.672 267.546 698.072 c
+266.746 704.472 259.892 720.335 227.546 694.872 c
+201.364 674.544 189.109 693.817 Y
+184.309 689.672 L
+166.309 722.872 177.073 665.126 Y
+185.873 630.726 318.746 674.872 y
+487.547 705.272 498.747 709.272 v
+509.947 713.272 600.783 707.236 y
+595.547 726.581 L
+530.747 772.981 515.547 746.872 501.947 750.872 c
+488.347 754.872 490.747 745.272 487.547 744.472 c
+484.347 743.672 445.147 768.472 438.747 767.672 c
+f
+0.045 0.25 0.386 0 k
+440.056 765.927 m
+433.655 765.127 407.313 788.387 423.255 757.127 c
+443.656 717.126 364.855 717.926 348.055 729.126 c
+331.255 740.326 355.255 710.726 Y
+373.655 690.726 339.255 707.526 y
+304.855 720.326 280.855 694.726 277.655 693.926 c
+274.455 693.126 269.655 689.926 268.855 696.326 c
+268.055 702.726 261.332 718.422 228.855 693.126 c
+201.436 672.053 189.491 690.781 Y
+184.291 688.126 L
+168.291 718.326 177.727 662.071 Y
+186.527 627.671 320.055 673.126 y
+488.856 703.526 500.056 707.526 v
+511.256 711.526 601.22 705.563 y
+596.056 725.417 L
+531.256 771.817 516.856 745.126 503.256 749.126 c
+489.656 753.127 492.056 743.526 488.856 742.726 c
+485.656 741.926 446.456 766.727 440.056 765.927 c
+f
+0.036 0.2 0.309 0 k
+441.365 764.181 m
+434.965 763.381 407.523 786.056 424.565 755.381 c
+446.565 715.781 366.164 716.181 349.364 727.381 c
+332.564 738.581 356.564 708.981 Y
+374.964 688.981 340.564 705.781 y
+306.164 718.581 282.164 692.981 278.964 692.181 c
+275.764 691.381 270.964 688.181 270.164 694.581 c
+269.364 700.981 262.773 716.508 230.164 691.381 c
+201.509 669.562 189.873 687.744 Y
+184.273 686.581 L
+169.872 714.981 178.382 659.017 Y
+187.182 624.616 321.364 671.381 y
+490.165 701.781 501.365 705.781 v
+512.565 709.781 601.656 703.89 y
+596.565 724.254 L
+531.765 770.654 518.165 743.381 504.565 747.381 c
+490.965 751.381 493.365 741.781 490.165 740.981 c
+486.965 740.181 447.765 764.981 441.365 764.181 c
+f
+0.027 0.15 0.231 0 k
+442.674 762.435 m
+436.274 761.635 408.832 784.311 425.874 753.635 c
+447.874 714.035 367.474 714.435 350.674 725.635 c
+333.874 736.835 357.874 707.235 Y
+376.274 687.235 341.874 704.035 y
+307.473 716.835 283.473 691.235 280.273 690.435 c
+277.073 689.635 272.273 686.435 271.473 692.835 c
+270.673 699.235 264.214 714.595 231.473 689.635 c
+201.582 667.071 190.255 684.707 Y
+184.255 685.035 L
+170.654 711.436 179.037 655.962 Y
+187.837 621.562 322.673 669.635 y
+491.474 700.035 502.674 704.035 v
+513.874 708.035 602.093 702.217 y
+597.075 723.09 L
+532.274 769.49 519.474 741.635 505.874 745.635 c
+492.274 749.635 494.674 740.035 491.474 739.235 c
+488.274 738.435 449.074 763.235 442.674 762.435 c
+f
+0.018 0.1 0.154 0 k
+443.983 760.69 m
+437.583 759.89 410.529 782.777 427.183 751.89 c
+449.183 711.09 368.783 712.69 351.983 723.89 c
+335.183 735.09 359.183 705.49 Y
+377.583 685.49 343.183 702.29 y
+308.783 715.09 284.783 689.49 281.583 688.69 c
+278.382 687.89 273.582 684.69 272.782 691.09 c
+271.982 697.49 265.654 712.682 232.782 687.89 c
+201.655 664.58 190.637 681.671 Y
+184.236 683.49 L
+171.236 707.49 179.691 652.907 Y
+188.491 618.507 323.983 667.89 y
+492.783 698.29 503.983 702.29 v
+515.183 706.29 602.529 700.544 y
+597.583 721.926 L
+532.783 768.327 520.783 739.89 507.183 743.89 c
+493.583 747.89 495.983 738.29 492.783 737.49 c
+489.583 736.69 450.383 761.49 443.983 760.69 c
+f
+0.009 0.05 0.077 0 k
+445.292 758.945 m
+438.892 758.145 412.917 781.589 428.492 750.145 c
+449.692 707.344 370.092 710.944 353.292 722.144 c
+336.492 733.344 360.492 703.744 Y
+378.892 683.744 344.492 700.544 y
+310.092 713.344 286.092 687.744 282.892 686.944 c
+279.692 686.144 274.892 682.944 274.092 689.344 c
+273.292 695.744 267.095 710.768 234.092 686.144 c
+201.727 662.089 191.018 678.635 Y
+184.218 681.944 L
+171.418 705.144 180.346 649.853 Y
+189.146 615.453 325.292 666.144 y
+494.093 696.544 505.293 700.544 v
+516.493 704.544 602.965 698.872 y
+598.093 720.763 L
+533.292 767.163 522.093 738.144 508.493 742.144 c
+494.893 746.145 497.293 736.544 494.093 735.744 c
+490.892 734.944 451.692 759.745 445.292 758.945 c
+f
+1 g
+184.2 680.399 m
+171.4 702.4 181 646.799 Y
+189.8 612.399 326.6 664.399 y
+495.401 694.8 506.601 698.8 v
+517.801 702.8 603.401 697.2 y
+598.601 719.6 L
+533.801 766 523.401 736.4 509.801 740.4 c
+496.201 744.4 498.601 734.8 495.401 734 c
+492.201 733.2 453.001 758 446.601 757.2 c
+440.201 756.4 414.981 780.207 429.801 748.4 c
+452.028 700.693 369.041 710.773 354.6 720.4 c
+337.8 731.6 361.8 702 Y
+380.2 681.999 345.8 698.8 y
+311.4 711.6 287.4 685.999 284.2 685.199 c
+281 684.399 276.2 681.199 275.4 687.599 c
+274.6 694 268.535 708.856 235.4 684.399 c
+201.8 659.599 191.4 675.599 Y
+184.2 680.399 L
+f
+0 g
+225.8 650.399 m
+218.6 638.799 239.4 625.599 V
+240.8 624.199 222.8 628.399 V
+216.6 630.399 215 640.799 V
+210.2 645.199 205.4 650.799 v
+200.6 656.399 225.8 650.399 y
+f
+0.8 g
+365.8 698 m
+383.498 671.179 382.9 666.399 v
+381.6 655.999 381.4 646.399 384.6 642.399 c
+387.801 638.399 396.601 605.199 y
+396.201 603.999 408.601 641.999 V
+420.201 657.999 400.201 676.399 V
+365 705.2 365.8 698 v
+f
+0 g
+1 J 0.1 w
+245.8 623.599 m
+257 616.399 242.6 585.199 V
+249 587.599 l
+248.2 576.399 245 573.999 V
+252.2 577.199 l
+257 569.199 253 564.399 V
+269.8 556.399 269 549.999 V
+275.4 557.999 271.4 564.399 v
+267.4 570.799 260.2 566.799 261 585.199 C
+252.2 581.999 l
+257.8 590.799 257.8 597.199 V
+249.8 594.799 l
+265.269 621.377 254.6 622.799 v
+248.6 623.599 245.8 623.599 Y
+f
+0.8 g
+278.2 606.799 m
+281 611.199 278.2 610.399 v
+275.4 609.599 244.2 594.799 238.2 585.199 C
+272.6 609.599 278.2 606.799 V
+f
+288.6 598.799 m
+291.4 603.199 288.6 602.399 v
+285.8 601.599 254.6 586.799 248.6 577.199 C
+283 601.599 288.6 598.799 V
+f
+301.8 613.999 m
+304.6 618.399 301.8 617.599 v
+299 616.799 267.8 601.999 261.8 592.399 C
+296.2 616.799 301.8 613.999 V
+f
+278.6 570.399 m
+278.6 576.399 275.8 575.599 v
+273 574.799 237 557.199 231 547.599 C
+273 573.199 278.6 570.399 V
+f
+279.8 581.199 m
+281 585.999 278.2 585.199 V
+276.2 585.199 249.8 573.599 243.8 563.999 C
+273.4 585.599 279.8 581.199 V
+f
+265.4 533.599 m
+255.4 525.999 l
+265.8 533.599 269.4 532.399 V
+262.6 521.199 261.8 515.999 V
+272.2 528.799 277.8 528.399 V
+285.4 527.999 285.4 517.199 V
+291 527.599 294.2 527.199 V
+295.4 520.799 294.2 513.999 V
+298.2 521.599 302.2 519.999 V
+308.6 521.999 307.8 510.399 V
+307.8 499.999 307 497.199 V
+312.6 523.599 315 523.999 V
+323 525.199 327.8 516.399 V
+323.8 523.999 328.6 521.999 V
+339.4 520.399 342.6 513.599 V
+335.8 525.599 341.4 522.399 V
+348.2 522.399 349.4 515.999 V
+357.8 494.799 359.8 493.199 V
+352.2 514.799 353.8 514.799 V
+351.8 526.799 357 511.999 V
+353.8 525.999 359.4 525.199 v
+365 524.399 369.4 514.399 377.8 516.799 C
+387.401 511.199 389.401 580.399 V
+265.4 533.599 L
+f
+0 g
+0 J 1 w
+270.2 626.399 m
+285 632.399 325 626.399 V
+332.2 625.999 339 634.799 v
+345.8 643.599 372.6 650.799 379 648.799 C
+388.601 642.399 l
+389.401 641.199 l
+401.801 630.799 402.201 623.199 v
+402.601 615.599 387.801 567.599 378.2 551.599 c
+368.6 535.599 359 523.199 339.8 525.599 C
+319 529.599 293.4 525.599 v
+264.2 527.199 261.4 535.199 v
+258.6 543.199 272.6 558.399 y
+277 566.799 275.8 581.199 v
+274.6 595.599 275 623.599 270.2 626.399 c
+f
+0.1 0.6 0.45 0 k
+292.2 624.399 m
+300.6 605.999 271 540.799 y
+269 539.199 283.66 533.154 293.8 535.599 c
+304.746 538.237 345 533.999 Y
+368.6 549.599 381.4 593.999 y
+391.801 617.999 374.2 621.199 v
+356.6 624.399 292.2 624.399 y
+f
+0.1 0.6 0.45 0.2 k
+290.169 593.503 m
+293.495 606.293 295.079 618.094 292.2 624.399 c
+354.6 617.999 365.8 638.799 v
+370.041 646.674 384.801 615.999 384.4 606.399 c
+321.4 591.999 306.6 603.199 V
+290.169 593.503 L
+f
+0.1 0.6 0.45 0.25 k
+294.6 577.199 m
+296.6 569.999 294.2 565.999 V
+292.6 565.199 291.4 564.799 V
+292.6 561.199 298.6 559.599 V
+300.6 555.199 303 554.799 v
+305.4 554.399 310.2 548.799 314.2 549.999 c
+318.2 551.199 329.4 555.199 y
+335 558.399 343.8 554.799 V
+346.175 555.601 346.6 559.599 v
+347.1 564.299 350.2 567.999 352.2 569.999 c
+354.2 571.999 363.8 584.799 362.6 585.199 c
+361.4 585.599 294.6 577.199 Y
+f
+0 0.55 0.5 0 k
+290.2 625.599 m
+287.4 603.199 290.6 594.799 v
+293.8 586.399 293 584.399 292.2 580.399 c
+291.4 576.399 295.8 566.399 301.4 560.399 C
+313.4 558.799 l
+328.6 562.399 337.8 559.599 V
+346.794 558.256 350.2 573.199 V
+355 579.599 362.2 582.399 v
+369.4 585.199 376.6 626.799 372.6 634.799 c
+368.6 642.799 354.2 647.199 338.2 631.599 c
+322.2 615.999 320.2 632.799 290.2 625.599 C
+b
+0 0 0.2 0 k
+0.5 w
+291.8 550.799 m
+291 552.799 286.6 553.199 V
+264.2 556.799 255.8 569.199 V
+249 574.799 253.4 563.199 V
+263.8 542.799 270.6 539.999 V
+287 535.999 291.8 550.799 V
+b
+0 0.55 0.5 0.2 k
+1 w
+371.742 614.771 m
+372.401 622.677 374.354 631.291 372.6 634.799 c
+366.154 647.693 349.181 642.305 338.2 631.599 c
+322.2 615.999 320.2 632.799 290.2 625.599 C
+288.455 611.636 289.295 601.624 v
+326.6 613.199 327.4 607.599 V
+329 610.799 338.2 610.799 v
+347.4 610.799 370.142 611.971 371.742 614.771 C
+f
+0 g
+0 0.55 0.5 0.35 K
+2 w
+328.6 624.799 m
+333.4 619.999 329.8 610.399 V
+315.4 594.399 317.4 580.399 v
+S
+0 0 0.2 0 k
+0 G
+0.5 w
+280.6 539.999 m
+276.2 552.799 285 545.999 V
+289.8 543.999 288.6 542.399 v
+287.4 540.799 281.8 536.799 280.6 539.999 C
+b
+285.64 538.799 m
+282.12 549.039 289.16 543.599 V
+293.581 541.151 292.04 540.719 v
+287.48 539.439 292.04 536.879 285.64 538.799 C
+b
+290.44 538.799 m
+286.92 549.039 293.96 543.599 V
+298.335 541.289 296.84 540.719 v
+293.48 539.439 296.84 536.879 290.44 538.799 C
+b
+297.04 538.599 m
+293.52 548.839 300.56 543.399 V
+304.943 541.067 303.441 540.519 v
+300.48 539.439 303.441 536.679 297.04 538.599 C
+b
+303.52 538.679 m
+300 548.919 307.041 543.479 V
+310.881 541.879 309.921 540.599 v
+308.961 539.319 309.921 536.759 303.52 538.679 C
+b
+310.2 537.999 m
+305.4 550.399 314.6 543.999 V
+319.4 541.999 318.2 540.399 v
+317 538.799 318.2 535.599 310.2 537.999 C
+b
+0 g
+0.1 0.6 0.45 0.25 K
+2 w
+281.8 555.199 m
+295 557.999 301 554.799 V
+307 553.599 308.2 553.999 v
+309.4 554.399 312.6 554.799 y
+S
+315.8 546.399 m
+327.8 559.999 339.8 555.599 v
+346.816 553.026 345.8 556.399 346.6 559.199 c
+347.4 561.999 347.6 566.199 352.6 569.199 c
+S
+0 0 0.2 0 k
+0 G
+0.5 w
+333 562.399 m
+329 573.199 326.2 560.399 v
+323.4 547.599 320.2 543.999 318.6 541.199 C
+318.6 535.999 327 536.399 V
+337.8 536.799 338.2 539.599 v
+338.6 542.399 337 553.999 333 562.399 C
+b
+0 g
+0.1 0.6 0.45 0.25 K
+2 w
+347 555.199 m
+350.6 557.599 353 556.399 v
+S
+353.5 571.599 m
+356.4 576.499 361.2 577.299 v
+S
+0.7 g
+0 G
+1 w
+274.2 534.799 m
+292.2 531.599 296.6 533.199 V
+305.4 533.199 297 531.199 V
+284.2 531.199 276.2 532.399 V
+264.6 537.999 274.2 534.799 V
+f
+0 0 0.2 0 k
+0.5 w
+288.2 627.999 m
+305.8 627.999 307.8 627.199 V
+315 596.399 311.4 588.799 V
+310.2 585.999 307.4 591.599 V
+289 624.399 285.8 626.399 v
+282.6 628.399 287 627.999 288.2 627.999 C
+b
+211.1 630.699 m
+220 628.999 232.6 626.399 V
+237.4 603.999 240.6 599.199 v
+243.8 594.399 240.2 594.399 236.6 597.199 c
+233 599.999 218.2 613.999 216.2 618.399 c
+214.2 622.799 211.1 630.699 y
+b
+232.961 626.182 m
+238.761 624.634 239.77 622.419 v
+240.778 620.205 238.568 616.908 y
+237.568 613.603 236.366 615.765 v
+235.164 617.928 232.292 625.588 232.961 626.182 c
+b
+0 g
+233 626.399 m
+236.6 621.199 240.2 621.199 v
+243.8 621.199 244.182 621.612 247 620.999 c
+251.6 619.999 251.2 621.999 257.8 620.799 c
+260.44 620.319 263 621.199 265.8 619.999 c
+268.6 618.799 271.8 619.599 273 621.599 c
+274.2 623.599 279 627.799 Y
+266.2 625.999 263.4 625.199 V
+241 623.999 233 626.399 V
+f
+0 0 0.2 0 k
+277.6 626.199 m
+271.15 622.699 270.75 620.299 v
+270.35 617.899 276 614.199 y
+278.75 609.599 279.35 611.999 v
+279.95 614.399 278.4 625.799 277.6 626.199 c
+b
+240.115 620.735 m
+247.122 609.547 247.339 620.758 V
+247.896 622.016 246.136 622.038 v
+240.061 622.114 241.582 626.216 240.115 620.735 C
+b
+247.293 620.486 m
+255.214 609.299 254.578 620.579 V
+254.585 620.911 252.832 621.064 v
+248.085 621.478 248.43 625.996 247.293 620.486 C
+b
+254.506 620.478 m
+262.466 609.85 261.797 619.516 V
+261.916 620.749 260.262 621.05 v
+256.37 621.756 256.159 625.005 254.506 620.478 C
+b
+261.382 620.398 m
+269.282 608.837 269.63 618.618 V
+271.274 619.996 269.528 620.218 v
+263.71 620.958 264.508 625.412 261.382 620.398 C
+b
+0 0 0.2 0.1 k
+225.208 616.868 m
+217.55 618.399 l
+214.95 623.399 212.85 629.549 y
+219.2 628.549 231.7 625.749 V
+232.576 622.431 234.048 616.636 v
+225.208 616.868 l
+f
+290.276 621.53 m
+288.61 624.036 287.293 625.794 286.643 626.2 c
+283.63 628.083 287.773 627.706 288.902 627.706 C
+305.473 627.706 307.356 626.953 V
+307.88 624.711 308.564 621.32 V
+298.476 623.33 290.276 621.53 V
+f
+0.2 0.55 0.85 0 k
+1 w
+343.88 759.679 m
+371.601 755.719 397.121 791.359 398.881 801.04 c
+400.641 810.72 390.521 822.6 Y
+391.841 825.68 387.001 839.76 381.721 849 c
+376.441 858.24 360.54 857.266 343 858.24 c
+327.16 859.12 308.68 835.8 307.36 834.04 c
+306.04 832.28 312.2 793.999 313.52 788.279 c
+314.84 782.559 312.2 756.159 y
+346.44 765.259 316.16 763.639 343.88 759.679 c
+f
+0.08 0.44 0.68 0 k
+308.088 833.392 m
+306.792 831.664 312.84 794.079 314.136 788.463 c
+315.432 782.847 312.84 756.927 y
+345.512 765.807 316.728 764.271 343.944 760.383 c
+371.161 756.495 396.217 791.487 397.945 800.992 c
+399.673 810.496 389.737 822.16 Y
+391.033 825.184 386.281 839.008 381.097 848.08 c
+375.913 857.152 360.302 856.195 343.08 857.152 c
+327.528 858.016 309.384 835.12 308.088 833.392 c
+f
+0.06 0.33 0.51 0 k
+308.816 832.744 m
+307.544 831.048 313.48 794.159 314.752 788.647 c
+316.024 783.135 313.48 757.695 y
+344.884 766.855 317.296 764.903 344.008 761.087 c
+370.721 757.271 395.313 791.615 397.009 800.944 c
+398.705 810.272 388.953 821.72 Y
+390.225 824.688 385.561 838.256 380.473 847.16 c
+375.385 856.064 360.063 855.125 343.16 856.064 c
+327.896 856.912 310.088 834.44 308.816 832.744 c
+f
+0.04 0.22 0.34 0 k
+309.544 832.096 m
+308.296 830.432 314.12 794.239 315.368 788.831 c
+316.616 783.423 314.12 758.463 y
+343.556 767.503 317.864 765.535 344.072 761.791 c
+370.281 758.047 394.409 791.743 396.073 800.895 c
+397.737 810.048 388.169 821.28 Y
+389.417 824.192 384.841 837.504 379.849 846.24 c
+374.857 854.976 359.824 854.055 343.24 854.976 c
+328.264 855.808 310.792 833.76 309.544 832.096 c
+f
+0.02 0.11 0.17 0 k
+310.272 831.448 m
+309.048 829.816 314.76 794.319 315.984 789.015 c
+317.208 783.711 314.76 759.231 y
+342.628 768.151 318.432 766.167 344.136 762.495 c
+369.841 758.823 393.505 791.871 395.137 800.848 c
+396.769 809.824 387.385 820.84 Y
+388.609 823.696 384.121 836.752 379.225 845.32 c
+374.329 853.888 359.585 852.985 343.32 853.888 c
+328.632 854.704 311.496 833.08 310.272 831.448 c
+f
+1 g
+344.2 763.2 m
+369.4 759.6 392.601 792 394.201 800.8 c
+395.801 809.6 386.601 820.4 Y
+387.801 823.2 383.4 836 378.6 844.4 c
+373.8 852.8 359.346 851.914 343.4 852.8 c
+329 853.6 312.2 832.4 311 830.8 c
+309.8 829.2 315.4 794.4 316.6 789.2 c
+317.8 784 315.4 760 y
+340.9 768.6 319 766.8 344.2 763.2 c
+f
+0.8 g
+390.601 797.2 m
+362.8 789.6 351.2 791.2 V
+335.4 797.8 326.6 776 V
+323 768.8 321 766.8 v
+319 764.8 390.601 797.2 Y
+f
+0 g
+394.401 799.4 m
+365.4 787.2 355.4 787.6 v
+339 792.2 330.6 777.6 V
+322.2 768.4 319 766.8 V
+318.6 765.2 325 769.2 V
+335.4 764 l
+350.2 754.4 359.8 770.4 V
+363.8 781.6 363.8 783.6 v
+363.8 785.6 385 791.2 386.601 791.6 c
+388.201 792 394.801 796.2 394.401 799.4 C
+f
+0.4 0.2 0.8 0 k
+347 763.486 m
+340.128 763.486 331.755 767.351 331.755 773.6 c
+331.755 779.848 340.128 786.113 347 786.113 c
+353.874 786.113 359.446 781.048 359.446 774.8 c
+359.446 768.551 353.874 763.486 347 763.486 c
+f
+0.4 0.2 0.8 0.2 k
+343.377 780.17 m
+338.531 779.448 333.442 777.945 333.514 778.161 c
+335.054 782.78 341.415 786.113 347 786.113 c
+351.296 786.113 355.084 784.135 357.32 781.125 c
+352.004 781.455 343.377 780.17 v
+f
+1 g
+355.4 780.4 m
+351 783.6 351 781.4 V
+354.6 777 355.4 780.4 V
+f
+0 g
+345.4 772.274 m
+342.901 772.274 340.875 774.3 340.875 776.8 c
+340.875 779.299 342.901 781.325 345.4 781.325 c
+347.9 781.325 349.926 779.299 349.926 776.8 c
+349.926 774.3 347.9 772.274 345.4 772.274 c
+f
+0.2 0.55 0.85 0 k
+241.4 785.6 m
+238.2 806.8 240.6 811.2 V
+251.4 821.2 251 824.8 V
+250.6 842.8 249.4 843.6 v
+248.2 844.4 240.6 850.4 234.6 844 C
+224.2 826 225 819.6 V
+225 817.6 l
+217.4 818 215.8 816 V
+214.6 810.8 213.4 810.4 V
+210.6 808 212.6 805.2 V
+210.6 802.8 211 798.8 V
+218.6 794.8 L
+220.6 780.4 231.4 775.2 v
+236.236 772.871 239.4 779.6 241.4 785.6 c
+f
+1 g
+240.4 787.44 m
+237.52 806.52 239.68 810.48 V
+249.4 819.48 249.04 822.72 V
+248.68 838.92 247.6 839.64 v
+246.52 840.36 239.68 845.76 234.28 840 C
+224.92 823.8 225.64 818.04 V
+225.64 816.24 l
+218.8 816.6 217.36 814.8 V
+216.28 810.12 215.2 809.76 V
+212.68 807.6 214.48 805.08 V
+212.68 802.92 213.04 799.32 V
+219.88 795.72 L
+221.68 782.76 231.4 778.08 v
+235.752 775.985 238.6 782.04 240.4 787.44 c
+f
+0.075 0.412 0.637 0 k
+248.95 842.61 m
+247.86 843.47 240.37 849.24 234.52 843 C
+224.38 825.45 225.16 819.21 V
+225.16 817.26 l
+217.75 817.65 216.19 815.7 V
+215.02 810.63 213.85 810.24 V
+211.12 807.9 213.07 805.17 V
+211.12 802.83 211.51 798.93 V
+218.92 795.03 L
+220.87 780.99 231.4 775.92 v
+236.114 773.65 239.2 780.21 241.15 786.06 c
+238.03 806.73 240.37 811.02 V
+250.9 820.77 250.51 824.28 V
+250.12 841.83 248.95 842.61 V
+f
+0.05 0.275 0.425 0 k
+248.5 841.62 m
+247.52 842.54 240.14 848.08 234.44 842 C
+224.56 824.9 225.32 818.82 V
+225.32 816.92 l
+218.1 817.3 216.58 815.4 V
+215.44 810.46 214.3 810.08 V
+211.64 807.8 213.54 805.14 V
+211.64 802.86 212.02 799.06 V
+219.24 795.26 L
+221.14 781.58 231.4 776.64 v
+235.994 774.428 239 780.82 240.9 786.52 c
+237.86 806.66 240.14 810.84 V
+250.4 820.34 250.02 823.76 V
+249.64 840.86 248.5 841.62 V
+f
+0.025 0.137 0.212 0 k
+248.05 840.63 m
+247.18 841.61 239.91 846.92 234.36 841 C
+224.74 824.35 225.48 818.43 V
+225.48 816.58 l
+218.45 816.95 216.97 815.1 V
+215.86 810.29 214.75 809.92 V
+212.16 807.7 214.01 805.11 V
+212.16 802.89 212.53 799.19 V
+219.56 795.49 L
+221.41 782.17 231.4 777.36 v
+235.873 775.206 238.8 781.43 240.65 786.98 c
+237.69 806.59 239.91 810.66 V
+249.9 819.91 249.53 823.24 V
+249.16 839.89 248.05 840.63 V
+f
+1 g
+240.4 787.54 m
+237.52 806.52 239.68 810.48 V
+249.4 819.48 249.04 822.72 V
+248.68 838.92 247.6 839.64 V
+246.84 840.68 239.68 845.76 234.28 840 C
+224.92 823.8 225.64 818.04 V
+225.64 816.24 l
+218.8 816.6 217.36 814.8 V
+216.28 810.12 215.2 809.76 V
+212.68 807.6 214.48 805.08 V
+212.68 802.92 213.04 799.32 V
+219.88 795.72 L
+221.68 782.76 231.4 778.08 v
+235.752 775.985 238.6 782.14 240.4 787.54 c
+f
+0.8 g
+237.3 793.8 m
+215.7 804 214.8 804.8 V
+223.9 796.6 224.7 796.6 v
+225.5 796.6 237.3 793.8 Y
+f
+0 g
+220.2 800 m
+238.6 796.4 238.6 792 v
+238.6 789.088 238.357 775.669 233 777.2 c
+224.6 779.6 228.2 794 220.2 800 c
+f
+0.4 0.2 0.8 0 k
+228.6 796.2 m
+237.578 794.726 238.6 792 v
+239.2 790.4 239.863 782.092 234.4 781 c
+229.848 780.089 227.618 790.31 228.6 796.2 c
+f
+0 g
+314.595 753.651 m
+314.098 755.393 315.409 755.262 317.2 755.8 c
+319.2 756.4 331.4 760.2 332.2 762.8 c
+333 765.4 346.2 761 Y
+348 760.2 352.4 757.6 Y
+357.2 756.4 363.8 756 Y
+366.2 755 369.6 752.2 Y
+384.2 742 396.601 749.2 Y
+416.601 755.8 410.601 773 Y
+407.601 782 410.801 785.4 Y
+411.001 789.2 418.201 782.8 Y
+420.801 778.6 421.601 773.6 Y
+429.601 762.4 426.201 780.2 Y
+426.401 781.2 423.601 784.8 423.601 786 c
+423.601 787.2 421.801 790.6 Y
+418.801 794 421.201 801 Y
+423.001 814.8 420.801 813 Y
+419.601 814.8 410.401 804.8 Y
+408.201 801.4 402.201 799.8 Y
+399.401 798 396.001 799.4 Y
+393.401 799.8 387.801 792.8 Y
+390.601 793 393.001 788.6 395.401 788.4 c
+397.801 788.2 399.601 790.8 401.201 791.4 c
+402.801 792 405.601 786.2 Y
+406.001 783.6 400.401 778.8 Y
+400.001 774.2 398.401 775.8 Y
+395.401 776.4 394.201 772.6 393.201 768 c
+392.201 763.4 388.001 763 y
+386.401 755.6 385.2 758.6 Y
+385 764.2 379 758.4 Y
+377.8 756.4 373.2 758.6 Y
+366.4 760.6 368.8 762.6 Y
+370.6 764.8 381.8 762.6 Y
+384 764.2 376 768.2 Y
+375.4 770 376.4 774.4 Y
+377.6 777.6 384.4 783.2 Y
+393.801 784.4 391.001 786 Y
+384.801 791.2 379 783.6 Y
+376.8 777.4 359.4 762.4 Y
+354.6 759 357.2 765.8 353.2 762.4 c
+349.2 759 328.6 768 y
+317.038 769.193 314.306 753.451 310.777 756.571 c
+316.195 748.051 314.595 753.651 v
+f
+509.401 920 m
+483.801 912 481.001 893.2 V
+478.601 870.4 499.001 852.8 V
+499.401 846.4 501.401 843.2 v
+499.801 838.4 518.601 846 V
+545.801 854.4 l
+552.201 856.8 557.401 865.6 v
+562.601 874.4 577.801 893.2 574.201 918.4 C
+575.401 929.6 569.401 930 V
+561.001 931.6 553.801 924 V
+547.001 920.8 544.601 921.2 V
+509.401 920 L
+f
+564.022 920.99 m
+566.122 929.92 561.282 925.08 V
+554.242 919.36 546.761 919.36 V
+532.241 917.16 527.841 903.96 V
+523.881 877.12 531.801 871.4 V
+536.641 863.92 543.681 870.52 v
+550.722 877.12 566.222 907.35 564.022 920.99 C
+f
+0.2 g
+563.648 920.632 m
+565.738 929.376 560.986 924.624 V
+554.074 919.008 546.729 919.008 V
+532.473 916.848 528.153 903.888 V
+524.265 877.536 532.041 871.92 V
+536.793 864.576 543.705 871.056 v
+550.618 877.536 565.808 907.24 563.648 920.632 C
+f
+0.4 g
+563.274 920.274 m
+565.354 928.832 560.69 924.168 V
+553.906 918.656 546.697 918.656 V
+532.705 916.536 528.465 903.816 V
+524.649 877.952 532.281 872.44 V
+536.945 865.232 543.729 871.592 v
+550.514 877.952 565.394 907.13 563.274 920.274 C
+f
+0.6 g
+562.9 919.916 m
+564.97 928.288 560.394 923.712 V
+553.738 918.304 546.665 918.304 V
+532.937 916.224 528.777 903.744 V
+525.033 878.368 532.521 872.96 V
+537.097 865.888 543.753 872.128 v
+550.41 878.368 564.98 907.02 562.9 919.916 C
+f
+0.8 g
+562.526 919.558 m
+564.586 927.744 560.098 923.256 V
+553.569 917.952 546.633 917.952 V
+533.169 915.912 529.089 903.672 V
+525.417 878.784 532.761 873.48 V
+537.249 866.544 543.777 872.664 v
+550.305 878.784 564.566 906.91 562.526 919.558 C
+f
+1 g
+562.151 919.2 m
+564.201 927.2 559.801 922.8 V
+553.401 917.6 546.601 917.6 V
+533.401 915.6 529.401 903.6 V
+525.801 879.2 533.001 874 V
+537.401 867.2 543.801 873.2 v
+550.201 879.2 564.151 906.8 562.151 919.2 C
+f
+0.1 0.55 0.85 0.3 k
+350.6 716 m
+330.2 735.2 322.2 736 V
+287.8 740 273 722 V
+290.6 742.4 318.2 736.8 V
+296.6 741.2 284.2 738 V
+267.4 738 257.8 724 V
+255 719.2 l
+259 734 277.4 740 V
+300.2 744.8 311 740 V
+289.4 746.8 279.4 744.8 V
+249 747.2 236.2 720.8 V
+240.2 735.2 255 742.4 V
+268.6 751.2 289 748.4 V
+303.4 745.2 308.6 742.8 v
+313.8 740.4 312.6 743.2 304.2 748 C
+298.6 758 284.6 757.6 V
+241.8 754 231.4 742 V
+245 753.2 255.4 756 V
+277.8 764 286.2 763.2 V
+311 762.2 318.6 766.2 V
+307.4 761.2 310.6 758 v
+313.8 754.8 320.6 747.2 320.6 746 c
+320.6 744.8 344.8 722.7 348.4 718.3 C
+350.6 716 l
+f
+0.8 g
+1 J 0.1 w
+489 522 m
+473.5 558.5 461 568 V
+487 552 490.5 534 V
+490.5 524 489 522 V
+f
+536 514.5 m
+509.5 569.5 491 593.5 V
+534.5 556 539.5 529.5 V
+540 524 l
+537 526.5 l
+536.5 517.5 536 514.5 V
+f
+592.5 563 m
+530 622.5 528.5 625 V
+589 559 592 551.5 V
+590 560.5 592.5 563 V
+f
+404 519.5 m
+423.5 571.5 442.5 549 V
+457.5 539 457 536 V
+453 542.5 435 542 V
+416 545 404 519.5 V
+f
+594.5 647 m
+549.5 675.5 542 677 v
+530.193 679.361 591.5 648 596.5 637.5 C
+598.5 640 594.5 647 V
+f
+0 g
+0 J 1 w
+443.801 540.399 m
+464.201 542.399 471.001 549.199 V
+475.401 545.599 l
+493.001 583.999 l
+496.601 578.799 l
+511.001 593.599 510.201 601.599 v
+509.401 609.599 523.001 595.599 y
+522.201 607.199 529.401 600.399 V
+527.001 615.999 535.401 607.999 V
+524.864 638.156 547.401 612.399 v
+553.001 605.999 548.601 612.799 y
+522.601 660.799 544.201 646.399 v
+546.201 669.199 545.001 673.599 v
+543.801 677.999 541.801 700.4 537.001 705.6 c
+532.201 710.8 537.401 712.4 543.001 707.2 C
+531.801 731.2 545.001 719.2 V
+541.401 734.4 537.001 737.2 V
+531.401 754.4 546.601 743.6 V
+542.201 756 539.001 759.2 V
+527.401 786.8 534.601 782 V
+539.001 778.4 l
+532.201 792.4 538.601 788 v
+545.001 783.6 545.001 784 y
+523.801 817.2 544.201 799.6 V
+536.042 813.518 532.601 820.4 V
+513.801 840.8 528.201 834.4 V
+533.001 832.8 l
+524.201 842.8 516.201 844.4 v
+508.201 846 518.601 852.4 525.001 850.4 c
+531.401 848.4 547.001 840.8 y
+559.801 822 563.801 821.6 V
+543.801 829.2 549.801 821.2 V
+564.201 807.2 557.001 807.6 V
+551.001 800.4 555.801 791.6 V
+537.342 809.991 552.201 784.4 v
+559.001 768 l
+534.601 792.8 545.801 770.8 V
+563.001 747.2 565.001 746.8 v
+567.001 746.4 571.401 737.6 y
+567.001 739.6 l
+572.201 730.8 l
+561.001 742.8 567.001 729.6 V
+572.601 715.2 l
+552.201 737.2 565.801 707.6 V
+549.401 712.8 558.201 695.6 V
+556.601 679.599 557.001 674.399 v
+557.401 669.199 558.601 640.799 554.201 632.799 c
+549.801 624.799 560.201 605.599 562.201 601.599 c
+564.201 597.599 567.801 586.799 559.001 595.999 c
+550.201 605.199 554.601 599.599 556.601 590.799 c
+558.601 581.999 564.601 566.399 563.801 560.799 C
+562.601 559.599 559.401 563.199 V
+544.601 585.999 546.201 571.599 V
+545.001 563.599 541.801 554.799 V
+538.601 543.999 538.601 552.799 V
+535.401 569.599 532.601 561.999 v
+529.801 554.399 526.201 548.399 523.401 545.999 c
+520.601 543.599 515.401 566.399 514.201 555.999 C
+502.201 568.399 497.401 551.999 V
+485.801 535.599 l
+485.401 547.999 484.201 541.999 V
+454.201 535.999 443.801 540.399 V
+f
+409.401 897.2 m
+397.801 905.2 393.801 904.8 v
+389.801 904.4 421.401 913.6 462.601 886 C
+467.401 883.2 471.001 883.6 V
+474.201 881.2 471.401 877.6 V
+462.601 868 473.801 856.8 V
+492.201 850 486.601 858.8 V
+497.401 854.8 499.801 850.8 v
+502.201 846.8 501.001 850.8 y
+494.601 858 488.601 863.2 V
+483.401 865.2 480.601 873.6 v
+477.801 882 475.401 892 479.801 895.2 C
+475.801 890.8 476.601 894.8 v
+477.401 898.8 481.001 902.4 482.601 902.8 c
+484.201 903.2 500.601 919 507.401 919.4 C
+498.201 918 495.201 919 v
+492.201 920 465.601 931.4 459.601 932.6 C
+442.801 939.2 454.801 937.2 V
+490.601 933.4 508.801 920.2 V
+501.601 928.6 483.201 935.6 V
+461.001 948.2 425.801 943.2 V
+408.001 940 400.201 938.2 V
+397.601 938.8 397.001 939.2 v
+396.401 939.6 384.6 948.6 357 941.6 C
+340 937 331.4 932.2 V
+316.2 931 312.6 927.8 V
+294 913.2 292 912.4 v
+290 911.6 278.6 904 277.8 903.6 C
+302.4 910.2 304.8 912.6 v
+307.2 915 324.6 917.6 327 916.2 c
+329.4 914.8 337.8 915.4 328.2 914.8 C
+403.801 900 404.601 898 v
+405.401 896 409.401 897.2 y
+f
+0.2 0.55 0.85 0 k
+480.801 906.4 m
+470.601 913.8 468.601 913.8 v
+466.601 913.8 454.201 924 450.001 923.6 c
+445.801 923.2 433.601 933.2 406.201 925 C
+405.601 927 409.201 927.8 V
+415.601 930 416.001 930.6 V
+436.201 934.8 443.401 931.2 V
+452.601 928.6 458.801 922.4 V
+470.001 919.2 473.201 920.2 V
+482.001 918 482.401 916.2 V
+488.201 913.2 486.401 910.6 V
+486.801 909 480.801 906.4 V
+f
+468.33 908.509 m
+469.137 907.877 470.156 907.779 470.761 906.97 c
+470.995 906.656 470.706 906.33 470.391 906.233 c
+469.348 905.916 468.292 906.486 467.15 905.898 c
+466.748 905.691 466.106 905.873 465.553 906.022 c
+463.921 906.463 462.092 906.488 460.401 905.8 C
+458.416 906.929 456.056 906.345 453.975 907.346 c
+453.917 907.373 453.695 907.027 453.621 907.054 c
+450.575 908.199 446.832 907.916 444.401 910.2 C
+441.973 910.612 439.616 911.074 437.188 911.754 c
+435.37 912.263 433.961 913.252 432.341 914.084 c
+430.964 914.792 429.507 915.314 427.973 915.686 c
+426.11 916.138 424.279 916.026 422.386 916.546 c
+422.293 916.571 422.101 916.227 422.019 916.254 c
+421.695 916.362 421.405 916.945 421.234 916.892 c
+419.553 916.37 418.065 917.342 416.401 917 C
+415.223 918.224 413.495 917.979 411.949 918.421 c
+408.985 919.269 405.831 917.999 402.801 919 C
+406.914 920.842 411.601 919.61 415.663 921.679 c
+417.991 922.865 420.653 921.763 423.223 922.523 c
+423.71 922.667 424.401 922.869 424.801 922.2 C
+424.935 922.335 425.117 922.574 425.175 922.546 c
+427.625 921.389 429.94 920.115 432.422 919.049 c
+432.763 918.903 433.295 919.135 433.547 918.933 c
+435.067 917.717 437.01 917.82 438.401 916.6 C
+440.099 917.102 441.892 916.722 443.621 917.346 c
+443.698 917.373 443.932 917.032 443.965 917.054 c
+445.095 917.802 446.25 917.531 447.142 917.227 c
+447.48 917.112 448.143 916.865 448.448 916.791 c
+449.574 916.515 450.43 916.035 451.609 915.852 c
+451.723 915.834 451.908 916.174 451.98 916.146 c
+453.103 915.708 454.145 915.764 454.801 914.6 C
+454.936 914.735 455.101 914.973 455.183 914.946 c
+456.21 914.608 456.859 913.853 457.96 913.612 c
+458.445 913.506 459.057 912.88 459.633 912.704 c
+462.025 911.973 463.868 910.444 466.062 909.549 c
+466.821 909.239 467.697 909.005 468.33 908.509 c
+f
+391.696 922.739 m
+389.178 924.464 386.81 925.57 384.368 927.356 c
+384.187 927.489 383.827 927.319 383.625 927.441 c
+382.618 928.05 381.73 928.631 380.748 929.327 c
+380.209 929.709 379.388 929.698 378.88 929.956 c
+376.336 931.248 373.707 931.806 371.2 933 C
+371.882 933.638 373.004 933.394 373.6 934.2 C
+373.795 933.92 374.033 933.636 374.386 933.827 c
+376.064 934.731 377.914 934.884 379.59 934.794 c
+381.294 934.702 383.014 934.397 384.789 934.125 c
+385.096 934.078 385.295 933.555 385.618 933.458 c
+387.846 932.795 390.235 933.32 392.354 932.482 c
+393.945 931.853 395.515 931.03 396.754 929.755 c
+397.006 929.495 396.681 929.194 396.401 929 C
+396.789 929.109 397.062 928.903 397.173 928.59 c
+397.257 928.351 397.257 928.049 397.173 927.81 c
+397.061 927.498 396.782 927.397 396.408 927.346 c
+395.001 927.156 396.773 928.536 396.073 928.088 c
+394.8 927.274 395.546 925.868 394.801 924.6 C
+394.521 924.794 394.291 925.012 394.401 925.4 C
+394.635 924.878 394.033 924.588 393.865 924.272 c
+393.48 923.547 392.581 922.132 391.696 922.739 c
+f
+359.198 915.391 m
+356.044 916.185 352.994 916.07 349.978 917.346 c
+349.911 917.374 349.688 917.027 349.624 917.054 c
+348.258 917.648 347.34 918.614 346.264 919.66 c
+345.351 920.548 343.693 920.161 342.419 920.648 c
+342.095 920.772 341.892 921.284 341.591 921.323 c
+340.372 921.48 339.445 922.429 338.4 923 C
+340.736 923.795 343.147 923.764 345.609 924.148 c
+345.722 924.166 345.867 923.845 346 923.845 c
+346.136 923.845 346.266 924.066 346.4 924.2 C
+346.595 923.92 346.897 923.594 347.154 923.848 c
+347.702 924.388 348.258 924.198 348.798 924.158 c
+348.942 924.148 349.067 923.845 349.2 923.845 c
+349.336 923.845 349.467 924.156 349.6 924.156 c
+349.736 924.155 349.867 923.845 350 923.845 c
+350.136 923.845 350.266 924.066 350.4 924.2 C
+351.092 923.418 351.977 923.972 352.799 923.793 c
+353.837 923.566 354.104 922.418 355.178 922.12 c
+359.893 920.816 364.03 918.671 368.393 916.584 c
+368.7 916.437 368.91 916.189 368.8 915.8 C
+369.067 915.8 369.38 915.888 369.57 915.756 c
+370.628 915.024 371.669 914.476 372.366 913.378 c
+372.582 913.039 372.253 912.632 372.02 912.684 c
+367.591 913.679 363.585 914.287 359.198 915.391 c
+f
+345.338 871.179 m
+343.746 872.398 343.162 874.429 342.034 876.221 c
+341.82 876.561 342.094 876.875 342.411 876.964 c
+342.971 877.123 343.514 876.645 343.923 876.443 c
+345.668 875.581 347.203 874.339 349.2 874.2 C
+351.19 871.966 355.45 871.581 355.457 868.2 c
+355.458 867.341 354.03 868.259 353.6 867.4 C
+351.149 868.403 348.76 868.3 346.38 869.767 c
+345.763 870.148 346.093 870.601 345.338 871.179 c
+f
+317.8 923.756 m
+317.935 923.755 324.966 923.522 324.949 923.408 c
+324.904 923.099 317.174 922.05 316.81 922.22 c
+316.646 922.296 309.134 919.866 309 920 C
+309.268 920.135 317.534 923.756 317.8 923.756 c
+f
+0 g
+333.2 914 m
+318.4 912.2 314 911 v
+309.6 909.8 291 902.2 288 900.2 C
+274.6 894.8 257.6 874.8 V
+265.2 878.2 267.4 881 V
+281 893.6 280.8 891 V
+293 899.6 292.4 897.4 V
+316.8 908.6 314.8 905.4 V
+336.4 910 335.4 908 V
+354.2 903.6 351.4 903.4 V
+345.6 902.2 352 898.6 V
+348.6 894.2 343.2 898.2 v
+337.8 902.2 340.8 900 335.8 899 C
+333.2 898.2 328.6 902.2 V
+323 906.8 314.2 903.2 V
+283.6 890.6 281.6 890 V
+278 887.2 275.6 883.6 V
+269.8 879.2 266.8 877.8 V
+254 866.2 252.8 864.8 V
+249.4 859.6 248.6 859.2 V
+255 863 257 865 V
+271 875 276.4 875.8 V
+280.8 878.8 281.6 880.2 V
+296 889.4 300.2 889.4 V
+309.4 884.2 311.8 891.2 V
+317.6 893 323.2 891.8 V
+326.4 894.4 325.6 896.6 V
+327.2 898.4 328.2 894.6 V
+331.6 891 336.4 893 V
+340.4 893.2 338.4 890.8 V
+334 887 322.2 886.8 V
+309.8 886.2 293.4 878.6 V
+263.6 868.2 254.4 857.8 V
+248 849 242.6 847.8 V
+236.8 847 230.8 839.6 V
+240.6 845.4 249.6 845.4 V
+253.6 847.8 249.8 844.2 V
+246.2 836.6 247.8 831.2 V
+247.2 826 246.4 824.4 V
+238.6 811.6 238.6 809.2 v
+238.6 806.8 239.8 797 240.2 796.4 c
+240.6 795.8 239.2 798 243 795.6 c
+246.8 793.2 249.6 791.6 250.4 788.8 c
+251.2 786 248.4 794.2 248.2 796 c
+248 797.8 243.8 805 244.6 807.4 C
+245.6 806.4 246.4 805 V
+245.8 805.6 246.4 809.2 V
+247.2 814.4 248.6 817.6 v
+250 820.8 252 824.6 252.4 825.4 c
+252.8 826.2 252.8 832 254.2 829.4 C
+257.6 826.8 l
+254.8 829.4 257 831.6 V
+256 837.2 257.8 839.8 V
+264.8 848.2 266.4 849.2 v
+268 850.2 266.6 849.8 y
+272.6 854 266.8 852.4 V
+262.8 850.8 259.8 850.8 V
+252.2 848.8 256.2 853 v
+260.2 857.2 270.2 862.6 274 862.4 C
+274.8 860.8 l
+286 863.2 l
+284.8 862.4 l
+284.6 862.6 288.8 863 v
+293 863.4 298.8 862 300.2 863.8 c
+301.6 865.6 305 866.6 304.6 865.2 c
+304.2 863.8 304 861.8 y
+309 867.6 308.4 865.4 v
+307.8 863.2 299.6 858 298.2 851.8 C
+308.6 860 l
+312.2 863 l
+315.8 860.8 316 862.4 v
+316.2 864 320.8 869.8 322 869.6 c
+323.2 869.4 325.2 872.2 325 869.6 c
+324.8 867 332.4 861.6 y
+335.6 863.4 337 862 v
+338.4 860.6 342.6 881.8 y
+367.6 892.4 l
+411.201 895.8 l
+394.201 902.6 l
+333.2 914 l
+f
+0.2 0.55 0.85 0.5 K
+1 J 2 w
+351.4 715 m
+336.4 731.8 328 734.4 V
+314.6 741.2 290 733.4 v
+S
+324.8 735.8 m
+299.6 743.8 284.2 739.6 V
+265.8 737.6 257.4 723.8 v
+S
+321.2 737 m
+304.2 744.2 289.4 746.4 V
+272.8 749 256.2 741.8 V
+244 735.8 238.6 725.6 v
+S
+322.2 736.6 m
+306.8 747.6 305.8 749 V
+298.8 760 285.8 760.4 V
+264.4 759.6 247.2 751.6 v
+S
+0 G
+0 J 1 w
+320.895 745.593 m
+322.437 744.13 349.4 715.2 Y
+384.6 678.599 356.6 712.8 Y
+349 717.6 339.8 736.4 Y
+338.6 739.2 353.8 729.2 Y
+357.8 728.4 371.4 709.2 Y
+364.6 711.6 369.4 704.4 Y
+372.2 702.4 392.601 686.799 Y
+396.201 682.799 400.201 681.199 Y
+414.201 686.399 407.801 673.199 Y
+410.201 666.399 415.801 677.999 Y
+427.001 694.8 410.601 692.399 Y
+380.6 689.599 373.8 705.6 Y
+371.4 708 380.2 705.6 Y
+388.601 703.6 373 718 Y
+375.4 718 384.6 711.2 Y
+395.001 702 397.001 704 Y
+415.001 712.8 425.401 705.2 Y
+427.401 703.6 421.801 696.8 423.401 691.599 c
+425.001 686.399 429.801 673.999 Y
+427.401 672.399 427.801 661.599 Y
+444.601 638.399 435.001 640.399 Y
+419.401 640.799 434.201 633.199 Y
+437.401 631.199 446.201 623.999 Y
+443.401 625.199 441.801 619.999 Y
+446.601 615.999 443.801 611.199 Y
+437.801 609.999 436.601 605.999 Y
+443.401 597.999 433.401 597.599 Y
+437.001 593.199 432.201 581.199 Y
+427.401 581.199 421.001 575.599 Y
+423.401 570.799 413.001 565.199 Y
+404.601 563.599 407.401 556.799 Y
+399.401 550.799 397.001 534.799 Y
+396.201 524.399 393.801 521.199 399.001 523.199 c
+404.201 525.199 403.401 537.599 Y
+398.601 553.199 441.401 569.199 Y
+445.401 570.799 446.201 575.999 Y
+448.201 575.599 457.001 567.999 Y
+464.601 556.799 465.001 565.999 Y
+466.201 569.599 464.601 575.599 Y
+470.601 597.199 456.601 603.599 Y
+446.601 637.199 460.601 628.799 Y
+463.401 623.199 474.201 617.999 y
+477.801 620.399 L
+476.201 625.199 484.601 631.199 Y
+487.401 624.799 493.401 632.799 Y
+497.001 657.199 509.401 642.799 Y
+513.401 641.599 514.601 648.399 Y
+518.201 658.799 514.601 672.399 Y
+518.201 672.799 527.801 666.799 Y
+530.601 670.399 521.401 687.199 525.401 684.799 c
+529.401 682.399 533.801 680.799 Y
+534.601 682.799 524.601 695.199 Y
+520.201 698 515.001 718.4 Y
+522.201 714.8 512.201 730 Y
+512.201 733.2 518.201 744.4 Y
+517.401 751.2 518.201 750.8 Y
+521.001 749.6 529.001 748 522.201 754.4 c
+515.401 760.8 523.001 765.6 Y
+527.401 768.4 513.801 768 Y
+508.601 772.4 509.001 776.4 Y
+517.001 774.4 502.601 788.8 500.201 792.4 c
+497.801 796 507.401 801.2 Y
+520.601 804.8 509.001 808 Y
+489.401 807.6 500.201 818.4 Y
+506.201 818 504.601 820.4 Y
+499.401 821.6 489.801 828 Y
+485.801 831.6 489.401 830.8 Y
+506.201 829.6 477.401 840.8 Y
+485.401 840.8 467.401 851.2 Y
+465.401 852.8 462.201 860.4 Y
+456.201 865.6 451.401 872.4 Y
+451.001 876.8 446.201 881.6 Y
+434.601 895.2 429.001 894.8 Y
+414.201 898.4 409.001 897.6 Y
+356.2 893.2 l
+329.8 880.4 337.6 859.4 Y
+344 851 353.2 854.8 Y
+357.8 861 369.4 858.8 Y
+389.801 855.6 387.201 859.2 Y
+384.801 863.8 368.6 870 368.4 870.6 c
+368.2 871.2 359.4 874.6 Y
+356.4 875.8 352 885 Y
+348.8 888.4 364.6 882.6 Y
+363.4 881.6 370.8 877.6 Y
+388.201 878.6 398.801 867.8 Y
+409.601 851.2 409.801 859.4 Y
+412.601 868.8 400.801 890 Y
+401.201 892 409.401 885.4 Y
+410.801 887.4 411.601 881.6 Y
+411.801 879.2 415.601 871.2 Y
+418.401 858.2 422.001 865.6 Y
+426.601 856.2 L
+428.001 853.6 422.001 846 Y
+421.801 843.2 422.601 843.4 417.001 835.8 c
+411.401 828.2 414.801 823.8 Y
+413.401 817.2 422.201 817.6 Y
+424.801 815.4 428.201 815.4 Y
+430.001 813.4 432.401 814 Y
+434.001 817.8 440.201 815.8 Y
+441.601 818.2 449.801 818.6 Y
+450.801 821.2 451.201 822.8 454.601 823.4 c
+458.001 824 433.401 867 Y
+439.801 867.8 431.601 880.2 Y
+429.401 886.8 440.801 872.2 443.001 870.8 c
+445.201 869.4 446.201 867.2 444.601 867.4 c
+443.001 867.6 441.201 865.4 442.601 865.2 c
+444.001 865 457.001 850 460.401 839.8 c
+463.801 829.6 469.801 825.6 476.001 819.6 c
+482.201 813.6 481.401 789.4 Y
+481.001 780.6 487.001 770 Y
+489.001 766.2 484.801 748 Y
+482.801 745.8 484.201 745 Y
+485.201 743.8 492.001 730.6 Y
+490.201 730.8 493.801 727.2 Y
+499.001 721.2 492.601 724.2 Y
+486.601 725.8 493.601 716 Y
+494.801 714.2 485.801 718.8 Y
+476.601 719.4 488.201 712.2 Y
+496.801 705 485.401 709.4 Y
+480.801 711.2 484.001 704.4 Y
+487.201 702.8 504.401 695.8 Y
+504.801 691.999 501.801 686.999 Y
+502.201 682.999 500.001 679.599 Y
+498.801 671.399 498.201 670.599 Y
+494.001 670.399 486.601 656.599 Y
+484.801 653.999 474.601 641.999 Y
+472.601 634.999 454.601 642.199 Y
+448.001 638.799 450.001 642.199 Y
+449.601 644.399 454.401 650.399 Y
+461.401 652.999 458.801 663.799 Y
+462.801 665.199 451.601 667.999 451.801 669.199 c
+452.001 670.399 457.801 671.799 Y
+465.801 673.799 461.401 676.199 Y
+460.801 680.199 463.801 685.799 Y
+475.401 686.599 463.801 702.8 Y
+453.001 710.4 452.001 716.2 Y
+464.601 724.4 456.401 736.8 456.601 740.4 c
+456.801 744 458.001 765.6 Y
+456.001 771.8 453.001 785.4 Y
+455.201 790.6 462.601 803.2 Y
+465.401 807.4 474.201 812.2 472.001 815.2 c
+469.801 818.2 462.001 816.4 Y
+454.201 817.8 454.801 812.6 Y
+453.201 811.6 452.401 806.6 Y
+451.68 798.667 442.801 792.4 Y
+431.601 786.2 440.801 782.2 Y
+446.801 775.6 437.001 775.4 Y
+426.001 777.2 434.201 767 Y
+445.001 754.2 442.001 751.4 Y
+431.801 750.4 444.401 741.2 y
+443.601 743.2 443.801 741.4 v
+444.001 739.6 447.001 735.4 447.801 733.4 c
+448.601 731.4 444.601 731.2 Y
+445.201 721.6 429.801 725.8 y
+429.801 725.8 428.201 725.6 v
+426.601 725.4 415.401 726.2 409.601 728.4 c
+403.801 730.6 397.001 730.6 y
+393.001 728.8 385.4 729 v
+377.8 729.2 369.8 726.4 Y
+365.4 726.8 374 731.2 374.2 731 c
+374.4 730.8 380 736.4 372 735.8 c
+350.203 734.165 339.4 744.4 Y
+337.4 745.8 334.8 748.6 Y
+324.8 750.6 336.2 736.2 Y
+337.4 734.8 336 733.8 Y
+335.2 735.4 327.4 740.8 Y
+324.589 741.773 323.226 743.107 320.895 745.593 C
+f
+0.2 0.55 0.85 0.5 k
+1 J 2 w
+297 757.2 m
+308.6 751.6 311.2 748.8 v
+313.8 746 327.8 734.6 y
+322.4 736.6 319.8 738.4 v
+317.2 740.2 306.4 748.4 y
+302.6 754.4 297 757.2 v
+f
+0.4 0.2 0.8 0 k
+0 J 1 w
+238.991 788.397 m
+239.328 788.545 238.804 791.257 238.6 791.8 c
+237.578 794.526 228.6 796 y
+228.373 794.635 228.318 793.039 228.424 791.401 c
+233.292 785.882 238.991 788.397 v
+f
+0.4 0.2 0.8 0.2 k
+238.991 788.597 m
+238.542 788.439 238.976 791.331 238.8 791.8 c
+237.778 794.526 228.6 796.1 y
+228.373 794.735 228.318 793.139 228.424 791.501 c
+232.692 786.382 238.991 788.597 v
+f
+0 g
+234.6 788.454 m
+233.975 788.454 233.469 789.594 233.469 791 c
+233.469 792.405 233.975 793.545 234.6 793.545 c
+235.225 793.545 235.732 792.405 235.732 791 c
+235.732 789.594 235.225 788.454 234.6 788.454 c
+f
+234.6 791 m
+F
+189 690.399 m
+183.4 680.399 208.2 686.399 V
+222.2 687.599 224.6 689.999 V
+225.8 689.199 234.166 686.266 237 685.599 c
+243.8 683.999 252.2 694 y
+256.8 704.5 259.6 704.5 v
+262.4 704.5 259.2 702.9 y
+252.6 692.799 253 691.199 V
+247.8 671.199 231.8 670.399 V
+215.65 669.449 217 663.599 V
+225.8 665.999 228.2 663.599 V
+239 663.999 231 657.599 V
+224.2 645.999 l
+224.34 642.081 214.2 645.599 v
+204.4 648.999 194.1 661.899 y
+178.15 676.449 189 690.399 V
+f
+0.1 0.4 0.4 0 k
+187.8 686.399 m
+185.8 676.799 222.6 687.199 V
+227 687.199 229.4 686.399 v
+231.8 685.599 243.8 682.799 245.8 683.999 C
+238.6 670.399 227 671.999 V
+213.8 670.399 214.2 665.599 V
+218.2 658.399 223 655.999 V
+225.8 653.599 225.4 650.399 v
+225 647.199 222.2 645.599 220.2 644.799 c
+218.2 643.999 215 647.199 213.4 647.199 c
+211.8 647.199 203.4 653.599 199 658.399 c
+194.6 663.199 186.2 675.199 186.6 677.999 c
+187 680.799 187.8 686.399 Y
+f
+0.1 0.4 0.4 0.2 k
+191 668.949 m
+193.6 664.999 196.8 660.799 199 658.399 c
+203.4 653.599 211.8 647.199 213.4 647.199 c
+215 647.199 218.2 643.999 220.2 644.799 c
+222.2 645.599 225 647.199 225.4 650.399 c
+225.8 653.599 223 655.999 Y
+219.934 657.532 217.194 661.024 215.615 663.347 C
+215.8 660.799 210.6 661.599 v
+205.4 662.399 200.2 665.199 198.6 668.399 c
+197 671.599 194.6 673.999 196.2 670.399 c
+197.8 666.799 200.2 663.199 201.8 662.799 c
+203.4 662.399 203 661.199 200.6 661.599 c
+198.2 661.999 195.4 662.399 191 667.599 c
+F
+0.1 0.55 0.85 0.3 k
+188.4 689.999 m
+190.2 703.6 191.4 707.6 V
+190.6 714.4 193 718.6 v
+195.4 722.8 197.4 729 200.4 734.4 c
+203.4 739.8 203.6 743.8 207.6 745.4 c
+211.6 747 217.6 755.6 220.4 756.6 c
+223.2 757.6 223 756.8 y
+229.8 771.6 243.4 767.6 V
+227.2 770.4 243 779.8 V
+238.2 778.7 241.5 785.7 v
+243.701 790.368 243.2 783.6 232.2 771.8 C
+227.2 763.2 222 760.2 v
+216.8 757.2 204.8 750.2 203.6 746.4 c
+202.4 742.6 199.2 736.8 197.2 735.2 c
+195.2 733.6 192.4 729.4 192 726 C
+190.8 722 189.4 720.8 v
+188 719.6 187.8 716.4 187.8 714.4 c
+187.8 712.4 185.8 709.6 186 707.2 C
+186.8 688.199 186.4 686.199 V
+188.4 689.999 L
+f
+1 g
+179.8 685.399 m
+177.8 686.799 173.4 680.799 V
+180.7 647.799 180.7 646.399 V
+181.8 648.499 180.5 655.699 v
+179.2 662.899 178.3 675.599 y
+179.8 685.399 l
+f
+0.1 0.55 0.85 0.3 k
+201.4 746 m
+183.8 742.8 184.2 713.6 V
+183.4 688.799 l
+182.2 714.4 181 716 v
+179.8 717.6 183.8 728.8 180.6 722.8 C
+166.6 708.8 174.6 687.599 V
+176.1 684.299 173.1 688.899 V
+168.5 701.5 169.6 707.9 V
+169.8 710.1 171.7 712.9 V
+180.3 724.6 183 726.9 V
+184.8 741.3 200.2 746.5 V
+205.9 748.8 201.4 746 V
+f
+0 g
+340.8 812.2 m
+341.46 812.554 341.451 813.524 342.031 813.697 c
+343.18 814.041 343.344 815.108 343.862 815.892 c
+344.735 817.211 344.928 818.744 345.51 820.235 c
+345.782 820.935 345.809 821.89 345.496 822.55 c
+344.322 825.031 343.62 827.48 342.178 829.906 c
+341.91 830.356 341.648 831.15 341.447 831.748 c
+340.984 833.132 339.727 834.123 338.867 835.443 c
+338.579 835.884 339.104 836.809 338.388 836.893 c
+337.491 836.998 336.042 837.578 335.809 836.552 c
+335.221 833.965 336.232 831.442 337.2 829 C
+336.418 828.308 336.752 827.387 336.904 826.62 c
+337.614 823.014 336.416 819.662 335.655 816.188 c
+335.632 816.084 335.974 815.886 335.946 815.824 c
+334.724 813.138 333.272 810.693 331.453 808.312 c
+330.695 807.32 329.823 806.404 329.326 805.341 c
+328.958 804.554 328.55 803.588 328.8 802.6 C
+325.365 799.82 323.115 795.975 320.504 792.129 c
+320.042 791.449 320.333 790.24 320.884 789.971 c
+321.697 789.573 322.653 790.597 323.123 791.443 c
+323.512 792.141 323.865 792.791 324.356 793.434 c
+324.489 793.609 324.31 794.028 324.445 794.149 c
+327.078 796.496 328.747 799.432 331.2 801.8 C
+333.15 802.129 334.687 803.127 336.435 804.14 c
+336.743 804.319 337.267 804.07 337.557 804.265 c
+339.31 805.442 339.308 807.478 339.414 809.388 c
+339.464 810.272 339.66 811.589 340.8 812.2 c
+f
+331.959 816.666 m
+332.083 816.743 331.928 817.166 332.037 817.382 c
+332.199 817.706 332.602 817.894 332.764 818.218 c
+332.873 818.434 332.71 818.814 332.846 818.956 c
+335.179 821.403 335.436 824.427 334.4 827.4 C
+335.424 828.02 335.485 829.282 335.06 830.129 c
+334.207 831.829 334.014 833.755 333.039 835.298 c
+332.237 836.567 330.659 837.811 329.288 836.508 c
+328.867 836.108 328.546 835.321 328.824 834.609 c
+328.888 834.446 329.173 834.3 329.146 834.218 c
+329.039 833.894 328.493 833.67 328.487 833.398 c
+328.457 831.902 327.503 830.391 328.133 829.062 c
+328.905 827.433 329.724 825.576 330.4 823.8 C
+329.166 821.684 330.199 819.235 328.446 817.358 c
+328.31 817.212 328.319 816.826 328.441 816.624 c
+328.733 816.138 329.139 815.732 329.625 815.44 c
+329.827 815.319 330.175 815.317 330.375 815.441 c
+330.953 815.803 331.351 816.29 331.959 816.666 c
+f
+394.771 826.977 m
+396.16 825.185 396.45 822.39 394.401 821 C
+394.951 817.691 398.302 819.67 400.401 820.2 C
+400.292 820.588 400.519 820.932 400.802 820.937 c
+401.859 820.952 402.539 821.984 403.601 821.8 C
+404.035 823.357 405.673 824.059 406.317 825.439 c
+408.043 829.134 407.452 833.407 404.868 836.653 c
+404.666 836.907 404.883 837.424 404.759 837.786 c
+404.003 839.997 401.935 840.312 400.001 841 C
+398.824 844.875 398.163 848.906 396.401 852.6 C
+394.787 852.85 394.089 854.589 392.752 855.309 c
+391.419 856.028 390.851 854.449 390.892 853.403 c
+390.899 853.198 391.351 852.974 391.181 852.609 c
+391.105 852.445 390.845 852.334 390.845 852.2 c
+390.846 852.065 391.067 851.934 391.201 851.8 C
+390.283 850.98 388.86 850.503 388.565 849.358 c
+387.611 845.648 390.184 842.523 391.852 839.322 c
+392.443 838.187 391.707 836.916 390.947 835.708 c
+390.509 835.013 390.617 833.886 390.893 833.03 c
+391.645 830.699 393.236 828.96 394.771 826.977 c
+f
+357.611 808.591 m
+356.124 806.74 352.712 804.171 355.629 802.243 c
+355.823 802.114 356.193 802.11 356.366 802.244 c
+358.387 803.809 360.39 804.712 362.826 805.294 c
+362.95 805.323 363.224 804.856 363.593 805.017 c
+365.206 805.72 367.216 805.662 368.4 807 C
+372.167 806.776 375.732 807.892 379.123 809.2 c
+380.284 809.648 381.554 810.207 382.755 810.709 c
+384.131 811.285 385.335 812.213 386.447 813.354 c
+386.58 813.49 386.934 813.4 387.201 813.4 C
+387.161 814.263 388.123 814.39 388.37 815.012 c
+388.462 815.244 388.312 815.64 388.445 815.742 c
+390.583 817.372 391.503 819.39 390.334 821.767 c
+390.049 822.345 389.8 822.963 389.234 823.439 c
+388.149 824.35 387.047 823.496 386 823.8 C
+385.841 823.172 385.112 823.344 384.726 823.146 c
+383.867 822.707 382.534 823.292 381.675 822.854 c
+380.313 822.159 379.072 821.99 377.65 821.613 c
+377.338 821.531 376.56 821.627 376.4 821 C
+376.266 821.134 376.118 821.368 376.012 821.346 c
+374.104 820.95 372.844 820.736 371.543 819.044 c
+371.44 818.911 370.998 819.09 370.839 818.955 c
+369.882 818.147 369.477 816.913 368.376 816.241 c
+368.175 816.118 367.823 816.286 367.629 816.157 c
+366.983 815.726 366.616 815.085 365.974 814.638 c
+365.645 814.409 365.245 814.734 365.277 814.99 c
+365.522 816.937 366.175 818.724 365.6 820.6 C
+367.677 823.12 370.194 825.069 372 827.8 C
+372.015 829.966 372.707 832.112 372.594 834.189 c
+372.584 834.382 372.296 835.115 372.17 835.462 c
+371.858 836.316 372.764 837.382 371.92 838.106 c
+370.516 839.309 369.224 838.433 368.4 837 C
+366.562 836.61 364.496 835.917 362.918 837.151 c
+361.911 837.938 361.333 838.844 360.534 839.9 c
+359.549 841.202 359.884 842.638 359.954 844.202 c
+359.96 844.33 359.645 844.466 359.645 844.6 c
+359.646 844.735 359.866 844.866 360 845 C
+359.294 845.626 359.019 846.684 358 847 C
+358.305 848.092 357.629 848.976 356.758 849.278 c
+354.763 849.969 353.086 848.057 351.194 847.984 c
+350.68 847.965 350.213 849.003 349.564 849.328 c
+349.132 849.544 348.428 849.577 348.066 849.311 c
+347.378 848.807 346.789 848.693 346.031 848.488 c
+344.414 848.052 343.136 846.958 341.656 846.103 c
+340.171 845.246 339.216 843.809 338.136 842.489 c
+337.195 841.337 337.059 838.923 338.479 838.423 c
+340.322 837.773 341.626 840.476 343.592 840.15 c
+343.904 840.099 344.11 839.788 344 839.4 C
+344.389 839.291 344.607 839.52 344.8 839.8 C
+345.658 838.781 346.822 838.444 347.76 837.571 c
+348.73 836.667 350.476 837.085 351.491 836.088 c
+353.02 834.586 352.461 831.905 354.4 830.6 C
+353.814 829.287 353.207 828.01 352.872 826.583 c
+352.59 825.377 353.584 824.18 354.795 824.271 c
+356.053 824.365 356.315 825.124 356.8 826.2 C
+357.067 825.933 357.536 825.636 357.495 825.42 c
+357.038 823.033 356.011 821.04 355.553 818.609 c
+355.494 818.292 355.189 818.09 354.8 818.2 C
+354.332 814.051 350.28 811.657 347.735 808.492 c
+347.332 807.99 347.328 806.741 347.737 806.338 c
+349.14 804.951 351.1 806.497 352.8 807 C
+353.013 808.206 353.872 809.148 355.204 809.092 c
+355.46 809.082 355.695 809.624 356.019 809.754 c
+356.367 809.892 356.869 809.668 357.155 809.866 c
+358.884 811.061 360.292 812.167 362.03 813.356 c
+362.222 813.487 362.566 813.328 362.782 813.436 c
+363.107 813.598 363.294 813.985 363.617 814.17 c
+363.965 814.37 364.207 814.08 364.4 813.8 C
+363.754 813.451 363.75 812.494 363.168 812.292 c
+362.393 812.024 361.832 811.511 361.158 811.064 c
+360.866 810.871 360.207 811.119 360.103 810.94 c
+359.505 809.912 358.321 809.474 357.611 808.591 c
+f
+302.2 858 m
+292.962 860.872 281.8 835.2 V
+279.4 830 277 828 v
+274.6 826 263.4 822.4 261.4 818.4 C
+251 802.4 L
+265.8 818.4 269 820.8 V
+277 829.2 273.8 822.4 V
+259.8 811.6 261 802.4 V
+255.4 788 254.6 786 V
+270.6 818 273 819.2 v
+275.4 820.4 276.6 820.4 275.4 816.8 c
+274.2 813.2 273.8 796.8 271 794.8 C
+279 815.2 278.2 818.4 V
+281.4 822 283.8 816.8 V
+282.6 800.8 l
+287 788.8 l
+284.6 800 286.2 815.6 V
+284.2 826 288.2 820.4 v
+292.2 814.8 301.8 808.8 301.8 804 C
+296.6 821.6 287.4 826.4 V
+283.4 820.4 l
+282.2 822.4 l
+278.6 823.2 283 830 v
+287.4 836.8 287 837.6 y
+293.4 830.4 295 830.4 V
+308.2 838 309.4 813.6 V
+316.2 828 307 834.8 V
+292.2 836.8 293.4 842 V
+300.6 854.4 L
+304.2 859.6 302.6 856.8 y
+F
+282.2 841.6 m
+269.4 841.6 266.2 836.4 V
+259 826.8 l
+276.2 836.8 280.2 838 v
+284.2 839.2 282.2 841.6 Y
+f
+242.2 835.2 m
+240.2 834 239.8 831.2 v
+239.4 828.4 237 828 237.8 825.2 c
+238.6 822.4 240.6 820 240.6 824 c
+240.6 828 242.2 830 243 831.2 c
+243.8 832.4 245.4 836.8 242.2 835.2 c
+f
+233.4 774 m
+225 778 221.8 781.6 v
+218.6 785.2 219.052 780.034 214.2 780.4 c
+208.353 780.841 209.4 796.8 y
+205.4 789.2 l
+204.2 774.8 212.2 777.2 v
+216.107 778.372 217.4 776.8 215.8 776 c
+214.2 775.2 221.4 774.8 218.6 773.2 c
+215.8 771.6 230.2 776.8 227.8 766.4 C
+233.4 774 L
+f
+220.8 759.6 m
+205.4 755.2 201.8 764.8 V
+197 762.4 199.2 759.4 v
+201.4 756.4 202.6 756 y
+208 754.8 207.4 754 v
+206.8 753.2 204.4 749.8 y
+214.6 755.8 220.8 759.6 v
+f
+1 g
+449.201 681.399 m
+448.774 679.265 447.103 678.464 445.201 677.799 C
+443.284 678.757 440.686 681.863 438.801 679.799 C
+438.327 680.279 437.548 680.339 437.204 681.001 c
+436.739 681.899 437.011 682.945 436.669 683.743 c
+436.124 685.015 435.415 686.381 435.601 687.799 C
+437.407 688.511 438.002 690.417 437.528 692.18 c
+437.459 692.437 437.03 692.634 437.23 692.983 c
+437.416 693.306 437.734 693.533 438.001 693.8 C
+437.866 693.665 437.721 693.432 437.61 693.452 c
+437 693.558 437.124 694.195 437.254 694.582 c
+437.839 696.328 439.853 696.592 441.201 695.4 C
+441.457 695.965 441.966 695.771 442.401 695.8 C
+442.351 696.379 442.759 696.906 442.957 697.326 c
+443.475 698.424 445.104 697.318 445.901 697.93 c
+446.977 698.755 448.04 699.454 449.118 698.851 c
+450.927 697.838 452.636 696.626 453.835 694.885 c
+454.41 694.051 454.65 692.77 454.592 691.812 c
+454.554 691.165 453.173 691.517 452.83 690.588 c
+452.185 688.84 454.016 688.321 454.772 686.983 c
+454.97 686.634 454.706 686.33 454.391 686.232 c
+453.98 686.104 453.196 686.293 453.334 685.84 c
+454.306 682.647 451.55 681.969 449.201 681.399 C
+f
+439.6 661.799 m
+439.593 663.537 437.992 665.293 439.201 666.999 C
+439.336 666.865 439.467 666.644 439.601 666.644 c
+439.736 666.644 439.867 666.865 440.001 666.999 C
+441.496 664.783 445.148 663.855 445.006 661.009 c
+444.984 660.562 443.897 659.644 444.801 658.999 C
+442.988 657.651 442.933 655.281 442.001 653.399 C
+440.763 653.685 439.551 654.048 438.401 654.599 C
+438.753 656.085 438.636 657.769 439.456 659.089 c
+439.89 659.787 439.603 660.866 439.6 661.799 c
+f
+0.8 g
+273.4 670.799 m
+256.542 660.663 270.6 675.999 v
+279.4 685.599 289.4 691.199 y
+299.8 695.6 303.4 696.8 v
+307 698 322.2 703.2 325.4 703.6 c
+328.6 704 338.2 708 345 704 c
+351.8 700 359.8 695.6 y
+343.4 704 339.8 701.6 v
+336.2 699.2 329 699.6 323 696.4 C
+308.2 691.999 305 689.999 v
+301.8 687.999 291.4 676.399 289.8 677.199 c
+288.2 677.999 290.2 678.399 291.4 681.199 c
+292.6 683.999 290.6 685.599 282.6 679.199 c
+274.6 672.799 273.4 670.799 Y
+f
+0 g
+280.805 676.766 m
+282.215 689.806 290.693 688.141 V
+298.919 692.311 301.641 694.279 V
+309.78 695.981 311.09 696.598 v
+329.569 705.298 344.288 700.779 344.835 701.899 c
+345.381 703.018 365.006 695.901 368.615 691.815 c
+369.006 691.372 358.384 697.412 348.686 699.303 c
+340.413 700.917 318.811 699.056 307.905 693.52 c
+304.932 692.011 295.987 686.227 293.456 686.338 c
+290.925 686.45 280.805 676.766 Y
+f
+0.8 g
+277 651.199 m
+261.8 653.599 278.6 655.199 V
+296.6 657.199 300.6 662.399 V
+314.2 671.599 317 671.999 v
+319.8 672.399 349.8 679.599 350.2 681.999 c
+350.6 684.399 356.2 684.399 357.8 683.599 c
+359.4 682.799 358.6 681.599 355.8 680.799 c
+353 679.999 321.8 663.599 315.4 662.399 c
+309 661.199 297.4 653.599 292.6 652.399 c
+287.8 651.199 277 651.199 Y
+f
+0 g
+296.52 658.597 m
+287.938 659.426 296.539 660.245 V
+305.355 663.669 307.403 666.332 V
+314.367 671.043 315.8 671.247 v
+317.234 671.452 331.194 675.139 331.399 676.367 c
+331.604 677.596 365.67 690.177 370.09 686.987 c
+373.001 684.886 363.1 686.563 353.466 682.153 c
+352.111 681.533 318.258 666.946 314.981 666.332 c
+311.704 665.717 305.765 661.826 303.307 661.212 c
+300.85 660.597 296.52 658.597 Y
+f
+288.6 656.399 m
+293.8 656.799 292.6 655.199 v
+291.4 653.599 289 654.399 y
+288.6 656.399 l
+f
+281.4 654.799 m
+286.6 655.199 285.4 653.599 v
+284.2 651.999 281.8 652.799 y
+281.4 654.799 l
+f
+271 653.199 m
+276.2 653.599 275 651.999 v
+273.8 650.399 271.4 651.199 y
+271 653.199 l
+f
+263.4 652.399 m
+268.6 652.799 267.4 651.199 v
+266.2 649.599 263.8 650.399 y
+263.4 652.399 l
+f
+301.8 691.999 m
+306.2 691.999 305 690.399 v
+303.8 688.799 300.6 689.199 y
+301.8 691.999 l
+f
+291.8 686.399 m
+298.306 688.54 295.8 685.199 v
+294.6 683.599 292.2 684.399 y
+291.8 686.399 l
+f
+280.6 681.599 m
+285.8 681.999 284.6 680.399 v
+283.4 678.799 281 679.599 y
+280.6 681.599 l
+f
+273 675.599 m
+278.2 675.999 277 674.399 v
+275.8 672.799 273.4 673.599 y
+273 675.599 l
+f
+266.2 670.799 m
+271.4 671.199 270.2 669.599 v
+269 667.999 266.6 668.799 y
+266.2 670.799 l
+f
+305.282 664.402 m
+312.203 664.934 310.606 662.805 v
+309.009 660.675 305.814 661.74 y
+305.282 664.402 l
+f
+315.682 669.202 m
+322.603 669.734 321.006 667.605 v
+319.409 665.475 316.214 666.54 y
+315.682 669.202 l
+f
+326.482 673.602 m
+333.403 674.134 331.806 672.005 v
+330.209 669.875 327.014 670.94 y
+326.482 673.602 l
+f
+336.882 678.402 m
+343.803 678.934 342.206 676.805 v
+340.609 674.675 337.414 675.74 y
+336.882 678.402 l
+f
+309.282 696.402 m
+316.203 696.934 314.606 694.805 v
+313.009 692.675 309.014 692.94 y
+309.282 696.402 l
+f
+319.282 699.602 m
+326.203 700.134 324.606 698.005 v
+323.009 695.875 318.614 696.14 y
+319.282 699.602 l
+f
+296.6 659.599 m
+301.8 659.999 300.6 658.399 v
+299.4 656.799 297 657.599 y
+296.6 659.599 l
+f
+0.1 0.55 0.85 0.3 k
+223.4 758.8 m
+219 750 218.6 746.8 V
+219.4 755.6 220.6 757.6 v
+221.8 759.6 223.4 758.8 y
+f
+205 744.8 m
+201.8 730.4 202.2 727.6 V
+201 739.2 201.4 740.4 v
+201.8 741.6 205 744.8 y
+f
+0.8 g
+225.8 819.4 m
+225.6 816.2 l
+223.4 816 l
+237.6 803.4 238.2 795.8 V
+239 804 225.8 819.4 V
+f
+0 g
+229.784 818.135 m
+229.353 818.551 229.572 819.296 229.164 819.556 c
+228.355 820.072 230.462 820.129 230.234 820.845 c
+229.851 822.051 230.038 822.072 229.916 823.348 c
+229.859 823.946 230.447 825.486 230.832 825.926 c
+232.278 827.578 230.954 830.51 232.594 832.061 c
+232.898 832.35 233.274 832.902 233.559 833.32 c
+234.218 834.283 235.402 834.771 236.352 835.599 c
+236.67 835.875 236.469 836.702 237.038 836.61 c
+237.752 836.495 238.993 836.625 238.948 835.784 c
+238.835 833.664 237.506 831.944 236.226 830.276 C
+236.677 829.572 236.219 828.937 235.935 828.38 c
+234.6 825.76 234.789 822.919 234.615 820.079 c
+234.61 819.994 234.303 819.916 234.311 819.863 c
+234.664 817.528 235.248 815.329 236.127 813.1 c
+236.493 812.17 236.964 811.275 237.114 810.348 c
+237.225 809.662 237.328 808.829 236.92 808.124 C
+238.955 805.234 237.646 802.583 238.815 799.052 c
+239.022 798.427 240.714 796.513 240.251 796.674 c
+237.738 797.545 237.626 797.943 237.449 798.696 c
+237.303 799.319 236.973 800.696 236.736 801.298 c
+236.672 801.462 236.501 803.346 236.423 803.468 c
+234.91 805.85 236.268 805.674 234.898 808.032 C
+233.47 808.712 232.504 809.816 231.381 810.978 c
+231.183 811.182 232.326 811.906 232.145 812.119 c
+231.053 813.408 229.9 814.175 230.236 815.668 c
+230.391 816.358 230.528 817.415 229.784 818.135 c
+f
+226.2 816.4 m
+226.6 809.6 229 808 v
+231.4 806.4 230.2 807.2 227 808.4 c
+223.8 809.6 225 810.4 y
+222.2 810 224.6 808 v
+227 806 230.6 803.6 229 803.6 c
+227.4 803.6 219.8 807.6 219.8 810.4 c
+219.8 813.2 218.8 817.3 y
+219.9 818.1 224.7 818 V
+226.1 817.3 226.2 816.4 V
+f
+1 g
+1 J 0.1 w
+225.4 797.8 m
+216.88 800.591 198.4 797.2 V
+207.431 799.278 226.2 797 v
+236.5 795.75 225.4 797.8 Y
+b
+227.498 797.871 m
+219.252 801.389 200.547 799.608 V
+209.725 800.897 228.226 797.005 v
+238.38 794.869 227.498 797.871 Y
+b
+229.286 797.778 m
+221.324 801.899 202.539 801.514 V
+211.787 802.118 229.948 796.86 v
+239.914 793.975 229.286 797.778 Y
+b
+230.556 797.555 m
+223.732 801.862 206.858 802.96 V
+215.197 802.79 231.078 796.681 v
+239.794 793.328 230.556 797.555 Y
+b
+345.84 787.039 m
+344.91 786.395 345.124 787.576 v
+345.339 788.757 373.547 801.927 377.161 801.677 C
+346.913 788.471 345.84 787.039 V
+b
+342.446 786.4 m
+341.57 785.685 341.691 786.879 v
+341.812 788.073 368.899 803.418 372.521 803.452 C
+343.404 787.911 342.446 786.4 V
+b
+339.16 785.025 m
+338.332 784.253 338.374 785.453 v
+338.416 786.652 358.233 802.149 368.045 804.023 C
+350.015 795.896 339.16 785.025 V
+b
+336.284 783.162 m
+335.539 782.468 335.577 783.547 v
+335.615 784.627 353.449 798.574 362.28 800.26 C
+346.054 792.946 336.284 783.162 V
+b
+0.8 g
+0 J 1 w
+304.6 635.199 m
+289.4 637.599 306.2 639.199 V
+324.2 641.199 328.2 646.399 V
+341.8 655.599 344.6 655.999 v
+347.4 656.399 363.8 659.999 364.2 662.399 c
+364.6 664.799 370.6 667.199 372.2 666.399 c
+373.8 665.599 373.8 656.399 371 655.599 c
+368.2 654.799 349.4 647.599 343 646.399 c
+336.6 645.199 325 637.599 320.2 636.399 c
+315.4 635.199 304.6 635.199 Y
+f
+0 g
+377.6 672.599 m
+374.6 670.999 373.4 668.399 V
+367 657.799 352.8 654.599 V
+329.8 645.599 322 643.599 V
+308.6 638.599 301.2 639.399 V
+294.2 639.199 300.4 637.599 V
+320.6 639.599 324 641.399 V
+339.6 646.599 342.6 649.199 v
+345.6 651.799 363.8 656.799 366 658.799 c
+368.2 660.799 378 669.199 377.6 672.599 C
+f
+318.882 641.089 m
+324.111 641.315 322.958 639.766 v
+321.805 638.216 319.357 639.09 y
+318.882 641.089 l
+f
+311.68 639.737 m
+316.908 639.963 315.756 638.414 v
+314.603 636.864 312.155 637.737 y
+311.68 639.737 l
+f
+301.251 638.489 m
+306.48 638.716 305.327 637.166 v
+304.174 635.617 301.726 636.49 y
+301.251 638.489 l
+f
+293.617 637.945 m
+298.846 638.171 297.693 636.622 v
+296.54 635.072 294.092 635.946 y
+293.617 637.945 l
+f
+335.415 648.487 m
+342.375 648.788 340.84 646.726 v
+339.306 644.664 336.047 645.826 y
+335.415 648.487 l
+f
+345.73 652.912 m
+351.689 656.213 351.155 651.151 v
+350.885 648.595 346.362 650.251 y
+345.73 652.912 l
+f
+354.862 655.726 m
+362.021 659.427 360.287 653.965 v
+359.509 651.515 355.493 653.065 y
+354.862 655.726 l
+f
+364.376 660.551 m
+368.735 665.452 369.801 658.79 v
+370.207 656.252 365.008 657.89 y
+364.376 660.551 l
+f
+326.834 644.003 m
+332.062 644.23 330.91 642.68 v
+329.757 641.131 327.308 642.004 y
+326.834 644.003 l
+f
+1 g
+1 J 0.1 w
+362.434 765.397 m
+361.708 764.732 361.707 765.803 v
+361.707 766.873 379.191 780.137 388.034 781.521 C
+371.935 774.792 362.434 765.397 V
+b
+0 g
+0 J 1 w
+365.4 701.6 m
+387.401 679.199 396.601 675.599 V
+405.801 664.399 401.801 638.399 V
+398.601 630.799 395.401 651.599 V
+398.601 676.799 387.401 660.799 V
+379 670.699 385.4 670.399 V
+388.601 668.399 389.001 669.999 v
+389.401 671.599 381.4 685.199 364.2 699.6 c
+347 714 365.4 701.6 Y
+f
+1 g
+1 J 0.1 w
+307 662.799 m
+306.8 664.599 308.6 663.799 v
+310.4 662.999 404.601 656.799 436.201 632.799 C
+391.001 655.999 307 662.799 V
+b
+317.4 667.199 m
+317.2 668.999 319 668.199 v
+320.8 667.399 457.401 668.399 481.001 635.999 C
+459.001 661.199 317.4 667.199 V
+b
+329 671.199 m
+328.8 672.999 330.6 672.199 v
+332.4 671.399 505.801 684.399 529.401 651.999 C
+519.801 677.599 329 671.199 V
+b
+339 675.999 m
+338.8 677.799 340.6 676.999 v
+342.4 676.199 464.601 714.8 488.201 682.399 C
+474.801 707 339 675.999 V
+b
+281 653.199 m
+280.8 654.999 282.6 654.199 v
+284.4 653.399 302.2 651.199 304.2 612.399 C
+297 654.399 281 653.199 V
+b
+272.2 651.599 m
+272 653.399 273.8 652.599 v
+275.6 651.799 289.8 656.399 287 617.599 C
+288.2 652.799 272.2 651.599 V
+b
+264.2 651.199 m
+264 652.999 265.8 652.199 v
+267.6 651.399 283 650.799 270.6 628.399 C
+280.2 652.399 264.2 651.199 V
+b
+311.526 695.535 m
+311.082 693.536 312.631 694.753 v
+328.699 707.378 361.141 766.28 416.826 771.914 C
+378.518 784.024 311.526 695.535 V
+b
+322.726 697.335 m
+321.363 698.528 323.231 699.153 v
+325.099 699.778 437.541 772.28 476.826 764.314 C
+449.719 771.824 322.726 697.335 V
+b
+301.885 691.233 m
+301.376 689.634 303.087 690.61 v
+312.062 695.73 315.677 752.941 359.254 754.196 C
+326.843 768.91 301.885 691.233 V
+b
+281.962 680.207 m
+280.885 678.921 282.838 679.175 v
+293.084 680.507 314.489 721.778 358.928 716.699 C
+326.962 731.045 281.962 680.207 V
+b
+293.2 686.333 m
+292.389 684.864 294.258 685.489 v
+304.057 688.763 317.141 733.375 361.729 736.922 C
+327.603 744.865 293.2 686.333 V
+b
+274.922 675.088 m
+274.049 674.046 275.631 674.252 v
+283.93 675.331 301.268 708.76 337.264 704.646 C
+311.371 716.266 274.922 675.088 V
+b
+267.323 669.179 m
+266.318 668.134 267.909 668.252 v
+272.077 668.561 302.715 701.64 321.183 686.138 C
+309.168 704.861 267.323 669.179 V
+b
+336.855 701.102 m
+335.654 702.457 337.586 702.842 v
+339.518 703.226 460.221 760.939 498.184 748.073 C
+472.243 758.947 336.855 701.102 V
+b
+303.4 636.799 m
+303.2 638.599 305 637.799 v
+306.8 636.999 322.2 636.399 309.8 613.999 C
+319.4 637.999 303.4 636.799 V
+b
+313.8 638.399 m
+313.6 640.199 315.4 639.399 v
+317.2 638.599 335 636.399 337 597.599 C
+329.8 639.599 313.8 638.399 V
+b
+320.6 639.999 m
+320.4 641.799 322.2 640.999 v
+324 640.199 348.6 636.799 372.2 604.399 C
+336.6 641.199 320.6 639.999 V
+b
+328.225 642.028 m
+327.788 643.786 329.678 643.232 v
+331.568 642.678 352.002 644.577 390.099 610.401 C
+343.924 645.344 328.225 642.028 V
+b
+338.625 646.428 m
+338.188 648.186 340.078 647.632 v
+341.968 647.078 376.802 642.577 428.499 607.601 C
+354.324 649.744 338.625 646.428 V
+b
+298.2 657.999 m
+298 659.799 299.8 658.999 v
+301.6 658.199 355 655.599 385.4 628.799 C
+350.499 653.574 298.2 657.999 V
+b
+288.2 653.999 m
+288 655.799 289.8 654.999 v
+291.6 654.199 316.2 650.799 339.8 618.399 C
+304.2 655.199 288.2 653.999 V
+b
+349.503 651.038 m
+348.938 652.759 350.864 652.345 v
+352.79 651.932 387.86 649.996 441.981 618.902 C
+364.317 653.296 349.503 651.038 V
+b
+357.903 653.438 m
+357.338 655.159 359.264 654.745 v
+361.19 654.332 396.26 652.396 450.381 621.302 C
+373.317 656.096 357.903 653.438 V
+b
+367.503 658.438 m
+366.938 660.159 368.864 659.745 v
+370.79 659.332 413.86 654.996 503.582 620.702 C
+382.917 661.096 367.503 658.438 V
+b
+0 g
+0 J 1 w
+256.2 651.599 m
+261.4 651.999 260.2 650.399 v
+259 648.799 256.6 649.599 y
+256.2 651.599 l
+f
+287 637.599 m
+292.2 637.999 291 636.399 v
+289.8 634.799 287.4 635.599 y
+287 637.599 l
+f
+278.2 637.999 m
+283.4 638.399 282.2 636.799 v
+281 635.199 278.6 635.999 y
+278.2 637.999 l
+f
+182.831 649.818 m
+187.876 648.495 186.218 647.376 v
+184.561 646.256 182.554 647.798 y
+182.831 649.818 l
+f
+184.831 659.418 m
+189.876 658.095 188.218 656.976 v
+186.561 655.856 184.554 657.398 y
+184.831 659.418 l
+f
+177.631 663.818 m
+182.676 662.495 181.018 661.376 v
+179.361 660.256 177.354 661.798 y
+177.631 663.818 l
+f
+0.8 g
+1 J 0.1 w
+257.4 588.799 m
+255.8 588.799 251.8 586.799 V
+249.8 586.799 238.6 583.199 233 573.199 C
+245.4 582.799 257.4 588.799 V
+f
+345.116 496.153 m
+345.257 495.895 345.312 495.475 345.604 495.458 c
+346.262 495.418 347.495 495.117 347.37 495.753 c
+346.522 500.059 345.648 504.996 341.515 506.803 c
+340.876 507.082 339.434 506.669 339.36 505.785 c
+339.233 504.261 339.116 502.912 339.425 501.446 c
+339.725 500.025 341.883 500.015 342.8 501.399 C
+343.736 499.727 344.168 497.884 345.116 496.153 c
+f
+334.038 491.419 m
+334.786 490.006 334.659 488.147 336.074 487.584 c
+336.814 487.29 338.664 488.265 338.246 489.339 c
+337.444 491.4 337.056 493.639 335.667 495.45 c
+335.467 495.712 335.707 496.245 335.547 496.573 c
+334.953 497.793 333.808 498.528 332.4 498.199 C
+331.285 495.996 332.433 493.867 333.955 492.158 c
+334.091 492.006 333.925 491.63 334.038 491.419 c
+f
+294.436 496.609 m
+294.328 496.986 294.29 497.449 294.455 497.77 c
+294.986 498.803 295.779 499.925 295.442 500.947 c
+295.094 502.003 293.978 501.821 293.328 501.252 c
+292.193 500.258 292.144 498.432 291.453 497.073 c
+291.257 496.687 291.308 496.114 290.867 495.723 c
+290.393 495.302 289.953 493.778 290.049 493.207 c
+290.102 492.894 289.919 482.986 290.141 483.249 c
+290.76 483.982 293.81 493.716 293.879 494.608 c
+293.936 495.339 294.668 495.804 294.436 496.609 c
+f
+268.798 503.401 m
+271.432 505.9 274.222 508.861 273.78 512.573 c
+273.664 513.549 271.889 513.022 271.702 512.176 c
+270.9 508.551 268.861 505.89 266.293 503.498 c
+264.097 501.451 262.235 495.107 262 494.599 C
+265.697 499.855 267.954 502.601 268.798 503.401 c
+f
+255.224 509.365 m
+255.747 509.735 255.445 510.226 255.662 510.558 c
+256.615 512.016 257.916 513.262 257.934 515 c
+257.937 515.277 257.559 515.586 257.224 515.362 c
+256.947 515.178 256.605 515.048 256.497 514.918 c
+254.467 512.469 253.067 509.798 251.624 506.986 c
+251.441 506.629 250.297 502.138 250.61 502.027 c
+250.849 501.942 252.569 506.123 252.779 506.237 c
+254.042 506.923 254.054 508.538 255.224 509.365 c
+f
+271.957 489.821 m
+272.401 490.69 273.977 491.892 273.864 492.781 c
+273.746 493.709 274.214 495.152 273.302 494.464 c
+272.045 493.516 268.596 492.167 268.326 486.359 c
+268.3 485.788 271.274 488.481 271.957 489.821 c
+f
+286.4 506.999 m
+286.8 507.667 287.508 507.194 287.967 507.457 c
+288.615 507.829 289.226 508.387 289.518 509.036 c
+290.488 511.185 292.257 513.005 292.4 515.399 C
+290.909 516.804 290.23 514.764 289.6 513.799 C
+288.277 515.446 287.278 513.572 285.978 513.053 c
+285.908 513.025 285.695 513.372 285.62 513.345 c
+284.443 512.905 283.763 511.824 282.765 511.043 c
+282.594 510.909 282.189 511.089 282.042 510.953 c
+281.39 510.35 280.417 510.025 280.137 509.343 c
+279.027 506.636 275.887 504.541 274 496.999 C
+274.381 496.09 278.512 503.641 278.999 504.339 c
+279.835 505.535 279.953 502.678 281.229 503.344 c
+281.28 503.371 281.466 503.133 281.6 502.999 C
+281.794 503.279 282.012 503.508 282.4 503.399 C
+282.4 503.799 282.266 504.355 282.467 504.514 c
+283.704 505.491 283.62 506.559 284.4 507.799 C
+284.858 507.01 285.919 507.729 286.4 506.999 C
+f
+346.2 452.599 m
+353.6 472.999 349.2 484.199 V
+360.6 462.599 356 451.399 V
+355.6 461.799 351.6 466.799 V
+347.6 453.999 346.2 452.599 V
+f
+331.4 455.199 m
+336.8 463.999 328.8 482.399 V
+328 461.999 321.2 450.999 V
+335.4 471.199 331.4 455.199 V
+f
+321.4 457.199 m
+321.2 477.199 321.6 480.199 V
+317.8 463.599 307.6 453.999 V
+322 465.999 321.4 457.199 V
+f
+311.8 489.199 m
+317.8 475.599 307.8 457.199 V
+314.2 469.399 309.4 476.399 V
+312 479.799 311.8 489.199 V
+f
+292.6 457.599 m
+291.6 473.199 293.4 475.399 V
+293.6 481.799 293.2 482.799 V
+297.2 488.999 297.4 481.599 V
+298.8 473.799 301.6 469.199 V
+305.2 463.799 305 457.399 V
+295 487.599 292.6 457.599 V
+f
+289 485.199 m
+282.4 474.399 280.6 455.399 V
+279.2 461.599 283 475.999 V
+287.2 491.399 289 485.199 V
+f
+267.2 465.399 m
+272.2 470.799 273.6 475.799 V
+277.2 491.599 270.8 482.999 V
+271 474.999 262.8 467.599 V
+267.6 469.999 267.2 465.399 V
+f
+261.4 470.399 m
+264.8 487.799 265.6 488.599 V
+267.4 491.999 264.6 488.799 V
+255.8 469.599 251.8 462.999 V
+259.8 472.199 261.4 470.399 V
+f
+255.6 486.999 m
+267.2 509.399 245.4 483.599 V
+256.4 493.399 255.6 486.999 V
+f
+240.2 501.599 m
+245 520.399 247.6 520.199 V
+255.8 529.199 249.2 518.599 V
+243.2 508.999 243.8 499.199 V
+243.2 508.799 240.2 501.599 V
+f
+570.5 513 m
+558.5 523 556 526.5 V
+569.5 508 569.5 501 V
+572 508.5 570.5 513 V
+f
+576 535 m
+555 550 551.5 557.5 V
+578 528 578 523.5 V
+578.5 532.5 576 535 V
+f
+593 689 m
+581 697 579.5 695 V
+590 688.5 592.5 680 V
+591 689 593 689 V
+f
+601.5 608.5 m
+584 620.5 l
+603 603.5 603.5 599.5 V
+601.5 608.5 L
+f
+0 g
+1 w
+210.75 631 m
+232.75 626.25 l
+S
+261 469 m
+260.5 472.5 251.5 462 v
+S
+266.5 464 m
+268.5 470.5 262 466 v
+S
+320.5 455.5 m
+322 466.5 310.5 453.5 v
+S
+
+showpage
+
+%%Trailer
diff --git a/samples/samples.go b/samples/samples.go
new file mode 100644
index 0000000..ff30559
--- /dev/null
+++ b/samples/samples.go
@@ -0,0 +1,24 @@
+// Package samples provides examples which can be used with different
+// backends. They are also used for testing and coverage of the
+// draw2d package.
+package samples
+
+import "fmt"
+
+// Resource returns a resource filename for testing.
+func Resource(folder, filename, ext string) string {
+ var root string
+ if ext == "pdf" {
+ root = "../"
+ }
+ return fmt.Sprintf("%sresource/%s/%s", root, folder, filename)
+}
+
+// Output returns the output filename for testing.
+func Output(name, ext string) string {
+ var root string
+ if ext == "pdf" {
+ root = "../"
+ }
+ return fmt.Sprintf("%soutput/samples/%s.%s", root, name, ext)
+}
diff --git a/samples_test.go b/samples_test.go
index 2d49caf..fe22f06 100644
--- a/samples_test.go
+++ b/samples_test.go
@@ -6,27 +6,40 @@ import (
"testing"
"github.com/llgcode/draw2d"
- "github.com/llgcode/draw2d.samples"
- "github.com/llgcode/draw2d.samples/android"
- "github.com/llgcode/draw2d.samples/frameimage"
- "github.com/llgcode/draw2d.samples/gopher"
- "github.com/llgcode/draw2d.samples/helloworld"
- "github.com/llgcode/draw2d.samples/line"
- "github.com/llgcode/draw2d.samples/linecapjoin"
- "github.com/llgcode/draw2d.samples/postscript"
+ "github.com/llgcode/draw2d/samples/android"
+ "github.com/llgcode/draw2d/samples/frameimage"
+ "github.com/llgcode/draw2d/samples/geometry"
+ "github.com/llgcode/draw2d/samples/gopher"
+ "github.com/llgcode/draw2d/samples/gopher2"
+ "github.com/llgcode/draw2d/samples/helloworld"
+ "github.com/llgcode/draw2d/samples/line"
+ "github.com/llgcode/draw2d/samples/linecapjoin"
+ "github.com/llgcode/draw2d/samples/postscript"
)
func TestSampleAndroid(t *testing.T) {
test(t, android.Main)
}
+func TestSampleGeometry(t *testing.T) {
+ // Set the global folder for searching fonts
+ // The pdf backend needs for every ttf file its corresponding
+ // json/.z file which is generated by gofpdf/makefont.
+ draw2d.SetFontFolder("resource/font")
+ test(t, geometry.Main)
+}
+
func TestSampleGopher(t *testing.T) {
test(t, gopher.Main)
}
+func TestSampleGopher2(t *testing.T) {
+ test(t, gopher2.Main)
+}
+
func TestSampleHelloWorld(t *testing.T) {
// Set the global folder for searching fonts
- draw2d.SetFontFolder(samples.Dir("helloworld", ""))
+ draw2d.SetFontFolder("resource/font")
test(t, helloworld.Main)
}
@@ -38,7 +51,7 @@ func TestSampleLine(t *testing.T) {
test(t, line.Main)
}
-func TestSampleLineCap(t *testing.T) {
+func TestSampleLineCapJoin(t *testing.T) {
test(t, linecapjoin.Main)
}
diff --git a/test b/test
new file mode 100644
index 0000000..2a387e6
--- /dev/null
+++ b/test
@@ -0,0 +1,8 @@
+echo golint
+golint ./... | grep "draw2dpdf\|samples\|^advanced_path\|^arc\|draw2d[.]\|fileutil\|^gc\|math\|^path[.]\|rgba_interpolation\|test\|vertex2d"
+echo
+echo go vet
+go vet ./...
+echo
+echo go test
+go test -cover ./... | grep -v "no test"
\ No newline at end of file
diff --git a/test_test.go b/test_test.go
index ca58f79..33b8937 100644
--- a/test_test.go
+++ b/test_test.go
@@ -7,6 +7,7 @@ import (
"testing"
"github.com/llgcode/draw2d"
+ "github.com/llgcode/draw2d/draw2dimg"
)
type sample func(gc draw2d.GraphicContext, ext string) (string, error)
@@ -14,16 +15,16 @@ type sample func(gc draw2d.GraphicContext, ext string) (string, error)
func test(t *testing.T, draw sample) {
// Initialize the graphic context on an RGBA image
dest := image.NewRGBA(image.Rect(0, 0, 297, 210.0))
- gc := draw2d.NewGraphicContext(dest)
+ gc := draw2dimg.NewGraphicContext(dest)
// Draw Android logo
- fn, err := draw(gc, "png")
+ output, err := draw(gc, "png")
if err != nil {
- t.Errorf("Drawing %q failed: %v", fn, err)
+ t.Errorf("Drawing %q failed: %v", output, err)
return
}
// Save to png
- err = draw2d.SaveToPngFile(fn, dest)
+ err = draw2dimg.SaveToPngFile(output, dest)
if err != nil {
- t.Errorf("Saving %q failed: %v", fn, err)
+ t.Errorf("Saving %q failed: %v", output, err)
}
}